Working With Dimension & Position CSS PropertiesS2C Home « Working With Dimension & Position CSS Properties
In this lesson we look at how jQuery allows us to get and set box model dimensions and position the screen.
The jQuery dimension property methods allow us to retrieve and set the CSS box-model properties.
The jQuery position property methods allow us to retrieve and set the CSS position of an elements properties.
Dimension/Position Methods | Description |
---|---|
Dimensions | |
.height() | Retrieve the currently computed CSS content height of the first element within the matched set or set the CSS content height of every matched element. |
.innerHeight() | Retrieve the currently computed CSS height of the first element within the matched set including padding, but not border or margin. |
.outerHeight() | Retrieve the currently computed CSS height for the first element in the set of matched elements, including padding, border, and optionally margin. |
.width() | Retrieve the currently computed CSS content width of the first element within the matched set or set the CSS content width of every matched element. |
.innerWidth() | Retrieve the currently computed CSS width of the first element within the matched set including padding, but not border or margin. |
.outerWidth() | Retrieve the currently computed CSS width for the first element in the set of matched elements, including padding, border, and optionally margin. |
Positional | |
.offset() | Retrieve the current coordinates from the first element within the matched set relative to the document, or set the current coordinates within the matched set relative to the document. |
.position() | Retrieve the current coordinates from the first element within the matched set, relative to the offset parent. |
.scrollLeft() | Retrieve current horizontal position of the scroll bar for the first element within the matched set or set current horizontal position of the scroll bar for each element within the matched set. |
.scrollTop() | Retrieve current vertical position of the scroll bar for the first element within the matched set or set current vertical position of the scroll bar for each element within the matched set. |
Dimension Property Methods
These methods allow us to retrieve and set the CSS box-model properties.
The .height()
Method Top
Used to retrieve the currently computed CSS content height of the first element within the matched set or set the CSS content height of every matched element.
We will be using the .height()
signature for our example which retrieves the currently computed CSS content height from the first element within the matched set.
The second signature .height( value )
sets the height of the box in accordance with the CSS box-sizing property
and if this is set
to border-box
it will cause this function to change the outer height of the box instead of the content height.
The third signature .height( function(index, value) )
will execute a function that returns the CSS height to set.
Examples of all three signatures are available in the reference section.
In the example below when we press the button we get the heights of the table and the table row below.
Table Row 1, Table Data 1 | Table Row 1, Table Data 2 |
$(function(){
$('#btn5').on('click', function() {
alert('The table has a height of ' + $('#table1').height()
+ ' and the table row has a height of ' + $('#table1 tr').height());
});
});
The .innerHeight()
Method Top
Used to retrieve the currently computed CSS height of the first element within the matched set including padding, but not border or margin.
In the example below when we press the button we get the height and inner height of the image below. As you can see from the CSS the inner height includes the padding.
#main #img1 {
background-color: green;
margin: 20px;
padding: 20px;
border: 10px solid blue;
width: 200px;
height: 150px;
}
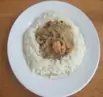
$(function(){
$('#btn6').on('click', function() {
alert('The image has a height of ' + $('#img1').height()
+ ' and an inner height of ' + $('#img1').innerHeight());
});
});
The .outerHeight( [includeMargin] )
Method Top
Used to retrieve the currently computed CSS height for the first element in the set of matched elements, including padding, border, and optionally margin.
In the example below when we press the button we get the height, inner height and outer height of the image below. As you can see from the CSS the outer height includes the padding and border.
#main #img2 {
background-color: green;
margin: 20px;
padding: 20px;
border: 10px solid blue;
width: 200px;
height: 150px;
}
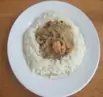
$(function(){
$('#btn7').on('click', function() {
alert('The image has a height of ' + $('#img2').height()
+ ' , an inner height of ' + $('#img2').innerHeight()
+ ' , an outer height of ' + $('#img2').outerHeight()
+ ' and a true outer height of ' + $('#img2').outerHeight(true));
});
});
The .width()
Method Top
Used to retrieve the currently computed CSS content width of the first element within the matched set or set the CSS content width of every matched element.
The first signature .width()
retrieves the currently computed CSS content width from the first element within the matched set.
The second signature .width( value )
sets the width of the box in accordance with the CSS box-sizing property
and if this is set
to border-box
it will cause this function to change the outerWidth of the box instead of the content width.
The third signature .width( function(index, value) )
will execute a function that returns the CSS width to set.
Similar to the .height()
method but for width. Examples of all three signatures are available in the reference section.
The .innerWidth()
Method Top
Used to retrieve the currently computed CSS width of the first element within the matched set including padding, but not border or margin.
Similar to the .innerHeight()
method but for width. An Example is available in the reference section.
The .outerWidth( [includeMargin] )
Method Top
Used to retrieve the currently computed CSS width for the first element in the set of matched elements, including padding, border, and optionally margin.
Similar to the .outerHeight()
method but for width. An Example is available in the reference section.
Position Property Methods
These methods allow us to retrieve and set the CSS position of an elements properties.
The .offset()
Method Top
Used to retrieve the current coordinates from the first element within the matched set relative to the document, or set the current coordinates within the matched set relative to the document.
We will be using the .offset( function(index, coordinates) )
signature for our example which will execute a function that returns the coordinates to set.
The second signature .offset()
retrieves the current coordinates from the first element within the matched set, relative to the document.
The third signature .offset( coordinates )
sets the current coordinates within the matched set, relative to the document.
Examples of all three signatures are available in the reference section.
In the example below when we press the button the first time we change the offset of the image with an index of 1.
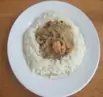
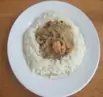
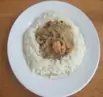
$(function(){
$('#btn8').one('click', function() {
$('#main .curry').offset( function (index, coordinates) {
if (index == 1) {
coordinates.top +=20;
coordinates.left +=20;
}
return $(this).offset(coordinates);
});
});
});
The .position()
Method Top
Used to retrieve the current coordinates from the first element within the matched set, relative to the offset parent.
Similar to the .offset()
signature of the .offset()
method but the position is relative to the offset parent. An Example is available in the reference section.
The .scrollLeft()
Method Top
Used to retrieve the current horizontal position of the scroll bar for the first element within the matched set or set the current horizontal position of the scroll bar for each element within the matched set.
We will be using the .scrollLeft()
signature for our example which will retrieve the current horizontal position of the scroll bar for the first element within the matched set.
The second signature .scrollLeft( value )
sets the current horizontal position of the scroll bar of each element within the matched set.
In the example below when we press the button we get the current horizontal position of the scroll bar within the 'iframe' below. Scroll the horizontal scroll bar and press the button again to see the position change.
$(function(){
$('#btn9').on('click', function() {
alert($('#ourIframe').contents()
.scrollLeft() + ' is the current horizontal scroll position.');
});
});
The .scrollTop()
Method Top
Used to retrieve the current vertical position of the scroll bar for the first element within the matched set or set the current vertical position of the scroll bar for each element within the matched set.
The first signature .scrollTop()
retrieves the current vertical position of the scroll bar for the first element within the matched set.
The second signature .scrollTop( value )
sets the current vertical position of the scroll bar of each element within the matched set.
Similar to the .scrollLeft()
method but for vertical positioning instead of horizontal. Examples are available in the reference section.
Lesson 11 Complete
In this lesson we learnt how jQuery allows us to get and set box model dimensions and position the screen.
Related Tutorials
jQuery 3.5 Basics - Lesson 8: Working With CSS Classes
jQuery 3.5 Basics - Lesson 9: Working With CSS Attributes
jQuery 3.5 Basics - Lesson 10: Working With General CSS Properties
What's Next?
This concludes the basic section of our jQuery tutorials. We start the intermediate section with a look at the lessons available for this section.
jQuery 3.5 Reference Methods
Attributes and Properties - .css()
method
Attributes & Properties - .height()
method
Attributes and Properties - .innerHeight()
method
Attributes and Properties - .innerWidth()
method
Attributes and Properties - .offset()
method
Attributes and Properties - .outerHeight()
method
Attributes and Properties - .outerWidth()
method
Attributes and Properties - .position()
method
Attributes & Properties - .scrollLeft()
method
Attributes & Properties - .scrollTop()
method
Attributes & Properties - .width()
method
Events - .on()
method
Events - .one()
method