Fading & Sliding EffectsS2C Home « Fading & Sliding Effects
For this lesson, we take our second look at effects by exploring the fade and slide effects jQuery has to offer.
The jQuery fade effects in this suite allow us to show or hide elements by adjusting the opacity or transparency respectively as well as toggling this effect. We can also adjust the opacity of elements to make them less or more visible.
The jQuery slide effects in this suite allow us to show or hide elements with a sliding motion as well as toggling this effect.
Fading & Sliding Effects Methods | Description |
---|---|
Fading | |
.fadeIn() | Show the matched set by fading it to opaque. |
.fadeOut() | Hide the matched set by fading it to transparent. |
.fadeTo() | Adjust the opacity of the matched set. |
.fadeToggle() | Animate the opacity of the matched set to hide or show it. |
Sliding | |
.slideDown() | Uses a sliding motion to hide the matched set. |
.slideToggle() | Uses a sliding motion to hide or show the matched set. |
.slideUp() | Uses a sliding motion to show the matched set. |
Fading Effects
The .fadeIn()
Method Top
Show the matched set by fading it to opaque.
We use this methods only signature .fadeIn( [duration] [, easing] [, callback] )
in our examples below which fades in
the matched set to opaque, optionally providing a duration and/or an easing and/or a callback function.
In the example below when the first button is pressed we fade in the first image and it defaults to a fade in a time of 400 milliseconds.
When the second button is pressed we use 'swing' easing to animate the picture as we fade it in.
When the third button is pressed we use a callback to alert when the image is faded in.
When the fourth button is pressed we use a duration, easing and a callback to fade in the last 3 images to their specified values.
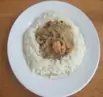
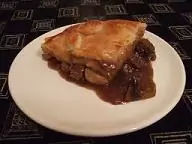
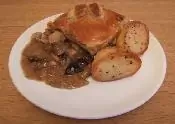
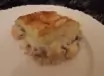
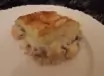
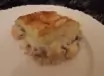
$(function(){
$('#btn1').on('click', function() {
$('#curry1').fadeIn();
});
$('#btn2').on('click', function() {
$('#pie1').fadeIn('swing');
});
$('#btn3').on('click', function() {
$('#pie2').fadeIn(function() {
alert('Animation finished.');
});
});
$('#btn4').on('click', function() {
$('.pie3').first().fadeIn('slow', 'swing', function() {
// using 'callee' so we don't have to name the function
$(this).next().fadeIn(1200, arguments.callee);
});
});
});
The .fadeOut()
Method Top
Hide the matched set by fading it to transparent.
We use this methods only signature .fadeOut( [duration] [, easing] [, callback] )
in our examples below which hides the
matched set by fading it to transparent, optionally providing a duration and/or an easing and/or a callback function.
In the example below when the first button is pressed we fade out the first image and it defaults to a fade out duration of 400 milliseconds.
In the example below when the second button is pressed we use 'swing' easing to animate the picture as we fade it out.
When the third button is pressed we use a callback to alert when the image is faded out.
When the fourth button is pressed we use a duration, easing and a callback to alert when the images are faded out.
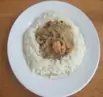
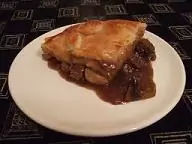
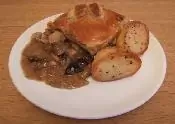
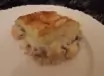
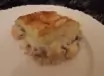
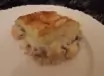
$(function(){
$('#btn5').on('click', function() {
$('#curry2').fadeOut();
});
$('#btn6').on('click', function() {
$('#pie4').fadeOut('swing');
});
$('#btn7').on('click', function() {
$('#pie5').fadeOut(function() {
alert('Animation finished.');
});
});
$('#btn8').on('click', function() {
$('.pie6').first().fadeOut('slow', 'swing', function() {
// using 'callee' so we don't have to name the function
$(this).next().fadeOut(1200, arguments.callee);
});
});
});
The .fadeTo()
Method Top
Adjust the opacity of the matched set.
We use this methods only signature .fadeTo( duration, opacity [, easing] [, callback] )
in our examples below which adjusts
the opacity of the matched set, optionally providing an easing and/or a callback function.
In the example below when the first button is pressed we fade the first first image to an opacity of 0.75 with a slow duration.
In the example below when the second button is pressed we use 'swing' easing with an opacity of 0.3 with a fast duration.
When the third button is pressed we use a duration, easing and a callback to fade the last 3 images to their specified values.
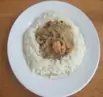
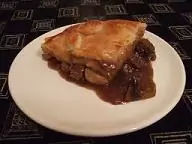
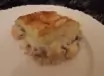
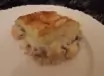
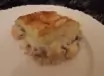
$(function(){
$('#btn13').on('click', function() {
$('#curry4').fadeTo('slow', 0.75);
});
$('#btn14').on('click', function() {
$('#pie10').fadeTo('fast', 0.3, 'swing');
});
$('#btn15').on('click', function() {
$('.pie11').first().fadeTo('fast', 0.8, 'linear', function() {
// using 'callee' so we don't have to name the function
$(this).next().fadeTo(1200, 0.4, arguments.callee);
});
});
});
The .fadeToggle()
Method Top
Animate the opacity of the matched set to hide or show it.
We use this methods only signature .fadeToggle( [duration] [, easing] [, callback] )
in our examples below which animates the opacity
of the matched set to hide or show it, optionally providing a duration and/or an easing and/or a callback function.
In the example below when the first button is pressed we fade in or out the first image and it defaults to a fade in a time of 400 milliseconds.
In the example below when the second button is pressed we use 'swing' easing to animate the picture as we fade it in or out.
When the third button is pressed we use a callback to alert when the image is faded in or out.
When the fourth button is pressed we use a duration, easing and a callback to fade in or out the last 3 images to their specified values.
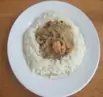
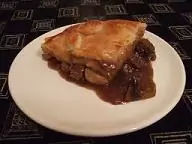
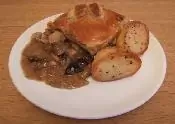
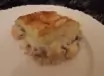
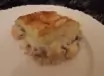
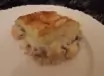
$(function(){
$('#btn9').on('click', function() {
$('#curry3').fadeToggle();
});
$('#btn10').on('click', function() {
$('#pie7').fadeToggle('swing');
});
$('#btn11').on('click', function() {
$('#pie8').fadeToggle(function() {
alert('Animation finished.');
});
});
$('#btn12').on('click', function() {
$('.pie9').first().fadeToggle('slow', 'swing', function() {
// using 'callee' so we don't have to name the function
$(this).next().fadeToggle(1200, arguments.callee);
});
});
});
Sliding Effects
The .slideDown()
Method Top
Adjust the opacity of the matched set, optionally providing an easing and/or a callback function.
We use this methods only signature .slideDown( [duration] [, easing] [, callback] )
in our examples below which uses a
sliding motion to show the matched set optionally providing a duration, and/or an easing and/or a callback function.
In the example below when the button is pressed we use a duration, easing and a callback to alert when the image is slid down.
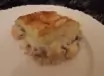
$(function(){
$('#btn18').on('click', function() {
$('#pie14').slideDown('slow', 'swing', function() {
alert('Animation complete.');
});
});
});
The .slideToggle()
Method Top
Adjust the opacity of the matched set, optionally providing an easing and/or a callback function.
We use this methods only signature .slideToggle( [duration] [, easing] [, callback] )
in our examples below which uses a
sliding motion to hide or show the matched set optionally providing a duration, and/or an easing and/or a callback function.
In the example below when the button is pressed we use 'swing' easing to animate the picture as we slide it up or down.
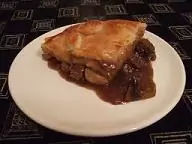
$(function(){
$('#btn22').on('click', function() {
$('#pie18').slideToggle('swing');
});
});
The .slideUp()
Method Top
Uses a sliding motion to hide the matched set optionally providing a duration, and/or an easing and/or a callback function.
We use this methods only signature .slideUp( [duration] [, easing] [, callback] )
in our examples below which uses a
sliding motion to hide the matched set optionally providing a duration, and/or an easing and/or a callback function.
In the example below when the left button is pressed we use slow duration time to animate the picture as we slide it up.
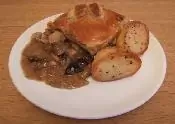
$(function(){
$('#btn25').on('click', function() {
$('#pie21').slideUp(2000);
});
});
Lesson 7 Complete
In this lesson we took a look at the jQuery fading and sliding effects methods.
Related Tutorials
jQuery 3.5 Intermediate - Lesson 6: Basic & Custom Effects
jQuery 3.5 Intermediate - Lesson 8: Controlling Effects
What's Next?
In the next lesson we investigate the jQuery methods for controlling effects.
jQuery 3.5 Reference Methods
Events - .on()
method
Traversal - .next()
method
Effects - .fadeIn()
method
Effects - .fadeOut()
method
Effects - .fadeTo()
method
Effects - .fadeToggle()
method
Effects - .slideDown()
method
Effects - .slideToggle()
method
Effects - .slideUp()
method