EL FunctionsS2C Home « EL Functions
In this JSTL lesson we look at EL Functions which are very similar in concept and implementation to the custom tags we created in the last lesson. Just Like custom tags EL functions require an entry within a Tag Library Descriptor (TLD) that maps the EL function class to the JSP page it is called from. Let's look at the steps involved in writing and using an EL function:
- Create a
public
class that containsstatic
methods where each static method represents an EL function. - Register the EL function within a TLD file.
- Reference the TLD file from within any JSP page you want to use your EL function within.
So why use EL functions if we can use custom tags? The answer is simplicity and reusability. EL functions can work for any class as long as the method used for the EL function is marked with the public static
modifier. So any existing classes that have methods with the public static
modifier, such as java.lang.Math
, are candidates to be used as EL functions.
EL Function ExampleTop
Lets create a simple EL function so we can see the mechanics involved. With EL functions there is no need to import the Servlet and JSP APIs, as you have to with custom tags, as EL functions are just standard Java classes.
package elfunc;
public class ReplaceString {
public static String replString(String s) {
return new StringBuffer(s).replace("a", "i");
}
}
Compiling Our EL FunctionTop
I put the Java code in directory c:\_JSTL\elfunctions\src\elfunc
The apache-tomcat-6.0.37
path is where I downloaded the Tomcat version I used into.
We compile ReplaceString.java
using the java compiler with the -cp
and -d
options as shown below
javac -d ..\..\classes ReplaceString.java
The following screenshot shows that we get a clean compile and also the ReplaceString
class now compiled into the classes\elfunc
directory.
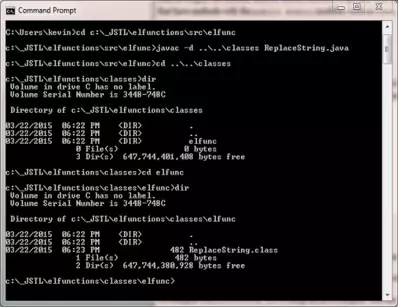
Registering EL Functions Within The TLDTop
The container and any JSP pages that want to make use of EL functions need a way to locate the EL function in question and this is achieved by registering the EL function using a Tag Library Descriptor (TLD) that points to the class and function that we are using for the EL function. The container will search in several places for TLDs as outlined below, so make sure that any you create are in one of the locations listed:
- Directly inside the
WEB-INF
folder - Directly inside a sub-directory of
WEB-INF
folder - Inside the
META-INF
directory inside aJAR
file that's inside theWEB-INF
folder - Inside a sub-directory of the
META-INF
directory, inside aJAR
file that's inside theWEB-INF
folder
A TLD is an XML file with the tld
extension, also note the namespace used for EL functions which is the same as for the custom tag libraries we used in the Creating Our Own Custom Tags lesson. In fact you
can use the same TLD file for custom tags and EL functions, there is no need to have separate TLD files, just make sure each entity within your TLD file has a unique name
The following code example shows the basic TLD file we use to map to an EL function. All TLD elements used with an EL function reside within the <function> top-level element. The
<description> sub-level element is optional and can be used to describe what the function does. The <name> sub-level element is mandatory and can be any logical name
that uniquely qualifies this function. The <function-class> sub-level element is mandatory and is the fully qualified name of the Java class containing the function implementation. The
<function-signature> sub-level element is mandatory and represents the signature of the Java method representing the function. The signature must include a return type or void
and any parameters must be listed in the same order as they appear in the Java method, although there is no need to specify parameter identifiers. Any objects used for return type or parameters must use their fully qualified class names
<?xml version="1.0" encoding="UTF-8"?>
<taglib xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee/web-jsptaglibrary_2_1.xsd" version="2.1"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/j2ee">
<description>EL Functions</description>
<tlib-version>1.0</tlib-version>
<function>
<description>String Character Replacement</description>
<name>replaceCharactersInString</name>
<function-class>elfunc.ReplaceString</function-class>
<function-signature>java.lang.String replString(java.lang.String)</function-signature>
</function>
</taglib>
Using EL FunctionsTop
When we want to use an EL function within our JSP pages we use the taglib
directive which can reference either a relative path of the context root or an absolute path that points to the location of the TLD file.
The following code example shows a JSP page called testelfunc.jsp
that uses the EL function we wrote above.
<%@ taglib uri="/WEB-INF/elfunction.tld" prefix="elf"%>
<!DOCTYPE html>
<html>
<head><title>Testing Our EL Function</title></head>
<body>
<h1>Testing Our EL Function</h1>
<p>${elf:replaceCharactersInString("The cat sat on the mat")}</p>
</body>
</html>
The screenshot below shows the file structure within Tomcat folder c:\apache-tomcat-6.0.37\webapps\elfunc
for the above entities.
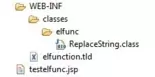
The following screenshot shows the results of running the testelfunc.jsp
JSP page within the elfunc
folder within Tomcat:
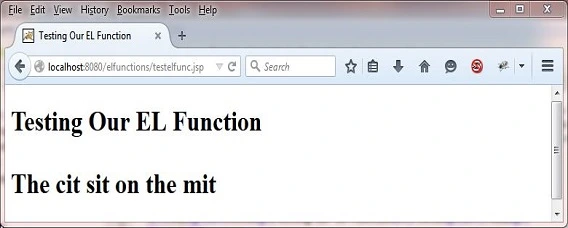
Lesson 8 Complete
In this lesson we looked at EL Functions.
What's Next?
In our final lesson on JSTL we look at Tag Files.