JSTL Core
Tag LibraryS2C Home « JSTL Core
Tag Library
In our first lesson on JSTL tag libraries we look at the Core
tag library. The Core
tag library consists of fourteen actions which we can group into four seperate areas covering general purpose, conditional,
iteration and URL related. We will look at each of these groups and the actions within each, in much more detail, as we work through this lesson.
Each Core
action can have mandatory attributes that have to be populated and each attribute may also allow a run-time expression to be used. If a run-time expression is allowed for an attribute this can be
populated using a static string or more interestingly a dynamic value which can be an EL or Java expression or a value set using the <jsp:attribute>
standard action. If no run-time expression is
allowed for an attribute then you can only populate that attribute using a static string.
General Purpose Core
ActionsTop
There are four general purpose Core
actions, these being <c:set>
which allows us to set an attribute within any scope, <c:remove>
which allows us to remove an
attribute from any scope, <c:out>
which allows us to output expressions and template text and <c:catch>
which allows us to intercept errors before they propogate up the stack.
Action / Attribute Name |
Mandatory | Run-time Expression? | Default Value | Object Type | Description |
---|---|---|---|---|---|
<c:set> | |||||
value | No | Yes | None | Object | String to be created, or reference to a scoped object, or new string property value. |
var | No | No | None | String | Name of scoped variable to be created. |
scope | No | No | Page | String | Scope that the created scoped variable exists in. |
target | No | Yes | None | Object | A JavaBeans or java.util. scoped object to assign a new string property value to. |
property | No | Yes | None | String | Name of the property to be assigned a new string property value. |
<c:remove> | |||||
var | Yes | No | None | String | Name of scoped variable to be removed. |
scope | No | No | Page | String | Scope that scoped variable to be removed exists in. |
<c:out> | |||||
value | Yes | Yes | None | Object | Expression to be evaluated. |
escapeXml | No | Yes | true | Boolean | Indicates whether the characters < > & ' and " are escaped to their HTML entity codes |
default | No | Yes | Empty string | Object | Default value. |
<c:catch> | |||||
var | No | No | None | String | Paged scoped variable representing the exception. |
<c:set>
Top
The <c:set>
tag allows us to create an object in any scope and depending on the form used, of which there are four variants, can be used to do any of the following:
- Create a scoped object that references a string property value.
- Create a scoped variable that references an existing scoped object.
- Modify the string property value of a scoped object.
The following code snippets shows the four variants of the <c:set>
tag available where the square brackets indicate optional attributes and the curly bracers indicate a choice of options within
them where the default is underlined.
// Create scoped object where value attribute specifes a string property value
<c:set value="value" var="varName" [scope="{page|request|session|application}"]>
// Create scoped object where value attribute specifes a string property value
// passed in the body content
<c:set var="varName" [scope="{page|request|session|application}"]>
specified value
</c:set>
// Modify the string property value of a scoped object
<c:set target="target" property="propertyName" value="value"">
// Modify the string property value of a scoped object passed in the body content
<c:set target="target" property="propertyName">
specified value
</c:set>
<c:remove>
Top
The <c:remove>
tag allows us to remove a scoped variable. The actual object referenced by the scoped variable is not removed so any other scoped variables that access the object can still do so.
The following code snippets shows the syntax of the <c:remove>
tag where the square brackets indicate optional attributes and the curly bracers indicate a choice of options within
them where the default is underlined.
// Remove scoped variable
<c:remove var="varName" [scope="{page|request|session|application}"]>
<c:out>
Top
The <c:out>
tag allows us to evaluate an expression and outputs the results to the current JspWriter
and consists of two variants, with or without a body.
The following code snippets shows the two variants of the <c:out>
tag where the square brackets indicate optional attributes and the curly bracers indicate a choice of options within
them where the default is underlined.
// Evaluate expression and output to current jspWriter
<c:out value="value" default="defaultValue" [escapeXml="{true|false}"]>
// Evaluate expression and output to current jspWriter defaulting to body content
<c:out var="varName" [escapeXml="{true|false}"]>
default value
</c:out>
<c:catch>
Top
The <c:catch>
tag allows us to catch an error within a JSP page before it propogates to a JSP error page or up the stack. The exception thrown is Throwable
which is right at the top of the exception hierarchy as
shown in the Java - Exception Hierarchy Diagram section, so only use the <c:catch>
tag for errors you
want to deal with within the JSP page itself.
The following code snippets shows the syntax of the <c:catch>
tag.
// Catch an error before it propogates to a JSP error page or up the stack
<c:catch var="exceptionNumber">
exception body
</c:catch>
General Purpose ExamplesTop
The following code shows examples of using general purpose tags within the Core
tag library.
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%-- Set a scoped object and property in request scope --%>
<c:set var="aVar" value="A stitch in time saves nine!" scope="request">
<%-- Output scoped object property value --%>
<c:out value="${requestScope.aVar}" default="Value not found!"/>
<%-- Remove a reference to a scoped object --%>
<c:remove var="aVar"scope="request">
<%-- Output scoped object property value --%>
<c:out value="${requestScope.aVar}" default="Value not found!"/>
<%-- Catch an exception --%>
<c:catch var="excNo">
<%
int num = 0;
out.write(6/num);
%>
</c:catch>
<p>Exception message is: ${excNo.message}</p>
The following screnshot shows the results of running the above JSP code.
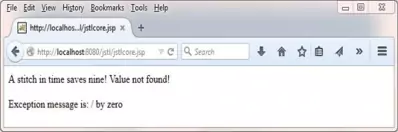
Conditional Core
ActionsTop
There are four conditional Core
actions, these being <c:if>
action which allows us to process the body content if a condition evaluates to true
, the <c:choose>
action which works in a similar way to a Java switch statement
when used in conjunction with the other two conditional actions; these being the <c:when>
action which acts like a Java
case
statement and the <c:otherwise>
action which acts like a Java default
statement.
Action / Attribute Name |
Mandatory | Run-time Expression? | Default Value | Object Type | Description |
---|---|---|---|---|---|
<c:if> | |||||
test | Yes | Yes | None | Boolean | Test condition to be tested for. If true body content is processed. |
var | No | No | None | String | Name of scoped variable referencing the value of the test condition |
scope | No | No | Page | String | Scope of the scoped variable specified by var . |
<c:choose> | |||||
The <c:choose> action contains no attributes although the body may contain one or more
<c:when> actions and zero or one <c:otherwise> actions | |||||
<c:when> | |||||
test | Yes | Yes | None | Boolean | Test condition to be tested for. If true body content is processed. |
<c:otherwise> | |||||
No attributes |
<c:if>
Top
The <c:if>
tag allows us to process the body content of the <c:if>
tag if a condition evaluates to true
and consists of two variants, with or without a body.
The following code snippets shows the two variants of the <c:if>
tag where the square brackets indicate optional attributes and the curly bracers indicate a choice of options within
them where the default is underlined.
// Evaluate expression and save test condition result to var
<c:if test="testCondition" var="varName" [scope="{page|request|session|application}"]>
// Evaluate expression and optionally save test condition result to var.
// Process body content if condition evaluates to true.
<c:if test="testCondition" [var="varName"] [scope="{page|request|session|application}"]>
body content to process
</c:if>
<c:choose>
Top
The <c:choose>
tag is used in conjunction with the <c:when>
and <c:otherwise>
tags and works in a similar way to the switch .. case .. default
Java conditional. There are two variants of the <c:choose>
tag as shown in the code snippets below:
// <c:choose>
and <c:when>
<c:choose>
<c:when test="S{expression to test}">
expression evaluates to true
</c:when>
<c:when test="S{expression to test}">
expression evaluates to true
</c:when>
</c:choose>
// <c:choose>
<c:when>
and <c:otherwise>
<c:choose>
<c:when test="S{expression to test}">
expression evaluates to true
</c:when>
<c:when test="S{expression to test}">
expression evaluates to true
</c:when>
<c:otherwise>
no expressions have evaluated to true
</c:otherwise>
</c:choose>
Conditional ExamplesTop
The following code shows examples of using the conditional tags within the Core
tag library.
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%-- Test an expression and process body if true --%>
<c:if test="${true}">
<p>Body content activated</p>
</c:if>
<%-- Test a number of expressions --%>
<c:choose>
<c:when test="${true and false}">
<p>First when was processed</p>
</c:when>
<c:when test="${false}">
<p>Second when was processed</p>
</c:when>
<c:otherwise>
<p>otherwise was processed</p>
</c:otherwise>
</c:choose>
The following screnshot shows the results of running the above JSP code.
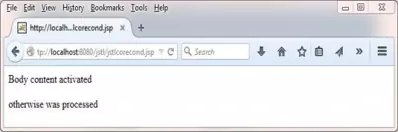
Iteration Core
ActionsTop
There are two iteration Core
actions, these being <c:forEach>
action which allows us iterate over a collection of objects or to iterate over the body content of the <c:forEach>
tag a set number of times (much like a for
loop) and the <c:forTokens>
action which allows us to do string tokenisation.
The indexes for the <c:forEach>
and <c:forTokens>
actions are zero-based.
Action / Attribute Name |
Manda |
Run-time Expression? | Default Value | Object Type | Description |
---|---|---|---|---|---|
<c:forEach> | |||||
var | No | No | None | String | Name of scoped variable referencing the current item within the iteration. |
items | No | Yes | None | Collec | Collection of objects to iterate over.
|
var | No | No | None | String | Name of scoped variable holding the status of the iteration which is of type javax.servlet. . |
begin | No | Yes | None | int | begin attribute must be >= 0.If items attribute specified then iteration begins at
object located at specified index.If items attribute not specified then iteration begins with value set by specified begin attribute. |
end | No | Yes | None | int | If items attribute specified then iteration ends after object located at specified index.If items attribute not specified then iteration ends at value set by specified end attribute. |
step | No | Yes | None | int | step attribute must be >= 1.Iteration will only process every step item within the collection. |
<c:forTokens> | |||||
var | No | No | None | String | Name of scoped variable referencing the current item within the iteration. |
items | Yes | Yes | None | String | The string of tokens to iterate over. |
delims | Yes | Yes | None | String | The delimiter set. |
var | No | No | None | String | Name of scoped variable holding the status of the iteration which is of type javax.servlet. . |
begin | No | Yes | None | int | begin attribute must be >= 0.Iteration begins with value set by specified begin attribute. |
end | No | Yes | None | int | Iteration ends at value set by specified end attribute. |
step | No | Yes | None | int | step attribute must be >= 1.Iteration will only process every step token within the string. |
<c:forEach>
Top
The <c:forEach>
tag allows us to iterate over a collection of objects or to iterate over the body content of the <c:forEach>
tag a set number of times (much like a for
loop).
We can iterate through a comma delimited String
object, an array of objects or array of primitives. We can also iterate over a java.util.Enumeration
or java.util.Iterator
, as well as all implementations of java.util.Map
and java.util.Collection
.
The following code snippets shows the two variants of the <c:forEach>
tag where the square brackets indicate optional attributes.
// Iterate over a collection of objects
<c:forEach items="collection" ["var="varName"] ["varStatus="varStatusName"]
["begin="begin"] ["end="end"] ["step="step"]>
body content
</c:forEach>
// Iterate over the body content (like a for loop)
<c:forEach ["var="varName"] ["begin="begin"] ["end="end"] ["step="step"]>
body content
</c:forEach>
<c:forTokens>
Top
The <c:forTokens>
tag allows us to iterate over a string of tokens that are delimited by the specified delimiters.
The following code snippets shows the syntax of the <c:forTokens>
tag where the square brackets indicate optional attributes.
// Iterate over a collection of objects
<c:forTokens items="stringOfTokens" delims="delimiters" "["var="varName"] ["varStatus="varStatusName"]
["begin="begin"] ["end="end"] ["step="step"]>
body content
</c:forTokens>
Iteration ExamplesTop
The following code shows an example of using the <c:forEach>
iteration tag of the Core
tag library.
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<table>
<%-- Iterate over request headers --%>
<c:forEach var="reqHeaders" items="${headerValues}">
<tr><th>The Value Of The Header Key</th><th>The Value Of The Header Value</th></tr>
<tr><td>${reqHeaders.key}</td><td>${reqHeaders.value[0]}</td></tr>
</c:forEach>
</table>
The following screnshot shows the results of running the above JSP code.
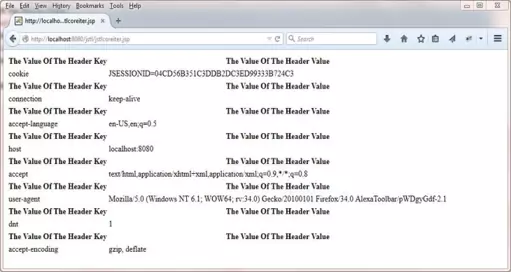
URL Related Core
ActionsTop
There are four URL related Core
actions, these being <c:import>
action which allows us to include request-time inclusion of other resources, the <c:url>
action which generates a URL for page output; the <c:redirect>
action which redirects the client to the specified URL and the <c:param>
action which is used to attach
parameters to the other three URL related actions.
Action / Attribute Name |
Manda |
Run-time Expression? | Default Value | Object Type | Description |
---|---|---|---|---|---|
<c:import> | |||||
url | Yes | Yes | None | String | The URL of the resource to import. |
context | No | Yes | None | String | Context name when accessing a relative URL resource belonging to a foreign context. |
var | No | No | None | String | Exported scoped variable name, with a type of String for the resource’s content. |
scope | No | No | Page | String | Scope of the scoped variable specified by var . |
char | No | Yes | ISO-8859-1 | String | Character encoding of the content at input resource. |
var | No | No | None | String | Exported scoped variable name, with a type of Reader for the resource’s content. |
<c:url> | |||||
value | No | Yes | None | String | The URL to be processed |
context | No | Yes | None | String | Context name when accessing a relative URL resource belonging to a foreign context. |
var | No | No | None | String | Exported scoped variable name, with a type of String for the resource’s content. |
scope | No | No | Page | String | Scope of the scoped variable specified by var . |
<c:redirect> | |||||
url | Yes | Yes | None | String | The URL of the resource to redirect to. |
context | No | Yes | None | String | Context name when accessing a relative URL resource belonging to a foreign context. |
<c:param> | |||||
name | No | Yes | None | String | Name of the query string parameter. |
value | No | Yes | None | String | Value of parameter. |
<c:import>
Top
The <c:import>
tag allows us to import the content of a URL-based resource just like the jsp:include
standard action. The difference is that the jsp:include standard action can only import resources from the same application, whereas
the <c:import>
tag allows us to import URL-based resources from different applications within the same container and even from an external resource outside the container.
The following code snippets shows the two variants of the <c:import>
tag where the square brackets indicate optional attributes.
// Resource content inlined or exported as a String object
<c:import url="url" [context="context"] [var="varName"]
[scope="{page|request|session|application}"] [charEncoding="charEncoding"]>
<c:param name="name" value="value">Somevalue</c:param>
</c:import>
// Resource content exported as a Reader object
<c:import url="url" [context="context"] varReader="varReaderName" [charEncoding="charEncoding"]>
body content where varReader is consumed by another action
</c:import>
<c:url>
Top
The <c:url>
tag allows us to build a URL with the proper rewriting rules applied, generally for reuse as a link within the JSP page.
The following code snippets shows the two variants of the <c:url>
tag where the square brackets indicate optional attributes.
// No body content
<c:url value="value" [context="context"] [var="varName"]
[scope="{page|request|session|application}"]>
// With body content to specify query string parameters
<c:url value="value" [context="context"] [var="varName"]
[scope="{page|request|session|application}"]>
<c:param name="name" value="value">Somevalue</c:param>
</c:url>
<c:redirect>
Top
The <c:redirect>
tag causes the client to redirect to the specified URL. The <c:import>
tag allows us to redirect to URL-based resources from different applications within the
same container and even to an external resource outside the container
The following code snippets shows the two variants of the <c:redirect>
tag where the square brackets indicate optional attributes.
// No body content
<c:redirect url="value" [context="context"]>
// With body content to specify query string parameters
<c:redirect url="value" [context="context"]>
<c:param name="name" value="value">Somevalue</c:param>
</c:redirect>
URL Related ExamplesTop
The following code shows examples of using the <c:import>
tag within the Core
tag library.
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%-- Import two resources from same application --%>
<c:import url="jstlcore.jsp">
<c:import url="jstlcorecond.jsp">
The following screnshot shows the results of running the above JSP code.
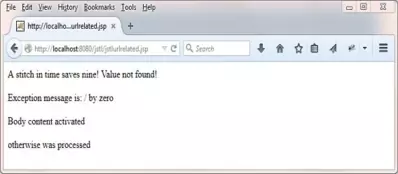
Lesson 2 Complete
In our first lesson on JSTL tag libraries we looked at the Core
tag library.
What's Next?
In our second lesson on JSTL tag libraries we look at the XML
tag library.