if
Construct QuizS2C Home « if
Construct Quiz
Fundamentals Quiz 11
The quiz below tests your knowledge of the material learnt in Fundamentals - Lesson 12 - if
Construct Quiz.
Question 1 : What will be output from this code snippet?
boolean a = false;
if (a = true) {System.out.println("true");}
else {System.out.println("false");}
- The snippet will output <code>true</code>. This is because <code>if ( a = true)</code> actually assigns <code>true</code> to 'a'.<br><code>if ( a == true)</code> would check <code>a</code> for equality and return <code>false</code>.
Quiz Progress Bar
Quiz 1
Code Structure & Syntax
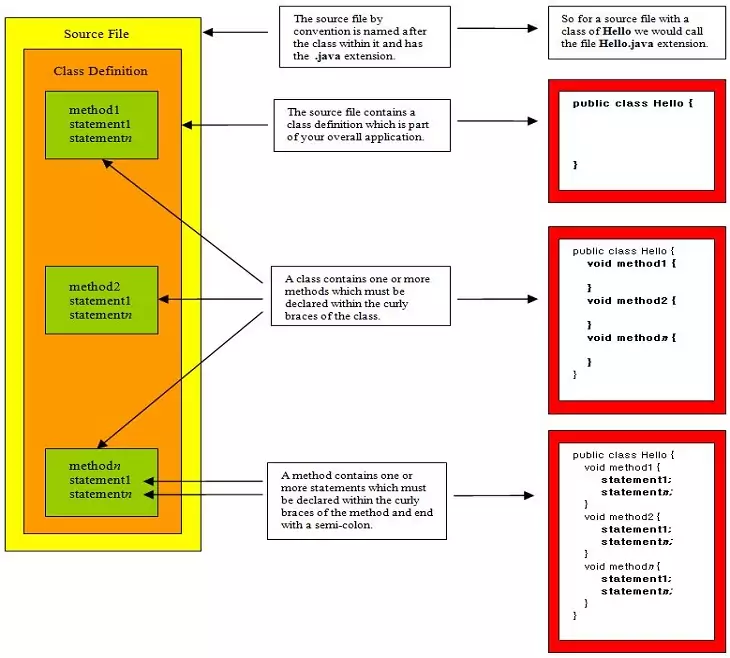
Quiz 2
Java Variables
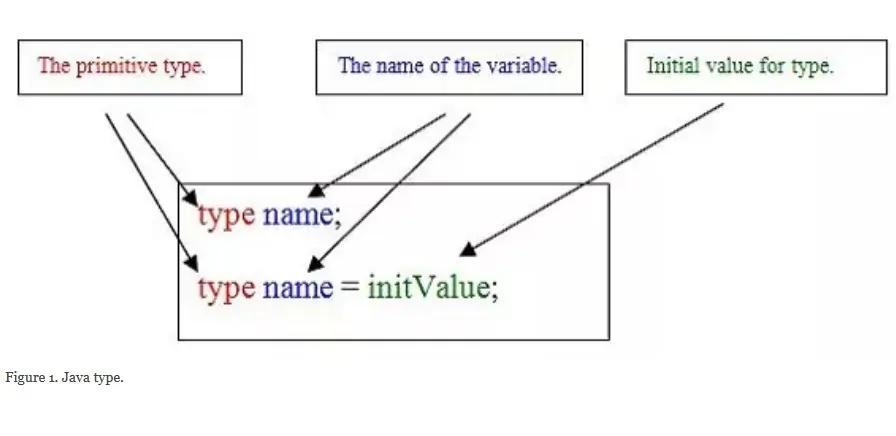
Quiz 3
Primitives - boolean
& char
data types
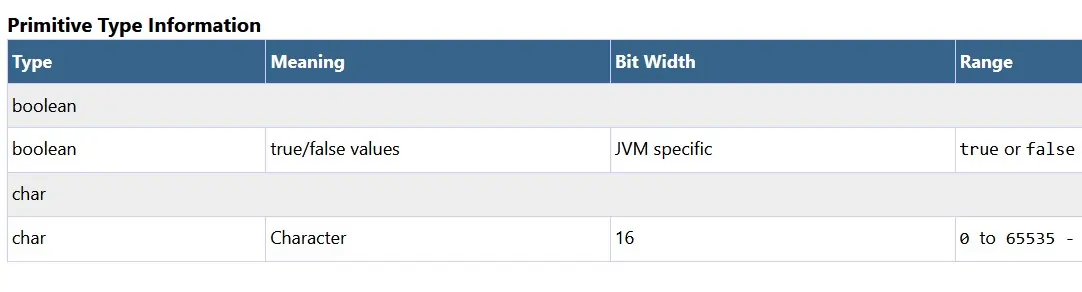
Quiz 4
Primitives - Numeric data types
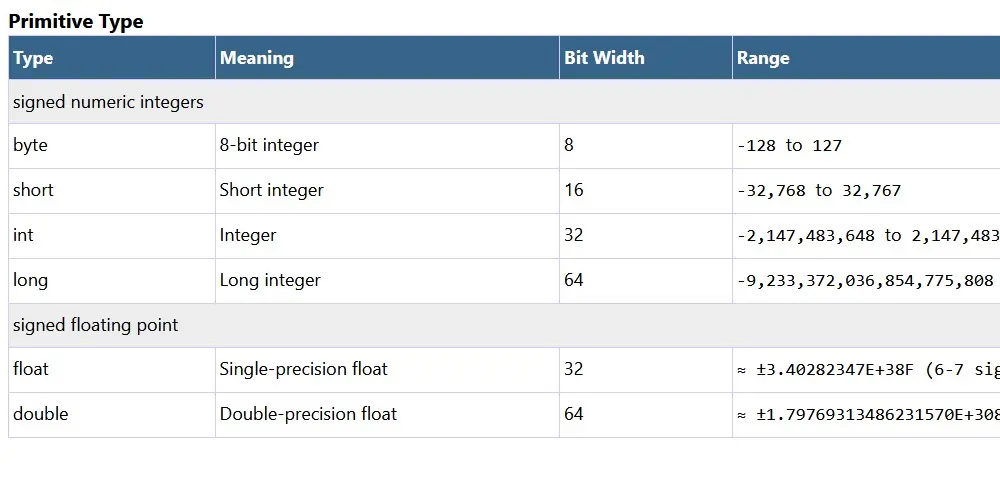
Quiz 5
Method Scope
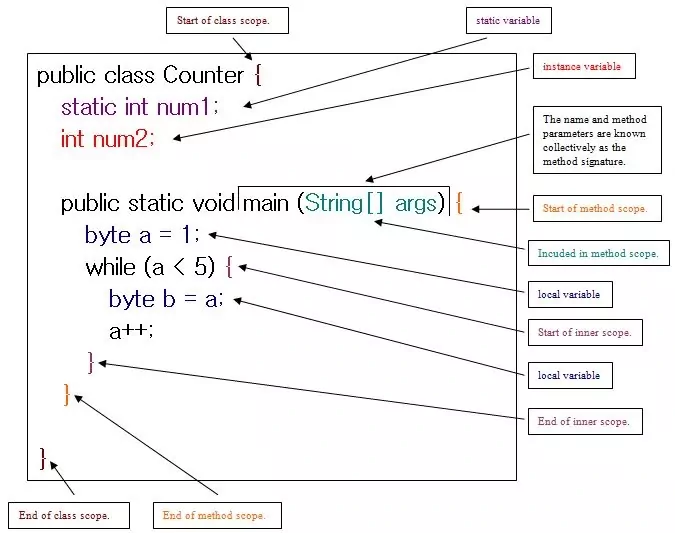
Quiz 6
Arithmetic Operators
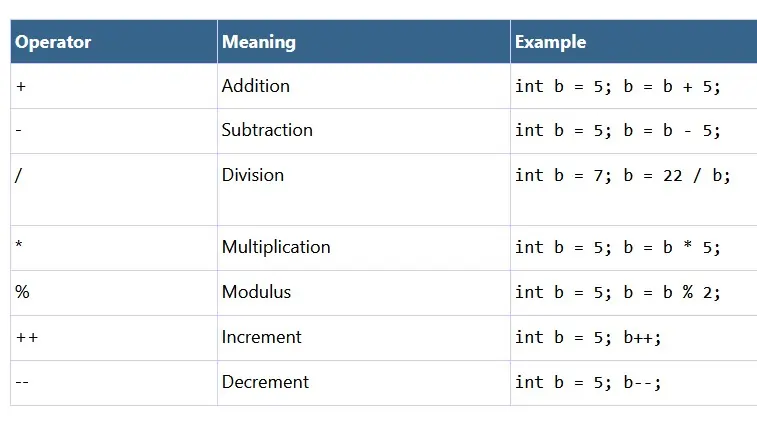
Quiz 7
Relational & Logical Operators
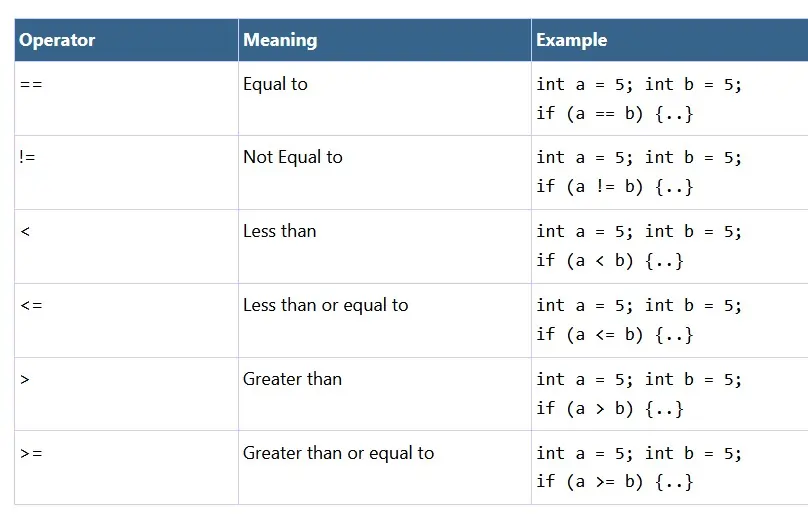
Quiz 8
Assignment Operators
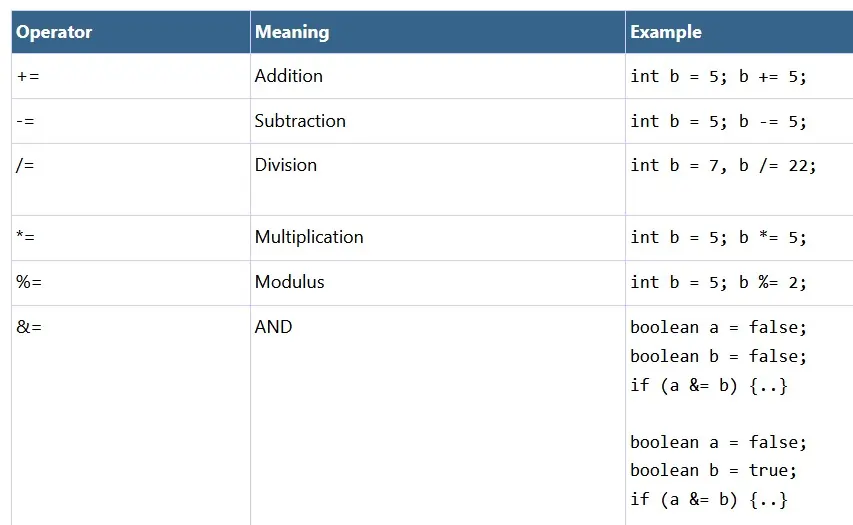
Quiz 9
Bitwise Logical Operators
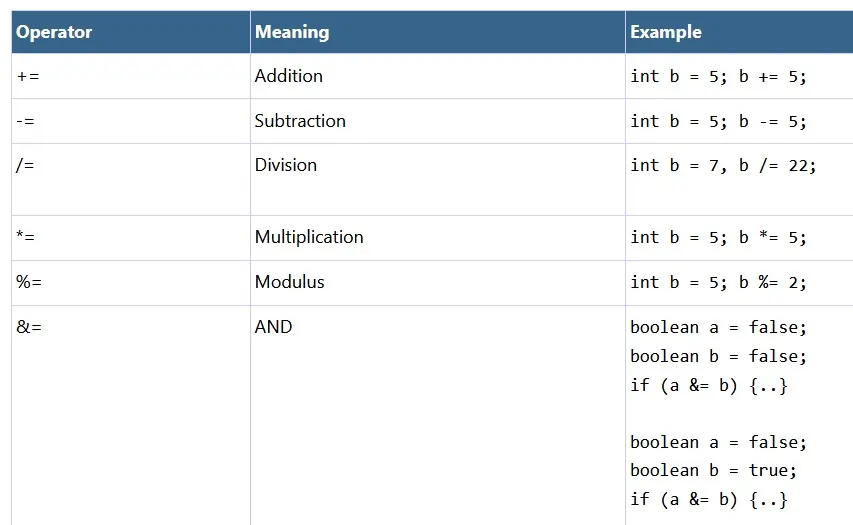
Quiz 10
Bitwise Shift Operators
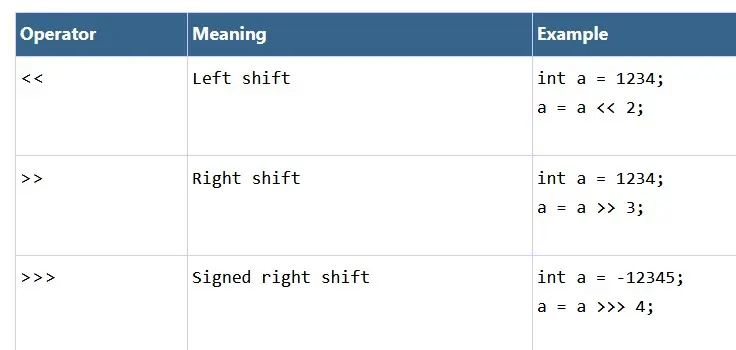
Quiz 12
switch
Construct
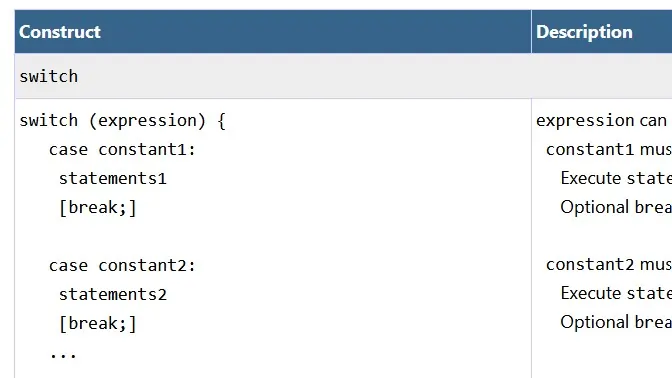
Quiz 13
for
Construct
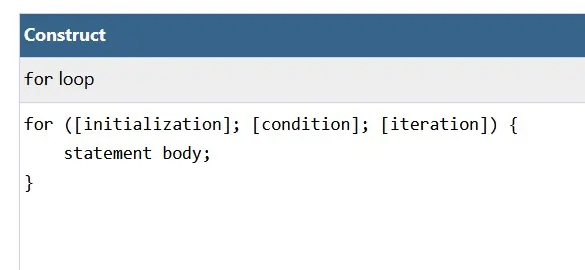
Quiz 14
while
Construct
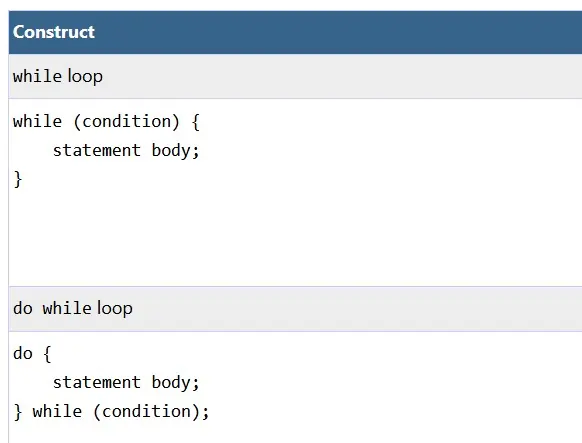
What's Next?
The next quiz on Java is all about Loop Statements.