Introduction to ELS2C Home « Introduction to EL
In this section we look at using the Expression Language (EL) and will be covering the 2.1 release. The main purpose of using EL is to remove Java syntax from JSP pages, thus making page creation more natural for non-Java programmers. EL was first introduced with JSP 2.0 and was packaged along with JSTL 1.0, and as such could be used by importing JSTL into applications. From JSP 2.0 onwards EL was incorporated into the JSP specification and as such we can use EL without importing JSTL into our applications.
EL can be used independently or in conjunction with standard and custom actions (JSTL) and allows us to author entirely script-free (no Java) JSP pages. Of course if so desired we could also use EL alongside Java scripting elements and although this goes against the philosophy of why EL was created, we will do so where relevant, to put points across in these lessons, or to make the learning easier.
EL SyntaxTop
An EL expression start with ${ and ends with } as shown below:
${expression}
EL expressions are always evaluated from left to right.
The following code snippet shows how to write the expression a+b
.
<!DOCTYPE html>
<html>
<head><title>Expression Evaluation</title></head>
<body>
<h1>Expression Evaluation</h1>
<%
request.setAttribute("a", 5);
request.setAttribute("b", 15);
%>
<p>Expression output: ${a+b}</p>
</body>
</html>
The above code produces a screen that looks like the following and as you can see the attribute values are added together:
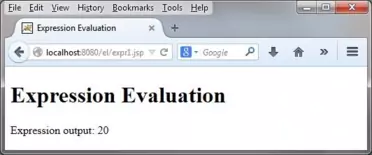
You can also concatenate expressions and these will be evaluated from left to right, coerced to String
objects and then concatenated. The following code snippet shows how to concatenate two
expressions.
<!DOCTYPE html>
<html>
<head><title>Expression Concatenation</title></head>
<body>
<h1>Expression Concatenation</h1>
<%
request.setAttribute("a", 5);
request.setAttribute("b", 15);
request.setAttribute("c", 6);
request.setAttribute("d", 3);
%>
<p>Expression output: ${a+b}${c+d}</p>
</body>
</html>
The above code produces a screen that looks like the following and as you can see the attribute values are added together within each expression and then concatenated together as a string:
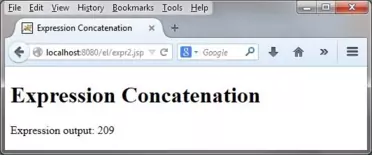
The following expression shows two expressions with a string literal in between them and again these will be evaluated from left to right, coerced to String
objects and then concatenated.
<!DOCTYPE html>
<html>
<head><title>Multiple Expressions With A String Literal</title></head>
<body>
<h1>Multiple Expressions With A String Literal</h1>
<%
request.setAttribute("a", 5);
request.setAttribute("b", 15);
request.setAttribute("c", 6);
request.setAttribute("d", 3);
%>
<p>Expression output: ${a+b} some text ${c+d}</p>
</body>
</html>
The above code produces a screen that looks like the following and as you can see the attribute values are added together within each expression and then concatenated together as a string:
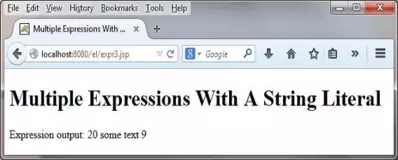
EL Naming Rules & Reserved WordsTop
These rules apply to all variables, as well as classes and methods (collectively known as identifiers)
- Must start with a letter, the underscore symbol (_) or the dollar sign ($). A name can't start with a number or any other symbol.
- You can use numbers after the first letter, you just can't start with a number.
- You cannot use reserved words recognized by the compiler. See the table below for the reserved words you can't use.
EL Reserved Words | |||
---|---|---|---|
and | div | empty | eq |
false | ge | gt | instanceof |
le | lt | mod | ne |
null | not | or | true |
EL LiteralsTop
There are five EL Literals that can be used within our JSP pages as shown in the following table:
Type | Examples | Notes |
---|---|---|
Boolean | ${true} ${false} | Valid values are true and false . |
Integer | ${12345} ${-12345} | Uses a java.lang.Long under the bonnet.Range: -9,233,372,036,854,775,808 to 9,233,372,036,854,775,807 |
Floating point | ${1.2345} ${1.23e+6} | Uses a java.lang.Double under the bonnet.64 bit Double-precision float. |
Null | ${null} | Same as Java null literal. |
String | ${'fred'} ${"margaret"} | Characters enclosed within single or double quotes. |
Lesson 1 Complete
In our first lesson on EL we looked at EL syntax, EL keywords and EL literals.
What's Next?
The next lesson is all about the symbols used in EL for mathematical and logical manipulation which are known as EL operators.