Other JSP Standard ActionsS2C Home « Other JSP Standard Actions
In our second lesson on JSP standard actions we look at the jsp:include
, jsp:forward
and jsp:param
standard actions and how to use them.
The jsp:include
and jsp:forward
standard actions are equivalent to the include
and forward
RequestDispatcher
mechanisms that we investigated in the
Redirects & Request Dispatching lesson but as with all standard actions allow us to code them purely with XML.
The jsp:param
standard action allows us to add additional parameters to the jsp:include
and jsp:forward
standard actions at request time.
jsp:include
Top
The jsp:include
standard action allows us to dynamically declare another resource to include in the current JSP page. The resources you can include using the jsp:include
standard action are
another JSP page, a HTML page or a servlet.
The following diagram shows the form of the jsp:include
standard action.
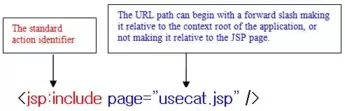
Before we look at an example of using the jsp:include
standard action lets address the differences between it and the include
directive we looked at in the JSP Directives lesson:
- Inclusion in a JSP page using the
jsp:include
standard action occurs at request time and so any changes to the included page will be included.- Inclusion in a JSP page using the
include
directive occurs at translation time when the container generates a servlet from the JSP page and so any changes to the included page may NOT be included but this is container specific.
- Inclusion in a JSP page using the
- The page to include using the
jsp:include
standard action must end with the.jsp
extension to be processed as a JSP page.- The page to include using the
include
directive doesn't have to end with the.jsp
extension to be processed as a JSP page.
- The page to include using the
- The page to include using the
jsp:include
standard action can be executed conditionally.- The page to include using the
include
directive will always be executed.
- The page to include using the
- The
jsp:include
standard action allows parameters to be passed at request time via thejsp:param
standard action.- The
include
directive has no mechanism for passing parameters.
- The
- The page to include using the
jsp:include
standard action doesn't have to exist until request time.- The page to include using the
include
directive must exist at translation time.
- The page to include using the
Hopefully you can see from the differences in the above lists that the jsp:include
standard action offers dynamic inclusion of content at request time, that can use be included conditionally and set via
an expression making this method of inclusion much more flexible than the more rigid rules of the include
directive inclusion mechanism.
Lets look at an example of using the jsp:include
standard action:
<!DOCTYPE html>
<html>
<head><title>Using jsp:include</title></head>
<body>
<h1>JSP Page That includes Another Resource Via jsp:include</p>
<jsp:useBean id="date" class="java.util.Date">
<%= date %>
<jsp:include page="usecat.jsp">
</body>
</html>
The following screenshot shows the results of deploying and running the above JSP page within the standardactions
folder within Tomcat:
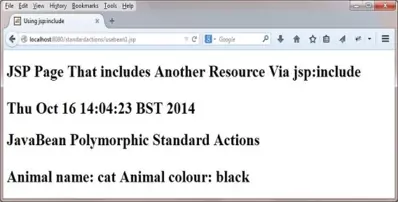
jsp:forward
Top
The jsp:forward
standard action is used to forward the current page to a different resource for processing.
The following diagram shows the form of the jsp:forward
standard action.
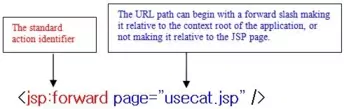
Lets look at an example of using the jsp:forward
standard action:
<!DOCTYPE html>
<html>
<head><title>Using jsp:forward</title></head>
<body>
<h1>JSP Page That Forwards To Another Resource Via jsp:forward</p>
<jsp:useBean id="date" class="java.util.Date">
<%= date %>
<jsp:forward page="usecat.jsp">
</body>
</html>
The following screenshot shows the results of deploying and running the above JSP page within the standardactions
folder within Tomcat:
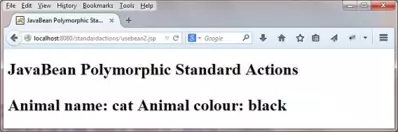
Did you notice from the above screenshot that the HTML header and the output from the expression <%= date %>
were not output? Using the jsp:forward
standard action transfers the
responsibility for outputting to the forwarded page, so anything in the forwarding page that was to be output before using the jsp:forward
standard action is effectively ignored.
jsp:param
Top
The jsp:param
standard action allows us to add one or more parameters within the body of the jsp:include
or jsp:forward
standard action.
The following diagram shows the form of the jsp:param
standard action.
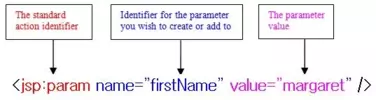
There are some points to remember when adding parameters to a request using the jsp:param
standard action:
- Paramaters added using the
jsp:param
standard action only exist for the duration of the page invoked using thejsp:include
orjsp:forward
standard action. - If a parameter already exists with the same name then any parameters added with the
jsp:param
standard action are added to the start of the existing parameter list.
The following JSP pages and output illustrate the above points:
First here's a forwarding page that passes a parameter which I called forwarding.jsp
.
<!DOCTYPE html>
<html>
<head><title>Forwarding Page</title></head>
<body>
<jsp:forward page="receiving.jsp" >
<jsp:param name="firstName" value="margaret">
</jsp:forward >
</body>
</html>
Now here's the forwarded page that I called receiving.jsp
that prints the parameters using a scriplet.
<!DOCTYPE html>
<html>
<head><title>Receiving Page</title></head>
<body>
<h1>Receiving Page That Prints Off Inbound Parameters</h1>
<%
String[] s = request.getParameterValues("firstName");
for (int i=0;i<s.length;i++) {
out.write("First name: " + s[i] + " | ");
}
%>
</body>
</html>
The following screenshot shows the results of deploying and running the the forwarding.jsp
JSP page with the following HTTP request:
http://localhost:8080/standardactions/forwarding.jsp?firstName=fred
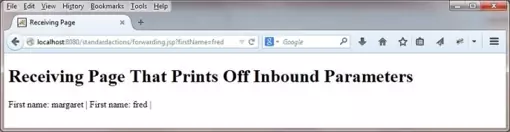
As you can see from the screenshot the firstName
parameter with the value of margaret
we added using the jsp:param
standard action is added to the start of the existing parameter list.
This ends our investigation of jsp technology and it's important to remember that JSPs are not a replacement for Java and Servlets but complement them. Think of these technologies as accompaniments to each other, where we use JSPs for our View and Java and Servlets for the Model and Controller; in fact all modern web applications that use the technologies will have a mixture of each.
Lesson 8 Complete
In this lesson we looked at the jsp:include
, jsp:forward
and jsp:param
standard actions.
What's Next?
That's it for the jsp part of the site. In the next section of the site we study the Expression language.