EL Implicit ObjectsS2C Home « EL Implicit Objects
We saw in the JSP Implicit Objects lesson how every JSP page comes with a collection of implicit objects we can use within that JSP page. The Expression Language (EL) also comes with its own set of implicit objects we can use within a JSP page and we look at these in detail in this lesson.
The table below shows all the EL implicit objects available to JSP authors and links to the sections within this lesson in which the EL implicit objects are discussed in greater detail:
Implicit Object | Type | Description |
---|---|---|
Cookie Information | ||
cookie | java.util.Map | A map containing all javax.servlet.http.Cookie objects using the names as the keys to each object. |
Header Information | ||
header | java.util.Map | A map containing all request headers using header names as the keys. First header value returned, for multiple values use headerValues . |
headerValues | java.util.Map | A map containing all request headers using header names as the keys. Values are held as an Array holding all values for relevant key. |
Page Context Information | ||
pageContext | javax.servlet. | A JavaBean containing all JSP Implicit Objects. |
Parameter Information | ||
initParam | java.util.Map | Map containing all context initialisation parameters using parameter names as the keys. |
param | java.util.Map | Map containing all parameter value using parameter names as the keys. First parameter value returned, for multiple values use paramValues . |
paramValues | java.util.Map | Map containing all parameter value using parameter names as the keys. Values are held as an Array holding all values for relevant key. |
Scoped Attributes | ||
applicationScope | java.util.Map | Map containing all context attributes within the ServletContext object using attribute names as the keys. |
sessionScope | java.util.Map | Map containing all session attributes within the HttpSession object using attributes names as the keys. |
requestScope | java.util.Map | Map containing all request attributes within the HttpServletRequest object using attribute names as the keys. |
pageScope | java.util.Map | Map containing all page attributes within page scope using attribute names as the keys. |
cookie
Implicit ObjectTop
The cookie
implicit object is of type java.util.Map
and can be used to access javax.servlet.http.Cookie
objects using the names as the keys to each object.
This is the same as using the getCookies()
method of the javax.servlet.http.HTTPServletRequest
object and iterating over it for the required name. Using the EL implicit object it's a lot
easier to code but of course is still specific to the HTTP protocol.
The following code snippet shows some cookie
implicit object usage and the various syntactical styles available using the .
and []
operators:
<%-- Using cookie Implicit Object --%>
${cookie.JSESSIONID.value}<br>
${cookie.JSESSIONID["value"]}<br>
${cookie["JSESSIONID"].value}<br>
${cookie["JSESSIONID"]["value"]}
The above code produces a screen that looks like the following:
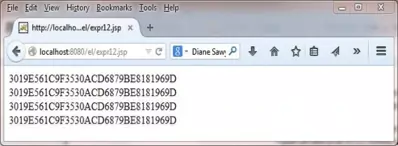
For more information on the javax.servlet.http.HTTPServletRequest
interface and the methods available take a look at the HttpServletRequest
Overview section.
header
Implicit ObjectTop
The header
implicit object is of type java.util.Map
and can be used to get request headers using header names as the keys. The first value within each header is returned, so to return
multiple values you would need to use the headerValues
implicit object instead.
This is equivalent to using the getHeader()
method of the javax.servlet.http.HttpServletRequest
object but without the scripting and of course using the EL implicit object is still specific to the HTTP protocol.
The following code snippet shows some header
implicit object use:
<%-- Using header Implicit Object --%>
${header.accept}<br>
${header["accept-encoding"]}<br>
${header["accept-language"]}<br>
${header["host"]}
The above code produces a screen that looks like the following:
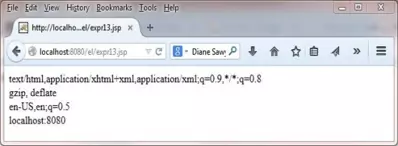
For more information on the javax.servlet.http.HTTPServletRequest
interface and the methods available take a look at the HttpServletRequest
Overview section.
headerValues
Implicit ObjectTop
The headerValues
implicit object is of type java.util.Map
and can be used to get request headers using header names as the keys.
This is equivalent to using the getHeaders()
method of the javax.servlet.http.HTTPServletRequest
object but without the scripting and of course using the EL implicit object is still specific to the HTTP protocol.
The following code snippet shows some headerValues
implicit object use:
<%-- Using headerValues Implicit Object --%>
${headerValues.accept[0]}<br>
${headerValues.accept[1]}<br>
${headerValues["accept-charset"][2]}<br>
${headerValues["content-type"][1]}
For more information on the javax.servlet.http.HTTPServletRequest
interface and the methods available take a look at the HttpServletRequest
Overview section.
pageContext
Implicit ObjectTop
The pageContext
implicit object is of type javax.servlet.jsp.PageContext
and is the container's implementation of the JSP page in question. The pageContext
implicit object
allows us to access all the regular Servlet objects via their JSP Implicit Objects.
This also means we can access Servlet objects using the pageContext
in tandem with the Expression Language as shown in the following code snippet.
<%-- Using pageContext Implicit Object With EL --%>
${pageContext.request.servletPath}<br>
${pageContext.request.method}<br>
${pageContext.session.id}
The above code produces a screen that looks like the following:
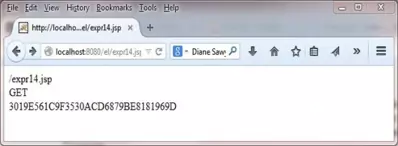
initParam
Implicit ObjectTop
The initParam
implicit object is of type java.util.Map
and can be used to retrieve context initialisation parameters using parameter names as the keys.
This is equivalent to using config.getServletContext.getInitParameter()
method of the javax.servlet.ServletConfig
object or using application.getInitParameter()
of the
javax.servlet.ServletContext
object but without the scripting.
The following code snippet shows some initParam
implicit object use:
<%-- Using initParam Implicit Object --%>
${initParam.someInitParam}<br>
${initParam["someInitParam"]}
For more information on the javax.servlet.ServletConfig
interface and the methods available take a look at the ServletConfig
Interface section.
For more information on the javax.servlet.ServletContext
interface and the methods available take a look at the ServletContext
Interface section.
param
Implicit ObjectTop
The param
implicit object is of type java.util.Map
and can be used to get parameter values using parameter names as the keys. The first value within each parameter is returned, so to return
multiple values you would need to use the paramValues
implicit object instead.
This is equivalent to using the getParameter()
method of the javax.servlet.http.HttpServletRequest
object but without the scripting.
The following code snippet shows some header
implicit object use:
<%-- Using param Implicit Object --%>
${param.someReqParam}<br>
${param["someReqParam"]}
For more information on the javax.servlet.ServletRequest
interface and the methods available take a look at the ServletRequest
Overview section.
paramValues
Implicit ObjectTop
The paramValues
implicit object is of type java.util.Map
and can be used to get request headers using header names as the keys.
This is equivalent to using the getParameterValues()
method of the javax.servlet.http.HTTPServletRequest
object but without the scripting.
The following code snippet shows some paramValues
implicit object use:
<%-- Using paramValues Implicit Object --%>
${paramValues.someReqParam[0]}<br>
${paramValues.someReqParam[1]}<br>
${paramValues["someReqParam"][2]}
For more information on the javax.servlet.ServletRequest
interface and the methods available take a look at the ServletRequest
Overview section.
applicationScope
Implicit ObjectTop
The applicationScope
implicit object is of type java.util.Map
and can be used to retrieve application (context) scoped attributes attached to the javax.servlet.ServletContext
object.
The following code snippet shows some applicationScope
implicit object use:
<%
pageContext.setAttribute("name", "charlie", PageContext.APPLICATION_SCOPE);
%>
<%-- Using applicationScope Implicit Object With EL --%>
${applicationScope.name}<br>
${applicationScope["name"]}
The above code produces a screen that looks like the following:
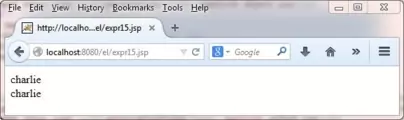
For more information on the javax.servlet.ServletContext
interface and the methods available take a look at the ServletContext
Interface section.
sessionScope
Implicit ObjectTop
The sessionScope
implicit object is of type java.util.Map
and can be used to retrieve session scoped attributes attached to the javax.servlet.http.HttpSession
object.
The following code snippet shows some sessionScope
implicit object use:
<%
pageContext.setAttribute("name", "charlie", PageContext.SESSION_SCOPE);
%>
<%-- Using sessionScope Implicit Object With EL --%>
${sessionScope.name}<br>
${sessionScope["name"]}
For more information on the javax.servlet.http.HttpSession
interface and the methods available take a look at the HttpSession
Interface section.
requestScope
Implicit ObjectTop
The requestScope
implicit object is of type java.util.Map
and can be used to retrieve request scoped attributes attached to the javax.servlet.ServletRequest
object.
The following code snippet shows some sessionScope
implicit object use:
<%
pageContext.setAttribute("name", "charlie", PageContext.REQUEST_SCOPE);
%>
<%-- Using requestScope Implicit Object With EL --%>
${requestScope.name}<br>
${requestScope["name"]}
For more information on the javax.servlet.ServletRequest
interface and the methods available take a look at the ServletRequest Overview section.
pageScope
Implicit ObjectTop
The pageScope
implicit object is of type java.util.Map
and can be used to retrieve page scoped attributes attached to the javax.servlet.jsp.PageContext
object.
The following code snippet shows some pageScope
implicit object use:
<%
pageContext.setAttribute("name", "charlie", PageContext.PAGE_SCOPE);
%>
<%-- Using pageScope Implicit Object With EL --%>
${pageScope.name}<br>
${pageScope["name"]}
Lesson 4 Complete
In this lesson we looked at EL implicit objects and their usage.
What's Next?
We finish our study of the Expression Language by looking at how we can configure our pages for script-free EL or non-EL use.