ListsS2C Home « Lists
In the second of three lessons on the different collection types within the Collection hierarchy we look at three of the concrete implementations of the List<E>
interface. So what type of
collection is a List? Lists are ordered collections that use an index for the ordering of the elements within the collection. As such lists have methods for the index that you don't find in other collection
types within the The Collections Framework. An element is added to a list by specifying the index position or to the end of the list when no index is specified.
List Hierarchy DiagramTop
The diagram below is a representation of the List hierarchy and covers the interfaces and classes we will study in this lesson. The diagram has several interfaces missing and also the
java.util.Stack<E>
concrete implemetation which is not covered on the site, but should help in visualisation:
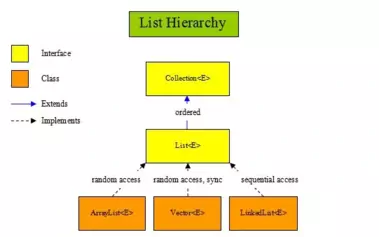
List Interfaces & ClassesTop
The table below gives a description of each interface and class within the above diagram. Click a link to go to detailed information about a particular interface or class:
Interface/Class | Description |
---|---|
Collection<E> | The root interface in the Collection hierarchy which the List<E> interface extends. There is no
direct implementation of the Collection<E> interface within the JDK . |
List<E> | Interface for ordered collections of elements. |
ArrayList<E> | Random access, resizable-array implementation of the List<E> interface that
implements all optional list operations and permits all elements, including null . |
Vector<E> | Synchronized random access resizable-array implementation of the List<E> interface. |
LinkedList<E> | Sequential access linked implementation of the List<E> interface that implements all optional list
operations, and permits all elements, including null . The class also implements the Queue<E> interface, providing first-in-first-out queue operations. |
List Types and OrderingTop
The table below extrapolates the commented information about ordering from the diagram above into a more readable tabular format:
Collection Type | Ordering | |
---|---|---|
List | Ordered | Sorted |
ArrayList<E> | By the index | No |
Vector<E> | By the index | No |
LinkedList<E> | By the index | No |
ArrayListsTop
An ArrayList is a resizable-array, ordered implementation of the List<E>
interface that permits all elements including null
. ArrayList allows random access to elements and is similar to
Vector apart from being unsynchronized.
This type of list is a good choice when you want fast access and iteration but are not doing a lot of insertions and deletions.
ArrayList<E>
Method OverviewTop
The table below shows the declarations of all the methods for the ArrayList<E>
class implemented from the List<E>
interface:
Method Declaration | Description |
---|---|
Used in the example below | |
public boolean add(E o) | Appends the specified element to the end of this ArrayList. |
public void add(int index, E element) | Inserts the specified element at the specified position in this ArrayList. |
public int indexOf(Object elem) | Searches for the first occurence of the given argument, testing for equality using the equals() method. |
public int lastIndexOf(Object elem) | Searches for the last occurence of the given argument in this ArrayList. |
public boolean remove(int index) | Removes the element at the specified index in this ArrayList. |
public int size() | Returns the number of elements in this ArrayList, ie. its cardinality. |
Not used in the example below | |
public boolean addAll(Collection<? extends E> c) | Adds all of the elements in the specified collection to this ArrayList. |
public boolean addAll(int index, | Adds all of the elements in the specified collection to this ArrayList, starting at the specified index . |
public void clear() | Removes all of the elements from this ArrayList. |
public Object clone() | Creates and returns a shallow copy of this ArrayList although the elements themselves are not cloned. |
public boolean contains(Object o) | Returns true if this ArrayList contains the specified element. |
public void ensureCapacity(int minCapacity) | Increases the capacity of this ArrayList instance if required, to ensure that it can hold at least the number of elements specified by minCapacity . |
public E get(int index) | Returns the element at the specified index in this ArrayList. |
public boolean isEmpty() | Returns true if this ArrayList contains no elements. |
public Iterator<E> iterator() | Returns an iterator over the elements in this ArrayList. |
public boolean remove(Object o) | Removes a single instance of the specified element from this ArrayList if it is present. |
protected void removeRange(int fromIndex, | Removes all elements whose index is between fromIndex inclusive, and toIndex exclusive, from this ArrayList. |
public E set(int index, E element) | Replaces the element at the specified index in this ArrayList with the specified element. |
public Object[] toArray() | Returns an array containing all of the elements in this ArrayList in the correct order. |
public <T> T[] toArray(T[] a) | Returns an array containing all of the elements in this ArrayList in the correct order; the runtime type of the returned array is that of the specified array. |
publicvoid trimToSize() | Trims the capacity of this ArrayList instance to be the current sizeof the ArrayList. |
Usage for the the first six methods is shown in the example below. For more information on the other methods in the ArrayList<E>
class the following link will take you to the online version of
documentation for the JavaTM 2 Platform Standard Edition 5.0 API Specification. Take a look at the documentation for the ArrayList<E>
class which you can find by scrolling down the lower left pane and clicking on ArrayList.
ArrayList<E>
ExampleTop
Lets write a simple class that creates an ArrayList and uses some of the methods from the ArrayList<E>
class:
/*
Simple class to create an ArrayList and use several methods from the class
*/
import java.util.*; // Import the java.util package
public class ArrayListClass {
public static void main (String[] args) {
List<Object> al = new ArrayList<Object>();
String s = "hello";
al.add(s);
al.add(new Integer(123));
al.add(s);
al.add("goodbye");
al.add(s+s);
al.add("goodbye");
System.out.println("array list size = " + al.size());
// Use enhanced for loop to iterate over the collection
for (Object o : al) {
System.out.println(o); // Print element
}
al.add(2, new Integer(123));
al.remove(4);
System.out.println("array list size = " + al.size());
System.out.println("first occurrence of s = " + al.indexOf(s));
System.out.println("last occurrence of s = " + al.lastIndexOf(s));
// Use enhanced for loop to iterate over the collection
for (Object o : al) {
System.out.println(o); // Print element
}
}
}
Save, compile and run the ArrayListClass
class in directory c:\_Collections in the usual way.
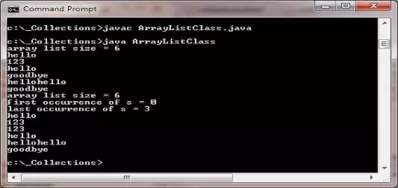
The above screenshot shows the results of creating and running our ArrayListClass
class and using several of the methods contained within the ArrayList<E>
class.
VectorsTop
A Vector is a resizable-array, ordered implementation of the List<E>
interface that permits all elements including null
. Vector allows random access to elements and is similar to
ArrayList apart from being synchronized. In fact Vector is one of the two original collections shipped with Java, the other being Hashtable. Vector was retrofitted to implement List<E>
interface, so that it became a part of The Collections Framework when the framework was introduced in version 1.2.
There is no sound reason since the introduction of the ArrayList<E>
class to use the Vector<E>
class. If you need an ArrayList to be synchronized this can be achieved using
methods of the Collections
class without all the overheads of using Vector<E>
synchronized methods.
Vector<E>
Method OverviewTop
The methods for the Vector<E>
class are very similar to those used for the ArrayList<E>
class apart from being synchrozied so we won't go into them here. If you want to
take a look at the Vector<E>
class methods the following link will take you to the online version of documentation for the JavaTM 2 Platform Standard Edition 5.0 API Specification.
Take a look at the documentation for the Vector<E>
class which you can find by scrolling down the lower left pane and clicking on Vector.
Vector<E>
ExampleTop
Lets write a simple class that creates a Vector and uses some of the methods from the Vector<E>
class:
/*
Simple class to create an Vector and use several methods from the class
*/
import java.util.*; // Import the java.util package
public class VectorClass {
public static void main (String[] args) {
List<Object> vec = new Vector<Object>();
String s = "hello";
vec.add(s);
vec.add(new Integer(123));
vec.add(s);
vec.add("goodbye");
vec.add(s+s);
vec.add("goodbye");
System.out.println("vector size = " + vec.size());
// Use enhanced for loop to iterate over the collection
for (Object o : vec) {
System.out.println(o); // Print element
}
vec.add(2, new Integer(123));
vec.remove(4);
System.out.println("vector size = " + vec.size());
System.out.println("first occurrence of s = " + vec.indexOf(s));
System.out.println("last occurrence of s = " + vec.lastIndexOf(s));
// Use enhanced for loop to iterate over the collection
for (Object o : vec) {
System.out.println(o); // Print element
}
}
}
Save, compile and run the VectorClass
class in directory c:\_Collections in the usual way.
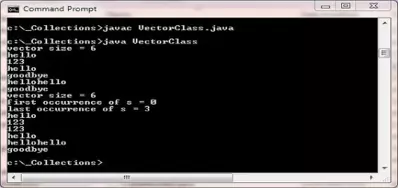
The above screenshot shows the results of creating and running our VectorClass
class and using several of the methods contained within the Vector<E>
class.
LinkedListsTop
A LinkedList is an ordered implementation of the List<E>
interface that is accessed sequentialy using a doubly-linked list running through all of its elements. Because of this, the sequential
access and the fact that the LinkedList<E>
class also implements the Queue<E>
interface, there are methods unique to this type of List.
This type of list is a good choice when you are doing a lot of insertions and deletions and because of the implementation of the Queue<E>
interface is also a good candidate for stacks and queues.
LinkedList<E>
Method OverviewTop
The table below shows the declarations of all the methods for the LinkedList<E>
class implemented from the List<E>
and Queue<E>
interfaces:
Method Declaration | Description |
---|---|
Used in the example below | |
public boolean add(E o) | Appends the specified element to the end of this LinkedList. |
public void add(int index, E element) | Inserts the specified element at the specified position in this LinkedList. |
public int size() | Returns the number of elements in this ArrayList, ie. its cardinality. |
Not used in the example below | |
public boolean addAll(Collection<? extends E> c) | Adds all of the elements in the specified collection to this LinkedList. |
public boolean addAll(int index, | Adds all of the elements in the specified collection to this LinkedList, starting at the specified index . |
public boolean addFirst(E o) | Adds the specified element to the start of this LinkedList. |
public boolean addLast(E o) | Appends the specified element to the end of this LinkedList. |
public void clear() | Removes all of the elements from this LinkedList. |
public Object clone() | Creates and returns a shallow copy of this LinkedList although the elements themselves are not cloned. |
public boolean contains(Object o) | Returns true if this LinkedList contains the specified element. |
public E element(E o) | Returns the first element of this LinkedList. |
public E get(int index) | Returns the element at the specified index in this LinkedList. |
public E getFirst() | Returns and removes the first element of this LinkedList. |
public E getLast() | Returns and removes the last element of this LinkedList. |
public int indexOf(Object elem) | Returns the index position within the LinkedList of the first occurrence of the specified element, or -1 if not found. |
public boolean isEmpty() | Returns true if this ArrayList contains no elements. |
public int lastIndexOf(Object elem) | Returns the index position within the LinkedList of the last occurrence of the specified element, or -1 if not found. |
public ListIterator<E> listIterator(int index) | Returns a correct order list-iterator of the elements in this LinkedList, starting at the specified index within the LinkedList. |
public boolean offer(E o) | Appends the specified element to the end of this LinkedList. |
public E peek() | Returns the first element of this LinkedList. |
public E poll() | Returns and removes the first element of this LinkedList. |
public E remove() | Returns and removes the first element of this LinkedList. |
public E remove(int index) | Removes the element at the specified index in this LinkedList. |
public E removeFirst(E o) | Removes the first element of this LinkedList. |
public E removeLast(E o) | Removes the last element of this LinkedList. |
public boolean remove(Object o) | Removes a single instance of the specified element from this ArrayList if it is present. |
public E set(int index, E element) | Replaces the element at the specified index in this LinkedList with the specified element. |
public Object[] toArray() | Returns an array containing all of the elements in this LinkedList in the correct order. |
public <T> T[] toArray(T[] a) | Returns an array containing all of the elements in this LinkedList in the correct order; the runtime type of the returned array is that of the specified array. |
Usage for the the first three methods is shown in the example below. For more information on the other methods in the LinkedList<E>
class the following link will take you to the online version of
documentation for the JavaTM 2 Platform Standard Edition 5.0 API Specification. Take a look at the documentation for the LinkedList<E>
class which you can find by scrolling down the lower left pane and clicking on LinkedList.
LinkedList<E>
ExampleTop
Lets write a simple class that creates a LinkedList and uses some of the methods from the LinkedList<E>
class:
/*
Simple class to create a LinkedList and use several methods fron from the class
*/
import java.util.*; // Import the java.util package
public class LinkedListClass {
public static void main (String[] args) {
List<Object> ll = new LinkedList<Object>();
String s = "hello";
ll.add(s);
ll.add(new Integer(123));
ll.add(s);
ll.add("goodbye");
ll.add(s+s);
System.out.println("linked list size = " + ll.size());
System.out.println("linked list contains: " + ll);
ll.add(3, new Integer(123));
System.out.println("linked list size = " + ll.size());
System.out.println("linked list contains: " + ll);
}
}
Save, compile and run the LinkedListClass
class in directory c:\_Collections in the usual way.
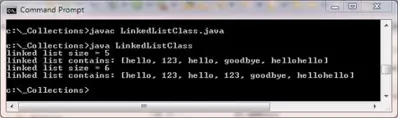
The above screenshot shows the results of creating and running our LinkedListClass
class.
Lesson 4 Complete
In this lesson we looked at Lists.
What's Next?
In our final lesson on the different collection types within the Collection hierarchy we look at the PriorityQueue<E>
concrete implementation of the Queue<E> interface.