Inheritance BasicsS2C Home « Inheritance Basics
Inheritance is a language mechanism, that allows us to group common characteristics together into a set of related items, which we can then redefine into more specific subsets. In Java terminology, the general class that holds the group of common characteristics is known as the superclass and the specialised subset of this is known as the subclass. We achieve this hierarchial connection in Java using the extends
keyword, wherein the subclass extends the superclass and is discussed in the Inheritance - Using extends
lesson.
By extending like this we have created an inheritance hierarchy where the subclass inherits the traits of the superclass, in other words the members of the superclass, assuming the access modifiers permit inheritance.
To begin looking at inheritance we will create a scenario where we start with one class and expand on this as we work through various aspects and concepts of using inheritance. So lets start by coding a Vehicle
class:
Vehicle
superclass Top
Here we code and test the Vehicle
class which is just a normal class like any other we have seen so far. This class holds all the generic stuff our yet to be developed subclasses will need all in one place, thus avoiding code duplication.
package com.server2client;
/*
A Vehicle Class
*/
public class Vehicle {
private String chassis;
private String motor;
private int wheels;
/*
Public getters and Setters
*/
public String getChassis() {
return chassis;
}
public void setChassis(String chassis) {
this.chassis = chassis;
}
public String getMotor() {
return motor;
}
public void setMotor(String motor) {
this.motor = motor;
}
public int getWheels() {
return wheels;
}
public void setWheels(int wheels) {
this.wheels = wheels;
}
/*
Instance Methods
*/
public void service(int month) {
System.out.println("Our vehicle needs servicing every " + month + " months.");
}
public void carry(int carry) {
System.out.println("Our vehicle carries " + carry + " passengers.");
}
public void load(String load) {
System.out.println("Our vehicle is loaded with " + load);
}
}
Ok our instance variables have the private
access modifier so these members are only accessible within the Vehicle
class or through their getters and setters. Now lets test our new Vehicle
class:
package com.server2client;
/*
Test Class for Vehicle
*/
public class TestVehicle {
public static void main (String[] args) {
Vehicle saloon = new Vehicle();
saloon.setChassis("2-axle chassis");
saloon.setMotor("4 stroke");
saloon.setWheels(4);
System.out.println("Our vehicle has a " + saloon.getChassis() + ", " + saloon.getMotor()
+ " motor and has " + saloon.getWheels() + " wheels.");
saloon.service(12);
saloon.carry(4);
saloon.load("shopping.");
}
}
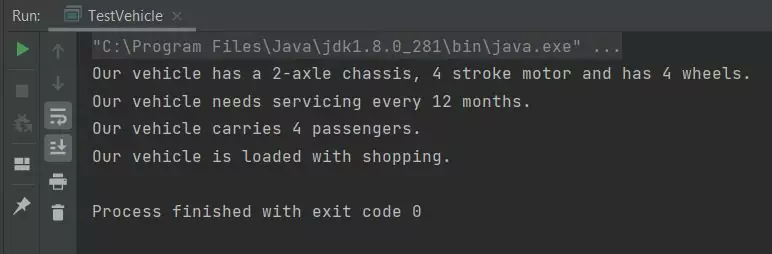
TestVehicle
class.The above screenshot shows the output of running our TestVehicle
class.
Related Quiz
OO Concepts Quiz 3 - Inheritance - Basics
Lesson 3 Complete
In this lesson we introduced the OO concept of Inheritance, what it means and how we start to use it.
What's Next?
We continue our investigation of inheritance by looking at subclassing using the extends
keyword.