JSTL Functions
Tag LibraryS2C Home « JSTL Functions
Tag Library
In our final lesson on JSTL tag libraries we look at the Functions
tag library. The Functions
tag library consists of sixteen actions. The fn:length
tag is used
for returning the length of a collection or for returning the number of characters within a string. The other fifteen Functions
tags are all used for string manipulation.
Array Function
ActionsTop
There are two array Function
actions, fn:join
which joins all elements of an array into a string and fn:split
which splits a string into an array of substrings.
Action / Attribute Name |
Object Type | Description |
---|---|---|
< | ||
array | String[] | The array of strings to be joined. |
separator | String | String to separate each element of the array in the resulting string. |
result | String | All array elements joined into one string. |
< | ||
string | String | Input string to be split into an array of strings. |
delimiters | String | Delimiter characters used to split the string. |
result | String[] | The resultant array of strings. |
fn:join
Top
The fn:join
tag joins all elements of an array into a string.
If the array
attribute is null
, an emptry string is returned.
If the separator
attribute is null
, it is processed as an emptry string.
If the separator
attribute is an empty string, the elements are joined together without any separator.
fn:join(array, separator) -> String
fn:split
Top
The fn:split
tag splits a string into an array of substrings.
If the string
attribute is null
, it is processed as an emptry string.
If the string
attribute is an empty string, a one element array is returned consisting of an emptry string.
If the delimiters
attribute is null
, it is processed as an emptry string.
If the delimiters
attribute is an empty string, a one element array is returned consisting of the original string.
fn:split(string, delimiters) -> String
Array ExamplesTop
The following code shows examples of using the fn:join
and fn:split
tags.
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<p>Function Library fn:join and fn:split Examples</p>
<c:set var="split" value='${fn:split("A Stitch in TIME saves NINE", " ")}'>
<table>
<c:forEach var="substring" items="${split}">
<tr><td>${substring}</tr></td>
</c:forEach>
</table>
<p>Join array into String: ${fn:join(split, "*")}<br></p>
The following screnshot shows the results of running the above JSP code.
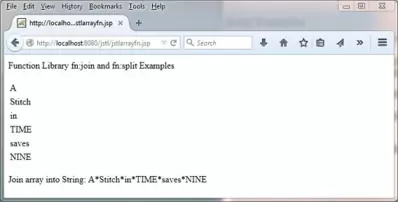
Capitalization Function
ActionsTop
There are two capitalization Function
actions, fn:toLowerCase
and fn:toUpperCase
which convert all characters of a string to lowercase and uppercase respectively.
Action / Attribute Name |
Object Type | Description |
---|---|---|
< | ||
string | String | Input string to transform to lower case. |
result | int | Input string transformed to lower case. |
< | ||
string | String | Input string to transform to upper case. |
result | int | Input string transformed to upper case. |
fn:toLowerCase
Top
The fn:toLowerCase
tag transforms the contents of a string to lower case.
If the string
attribute is null
it is treated as an emptry string and returned as such.
fn:toLowerCase(input) -> integer
fn:toUpperCase
Top
The fn:toUpperCase
tag transforms the contents of a string to upper case.
If the string
attribute is null
it is treated as an emptry string and returned as such.
fn:toUpperCase(input) -> integer
Capitalization ExamplesTop
The following code shows examples of using the fn:toLowerCase
and fn:toUpperCase
tags.
<%@taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<p>Function Library fn:toLowerCase and fn:toUpperCase Examples</p>
<p>String Transformed To Lower Case: ${fn:toLowerCase("A Stitch in TIME saves NINE")}<br></p>
<p>String Transformed To Upper Case: ${fn:toUpperCase("A Stitch in TIME saves NINE")}<br></p>
The following screnshot shows the results of running the above JSP code.
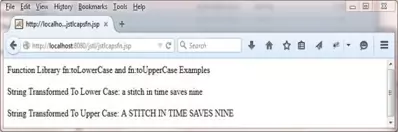
Contains Function
ActionsTop
There are five contains Function
actions these being fn:indexOf
which returns the index within a string of the first occurrence of a specified substring, fn:startsWith
which
tests if a string starts with the specified prefix, fn:endsWith
which tests if a string ends with the specified suffix, fn:contains
which tests if a string contains the specified substring and
fn:containsIgnoreCase
which tests if a string contains the specified substring in a case insensitive way.
Action / Attribute Name |
Object Type | Description |
---|---|---|
< | ||
string | String | Input string to which the substring function is applied. |
substring | String | Substring to search for within the input string. |
result | int | If the value of the substring attribute is a substring of the input string returns the index of the first character of the first such substring
occurrenceIf the value of the substring attribute does not occur as a substring then -1 is returned. |
< | ||
string | String | Input string to which the function is applied. |
prefix | String | Prefix to be matched against. |
result | boolean | Returns true if the value of the prefix attribute is the prefix of the character sequence represented by the string attribute, otherwise returns false . |
< | ||
string | String | Input string to which the function is applied. |
suffix | String | Suffix to be matched against. |
result | boolean | Returns true if the value of the prefix attribute is the suffix of the character sequence represented by the string attribute, otherwise returns false . |
< | ||
string | String | Input string to which the substring function is applied. |
substring | String | Substring to be etsted for. |
result | boolean | Returns true if character sequence of substring attribute exists in character sequence represented by the string attribute, otherwise returns false . |
< | ||
string | String | Input string to which the substring function is applied. |
substring | String | Substring to be etsted for. |
result | boolean | Returns true if character sequence of substring attribute exists in character sequence represented by the string attribute ignoring case differences, otherwise returns false . |
fn:indexOf
Top
The fn:indexOf
tag returns the index (0-based) first occurrence of a specified substring within a string.
If the string
attribute is null
, the input is treated as an empty string and is returned as such.
If the substring
attribute is is null
, it is processed as an empty string.
If the substring
attribute is empty, this matches the beginning of the string and 0
is returned.
fn:indexOf(string, substring) -> INT
fn:startsWith
Top
The fn:startsWith
tag tests if a string starts with the specified prefix.
If the string
attribute is null
, it is processed as an empty string.
If the prefix
attribute is null
, it is processed as an empty string.
fn:startsWith(string, prefix) -> boolean
fn:endsWith
Top
The fn:endsWith
tag tests if a string ends with the specified suffix.
If the string
attribute is null
, it is processed as an empty string.
If the suffix
attribute is null
, it is processed as an empty string.
If the suffix
attribute is empty this matches the end of the string and true
is returned.
fn:endsWith(string, suffix) -> boolean
fn:contains
Top
The fn:contains
tag tests if a string contains the specified substring.
If the string
attribute is null
, it is processed as an empty string.
If the substring
attribute is is null
, it is processed as an empty string.
If the substring
attribute is empty this matches the beginning of the string and true
is returned.
fn:contains(string, substring) -> string
fn:containsIgnoreCase
Top
The fn:containsIgnoreCase
tag tests if a string contains the specified substring in a case insensitive way.
If the string
attribute is null
, it is processed as an empty string.
If the substring
attribute is is null
, it is processed as an empty string.
If the substring
attribute is empty this matches the beginning of the string and true
is returned.
fn:containsIgnoreCase(string, substring) -> string
Contains ExamplesTop
The following code shows examples of using the fn:indexOf
, fn:startsWith
, fn:endsWith
, fn:contains
and fn:containsIgnoreCase
tags.
<%@taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<p>fn:indexOf, fn:startsWith, fn:endsWith, fn:contains and fn:containsIgnoreCase Examples<br></p>
<p>fn:indexOf - Index position: ${fn:indexOf("123456789", "56")}<br></p>
<p>fn:indexOf - Substring empty: ${fn:indexOf("123456789", "")}<br></p>
<p>fn:startsWith - True returned: ${fn:startsWith("123456789", "123")}<br></p>
<p>fn:startsWith - False returned: ${fn:startsWith("123456789", "234")}<br></p>
<p>fn:endsWith - True returned: ${fn:endsWith("123456789", "9")}<br></p>
<p>fn:contains - False returned: ${fn:contains("abcdef", "CDE")}<br></p>
<p>fn:containsIgnoreCase - True returned: ${fn:containsIgnoreCase("abcdef", "CDE")}<br></p>
The following screnshot shows the results of running the above JSP code.
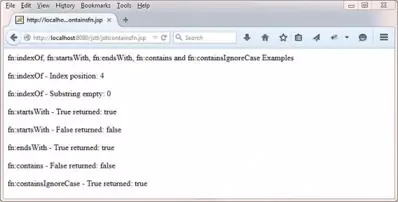
Escape XML Function
ActionTop
There is one escape XML Function
action fn:escapeXml
which can be used to escape characters that could be interpreted as XML markup.
Action / Attribute Name |
Object Type | Description | |||
---|---|---|---|---|---|
< | |||||
string | String | Input string on which the conversion is applied. | |||
result | String | Converted string. |
fn:escapeXml
Top
The fn:escapeXml
tag is used to escape characters that could be interpreted as XML markup.
If the string
attribute is null
, it is processed as an emptry string.
fn:escapeXml(string) -> string
Escape XML ExamplesTop
The following code shows examples of using the fn:escapeXml
tag.
<%@taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<p>Function Library fn:escapeXml Examples</p>
<p>Convert HTML markup: ${fn:escapeXml("<table><tr><td>Some data</tr></td></table>")}<br></p>
<p>Convert characters: ${fn:escapeXml("<>")}<br></p>
Length Function
ActionTop
There is one length Function
action fn:length
which can be used for returning the length of a collection or for returning the number of characters within a string.
Action / Attribute Name |
Object Type | Description | |||
---|---|---|---|---|---|
< | |||||
input | Supported type for items attribute in <c:forEach>
action, or String | Input collection or string on which the length is to be computed. | |||
result | int | Length of the collection or the string. |
fn:length
Top
The fn:length
tag returns the length of a collection or returns the number of characters within a string.
If the input
attribute is null
the input is treated as an empty collection and the value returned is 0
.
If the input
attribute is an empty string, the value returned is 0
.
fn:length(input) -> integer
Length ExamplesTop
The following code shows examples of using the fn:length
tag.
<%@taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<p>Function Library fn:length Examples</p>
<p>Size of collection: ${fn:length(headerValues)}<br></p>
<p>Characters in string: ${fn:length("1 2 3 4 5 6 7")}<br></p>
The following screnshot shows the results of running the above JSP code.
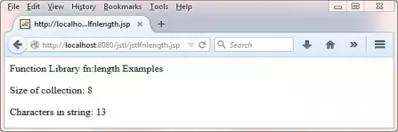
Replace Function
ActionTop
There is one replace Function
action fn:replace
which returns a string resulting from replacing all occurrences of a before substring within an input string, into occurrences of an after substring.
Action / Attribute Name |
Object Type | Description | |||
---|---|---|---|---|---|
< | |||||
inputString | String | Input string on which the replace function is applied. | |||
beforeSubstring | String | The before substring to be replaced. | |||
afterSubstring | String | The after substring used to replace the before substring. | |||
result | String | The string resulting from replacing the string value held within the beforeSubstring attribute with the string value held within the afterSubstring attribute. |
fn:replace
Top
The fn:replace
tag returns a string resulting from replacing all occurrences of a before substring within an input string, into occurrences of an after substring.
If the inputString
attribute is null
the input is treated as an empty string and is returned as such.
If the beforeSubstring
attribute is an empty string, it is processed as an empty string.
If the afterSubstring
attribute is an empty string, all occurrences of the beforeSubstring
attribute are removed from the inputString
attribute.
fn:replace(inputString, beforeSubstring, afterSubstring) -> string
Replace ExamplesTop
The following code shows examples of using the fn:replace
tag.
<%@taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<p>Function Library fn:replace Examples</p>
<p>Replacing Occurrences: ${fn:replace("123456789", "456", "789")}<br></p>
<p>Before substring empty: ${fn:replace("123456789", "", "789")}<br></p>
<p>After substring empty: ${fn:replace("123456789", "456", "")}<br></p>
The following screnshot shows the results of running the above JSP code.
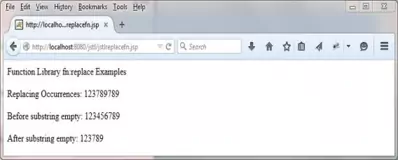
String Subset Function
ActionsTop
There are three string subset Function
actions these being fn:substring
which returns a subset of a string, fn:substringAfter
which returns a subset of a string following a
specific substring and fn:substringBefore
which returns a subset of a string before a specific substring.
Action / Attribute Name |
Object Type | Description |
---|---|---|
< | ||
string | String | Input string to which the substring function is applied. |
beginIndex | int | The beginning index (0-based), inclusive. |
endIndex | int | The ending index (0-based), inclusive. |
result | String | Substring of the input string. |
< | ||
string | String | Input string to which the substring function is applied. |
substring | String | Substring delimiting beginning of the subset of the input string to be returned. |
result | String | Substring of the input string. |
< | ||
string | String | Input string to which the substring function is applied. |
substring | String | Substring delimiting beginning of the subset of the input string to be returned. |
result | String | Substring of the input string. |
fn:substring
Top
The fn:substring
tag returns a subset of a string.
If the string
attribute is null
it is treated as an emptry string and returned as such.
If the beginIndex
attribute is greater than the last index of the input string an emptry string is returned.
If the beginIndex
attribute is less than 0
it is adjusted to 0
.
If the endIndex
attribute is less than 0
or greater than the length of the input string, its value is adjusted to be the length of the input string.
If the endIndex
attribute is less than the beginIndex
attribute an emptry string is returned.
fn:substring(string, beginIndex, endIndex) -> String
fn:substringAfter
Top
The fn:substringAfter
tag returns a subset of a string following a specific substring. The substring returned starts at the first character after the substring matched in the input string, and extends up to the end of the input string.
If the string
attribute is null
it is treated as an emptry string and returned as such.
If the substring
attribute is null
it is treated as an emptry string and returned as such.
If the string
attribute is an emptry string it is returned as such.
If the substring
attribute is an emptry string it matches the beginning of the input string and the input string is returned.
If the substring
attribute does not occur in the input string then an empty stringis returned.
fn:substringAfter(string, substring) -> String
fn:substringBefore
Top
The fn:substringBefore
tag returns a subset of a string before a specific substring. The substring returned starts at the first character in the input string and extends up to the character just before the substring matched in the input string.
If the string
attribute is null
it is treated as an emptry string and returned as such.
If the substring
attribute is null
it is treated as an emptry string and returned as such.
If the string
attribute is an emptry string it is returned as such.
If the substring
attribute is an emptry string it matches the beginning of the input string and an empty string is returned.
If the substring
attribute does not occur in the input string then an empty string is returned.
fn:substringBefore(string, substring) -> String
String Subset ExamplesTop
The following code shows examples of using the fn:substring
, fn:substringAfter
and fn:substringBefore
tags.
<%@taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<p>Function Library fn:substring, fn:substringAfter and fn:substringBefore Examples</p>
<p>Return subset of string: ${fn:substring("A Stitch in TIME saves NINE", 5, 10)}<br></p>
<p>Return subset of string after: ${fn:substringAfter("A Stitch in TIME", "Stit")}<br></p>
<p>Return subset of string before: ${fn:substringBefore("A Stitch in TIME", "IM")}<br></p>
The following screnshot shows the results of running the above JSP code.
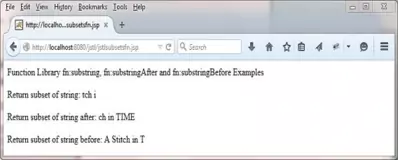
Trimming Function
ActionTop
There is one trimming Function
action fn:trim
which can be used to remove white space from both ends of a string.
Action / Attribute Name |
Object Type | Description | |||
---|---|---|---|---|---|
< | |||||
string | String | Input string on which the trim is applied. | |||
result | String | The trimmed string. |
fn:trim
Top
The fn:trim
tag removes white space from both ends of a string.
If the string
attribute is null
the input is treated as an empty string and is returned as such.
If the input
attribute is an empty string, the value returned is 0
.
fn:trim(string) -> string
Trimming ExamplesTop
The following code shows examples of using the fn:trim
tag.
<%@taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn" %>
<p>Function Library fn:trim Examples</p>
<p>Trimming both ends: ${fn:trim(" BOOM ")}<br></p>
<p>Trimming end: ${fn:trim("KABOOM ")}<br></p>
The following screnshot shows the results of running the above JSP code.
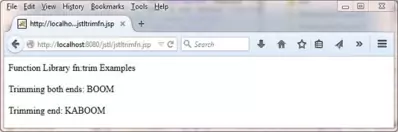
Lesson 6 Complete
In our final lesson on JSTL tag libraries we looked at the Functions
tag library.
What's Next?
In the next lesson we look at creating our own custom tags for when the functionality we need is not present in the JSTL tag libraries.