JSTL II8n
Tag LibraryS2C Home « JSTL II8n
Tag Library
In our third lesson on JSTL tag libraries we look at the II8n
tag library. The II8n
tag library consists of actions that allow us to format and internationalise currencies, numbers, percentages, dates and times.
Each II8n
action can have mandatory attributes that have to be populated and each attribute may also allow a run-time expression to be used. If a run-time expression is allowed for an attribute this can be
populated using a static string or more interestingly a dynamic value which can be an EL or Java expression or a value set using the <jsp:attribute>
standard action. If no run-time expression is
allowed for an attribute then you can only populate that attribute using a static string.
Number II8n
ActionsTop
There are two number II8n
actions for formatting and parsing numbers, these being <fmt:formatNumber>
which allows us to format a currency, number or percentage to our specifications
and <fmt:parseNumber>
which allows us to parse a string representation of currency, number or percentage.
Action / Attribute Name |
Manda |
Run-time Expression? | Default Value | Object Type | Description |
---|---|---|---|---|---|
<fmt:formatNumber> | |||||
value | Yes | Yes | None | String or Number | Numeric value to be formatted. |
type | No | Yes | number | String | Specify whether value is to be formatted as a number, currency or percent. |
pattern | No | Yes | None | String | Custom pattern for formatting. |
currency | No | Yes | None | String | ISO 4217 1 currency code which is only applicable when type attribute is 'currency', otherwise this attribute is ignored. |
currency | No | Yes | None | String | ISO 4217 1 currency symbol which is only applicable when type attribute is 'currency', otherwise this attribute is ignored. |
grouping | No | Yes | true | boolean | Specifies whether formatted output will contain grouping separators. |
max | No | Yes | None | int | Maximum number of digits in integer portion of the formatted output. |
min | No | Yes | None | int | Minimum number of digits in integer portion of the formatted output. |
max | No | Yes | None | int | Maximum number of digits in fractional portion of the formatted output. |
min | No | Yes | None | int | Minimum number of digits in fractional portion of the formatted output. |
var | No | No | None | String | Name of exported scoped variable to hold expression value. The type of scoped variable is String . |
scope | No | No | page | String | Scope that the created scoped variable var exists in. |
<fmt:parseNumber> | |||||
value | No | Yes | None | String | String value to be parsed. |
type | No | Yes | number | String | Specify whether value is to be formatted as a number, currency or percent. |
pattern | No | Yes | None | String | Custom pattern for formatting string value held within the value attribute. |
parse | No | Yes | None | String | Locale whose default formatting pattern is to be used during the parse operation, or to which the pattern specified via pattern attribute is applied. |
integer | No | Yes | true | boolean | Specifies whether just the integer portion of the given value should be parsed. |
var | No | No | None | String | Name of exported scoped variable to hold expression value. The type of scoped variable is Number . |
scope | No | No | page | String | Scope that the created scoped variable var exists in. |
1: For a full list of valid ISO 4217
codes follow this wikipedia link.
<fmt:formatNumber>
Top
The <fmt:formatNumber>
tag formats a numeric value in a locale-sensitive or customized manner as a number, currency, or percentage.
The numeric value to be formatted can be specified using the value
attribute or when omitted read from <fmt:formatNumber>
tag’s body content. The formatting pattern may be specified
via the pattern
attribute and if present takes precedence over the type
attribute and must adhere to the pattern syntax rules spcifed in the java.text.DecimalFormat
class.
Formatted results using the <fmt:formatNumber>
tag are output to the current JspWriter
object, unless the var
attribute is specified, in which case formatted results are stored in the named scoped variable.
The following code snippets shows the two variants of the <fmt:formatNumber>
tag where the square brackets indicate optional attributes and the curly bracers indicate a choice of options within
them where the default is underlined.
// No body content
<fmt:formatNumber value="numericValue" [type="{number|currency|percent}"] [pattern="customPattern"]
[currencyCode="currencyCode"] [currencySymbol="currencySymbol"] [groupingUsed="{true|false}"]
[maxIntegerDigits="maxIntegerDigits"] [minIntegerDigits="minIntegerDigits"]
[maxFractionDigits="maxFractionDigits"] [minFractionDigits="minFractionDigits"]
[var="varName"] [scope="{page|request|session|application}"]>
// Body content specifying numeric value to be formatted
<fmt:formatNumber [type="{number|currency|percent}"] [pattern="customPattern"]
[currencyCode="currencyCode"] [currencySymbol="currencySymbol"] [groupingUsed="{true|false}"]
[maxIntegerDigits="maxIntegerDigits"] [minIntegerDigits="minIntegerDigits"]
[maxFractionDigits="maxFractionDigits"] [minFractionDigits="minFractionDigits"]
[var="varName"] [scope="{page|request|session|application}"] >
numeric value to be formatted
</fmt:formatNumber>
<fmt:parseNumber>
Top
The <fmt:parseNumber>
tag parses the string representation of numbers, currencies, and percentages that were formatted in a locale-sensitive or customized manner.
The numeric value to be parsed can be specified using the value
attribute or when omitted read from <fmt:parseNumber>
tag’s body content. The formatting pattern may be specified
via the pattern
attribute and if present takes precedence over the type
attribute and must adhere to the pattern syntax rules spcifed in the java.text.DecimalFormat
class.
Formatted results using the <fmt:parseNumber>
tag are output to the current JspWriter
object using java.lang.Number.toString()
, unless the var
attribute is
specified, in which case formatted results are stored in the named scoped variable.
The following code snippets shows the two variants of the <fmt:parseNumber>
tag where the square brackets indicate optional attributes and the curly bracers indicate a choice of options within
them where the default is underlined.
// No body content
<fmt:parseNumber value="numericValue" [type="{number|currency|percent}"] [pattern="customPattern"]
[parseLocale="parseLocale"] [integerOnly="{true|false}"]
[var="varName"] [scope="{page|request|session|application}"]>
// Body content specifying numeric value to be parsed
<fmt:parseNumber [type="{number|currency|percent}"] [pattern="customPattern"]
[parseLocale="parseLocale"] [integerOnly="{true|false}"]
[var="varName"] [scope="{page|request|session|application}"] >
numeric value to be parsed
</fmt:parseNumber>
Number II8n
ExamplesTop
The following code shows examples of using general purpose tags within the Core
tag library.
<%@taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
<%-- Output formatted number --%>
Number: <fmt:formatNumber value="123456789"><br>
<%-- Output formatted number with maximum fraction digits --%>
Maximum Fraction Number: <fmt:formatNumber value="123456789" maxIntegerDigits="6"><br>
<%-- Output formatted number with minimum digits --%>
Minimum Digits Number: <fmt:formatNumber value="123456789" minFractionDigits="3"><br>
<%-- Output formatted number using a pattern --%>
Pattern Number: <fmt:formatNumber value="123456.789" pattern="#,#00.0#"><br>
<%-- Output formatted number with default currency type --%>
Default Currency: <fmt:formatNumber value="12345" type="currency"><br>
<%-- Output formatted number with Japanese currency type --%>
Japanese Currency: <fmt:formatNumber value="12345" type="currency" currencyCode="JPY"><br>
<%-- Output precentage number--%>
Percentage: <fmt:formatNumber value="45" type="percent"><br>
The following screnshot shows the results of running the above JSP code.
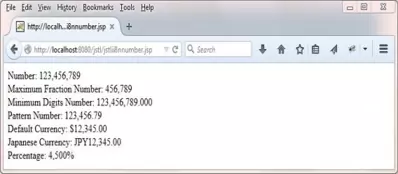
Date II8n
ActionsTop
There are two date II8n
actions for formatting and parsing dates, these being <fmt:formatDate>
which allows us to format a dates to our specifications
and <fmt:parseDate>
which allows us to parse a string representation of a date and time in locale-sensitive format.
Action / Attribute Name |
Manda |
Run-time Expression? | Default Value | Object Type | Description |
---|---|---|---|---|---|
<fmt:formatDate> | |||||
value | Yes | Yes | None | java.util. | Date and/or time to be formatted. |
type | No | Yes | date | String | Specify whether value is to be formatted as a date or time or both. |
date | No | Yes | default | String | Predefined formatting style for dates that follow the semantics defined in java.text. . |
time | No | Yes | default | String | Predefined formatting style for times that follow the semantics defined in java.text. . |
pattern | No | Yes | None | String | Custom pattern for formatting dates and times. |
timeZone | No | Yes | None | String or java.util. | The time zone used to represent the time. |
var | No | No | None | String | Name of exported scoped variable to hold expression value. The type of scoped variable is String . |
scope | No | No | page | String | Scope that the created scoped variable var exists in. |
<fmt:parseDate> | |||||
value | Yes | Yes | None | java.util. | Date and/or time to be formatted. |
type | No | Yes | date | String | Specify whether value is to be formatted as a date or time or both. |
dateStyle | No | Yes | default | String | Predefined formatting style for dates that follow the semantics defined in java.text. . |
timeStyle | No | Yes | default | String | Predefined formatting style for times that follow the semantics defined in java.text. . |
pattern | No | Yes | None | String | Custom pattern for formatting dates and times. |
timeZone | No | Yes | None | String or java.util. | The time zone used to represent the time. |
parse | No | Yes | None | String or java.util. | Locale whose predefined formatting styles are to be used during the parse
operation, or to which any present pattern attribute is applied. |
var | No | No | None | String | Name of exported scoped variable to hold expression value. The type of scoped variable is String . |
scope | No | No | page | String | Scope that the created scoped variable var exists in. |
<fmt:formatDate>
Top
The <fmt:formatDate>
tag allows us to which allows us to format a dates to our specifications or to locale-sensitive formats.
If the scope
attribute is specified the var
attribute must also be specified. If the value
attribute is null
or empty, nothing is written to the current JspWriter
object and any scoped variable specified is removed. If the timeZone
attribute is null
or empty, it is handled as if it wasn't specified.
If the <fmt:formatDate>
tag fails to determine a formatting locale, it uses java.util.Date.toString()
for formatting any output.
The following code snippet shows the syntax of the <fmt:formatDate>
tag where the square brackets indicate optional attributes and the curly bracers indicate a choice of options within
them where the default is underlined.
// Format a date
<fmt:formatDate value="date" [type="{date|time|both}"]
[dateStyle="{default|short|medium|long|full}"]
[timeStyle="{default|short|medium|long|full}"]
[pattern="customPattern"] [timezone="timezone"]
[var="varName"] [scope="{page|request|session|application}"]>
<fmt:parseDate>
Top
The <fmt:parseDate>
tag is used to parse the string representation of dates and times that were formatted in a locale-sensitive or customized manner.
If the date string to be parsed is null
or empty, the scoped variable defined by var
and scope
are removed, allowing "empty" input to be distinguished from "invalid" input,
which causes an exception. If the timeZone
attribute is null
or empty, it is handled as if it wasn't specified. If the parseLocale
attribute is null
or empty, it
is handled as if it wasn't specified. If an exception occurs during parsing of the value, it must be caught and rethrown as a JspException
.
If the <fmt:formatDate>
tag fails to determine a formatting locale, it uses java.util.Date.toString()
for formatting any output.
There are two variants of the <fmt:parseDate>
tag, with or without a body, as shown in the code snippets below:
// No body content
<fmt:parseDate value="dateString" [type="{date|time|both}"]
[dateStyle="{default|short|medium|long|full}"]
[timeStyle="{default|short|medium|long|full}"]
[pattern="customPattern"] [timezone="timezone"] [parseLocale="parseLocale"]
[var="varName"] [scope="{page|request|session|application}"]>
// Body content specifying date value to be parsed
<fmt:parseDate [type="{date|time|both}"]
[dateStyle="{default|short|medium|long|full}"]
[timeStyle="{default|short|medium|long|full}"]
[pattern="customPattern"] [timezone="timezone"] [parseLocale="parseLocale"]
[var="varName"] [scope="{page|request|session|application}"] >
numeric value to be parsed
</fmt:parseDate>
Date II8n
ExamplesTop
The following code shows examples of using general purpose tags within the Core
tag library.
<%@taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
<%-- Get current date and time --%>
<jsp:useBean id="now" class="java.util.Date">
<%-- Output formatted date --%>
Current Date: <fmt:formatDate value="${now}"><br>
<%-- Output formatted time --%>
Current Time: <fmt:formatDate value="${now}" type="time"><br>
<%-- Output formatted date and time --%>
Current Date and Time: <fmt:formatDate value="${now}" type="both"><br>
<%-- Output long date --%>
Current Long Date: <fmt:formatDate value="${now}" dateStyle="long"><br>
<%-- Output full time --%>
Current Full Time: <fmt:formatDate value="${now}" type="time" timeStyle="full"><br>
The following screnshot shows the results of running the above JSP code.
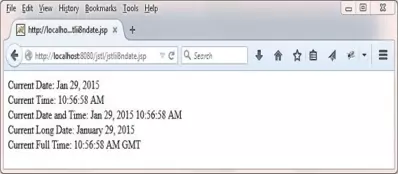
Time Zone II8n
ActionsTop
There are two time zone II8n
actions, these being <fmt:timeZone>
action which allows us to specify the time zone in which time information is to be formatted or parsed in its
body content and the <fmt:setTimeZone>
action which allows us to store the specified time zone in either a scoped variable or the time zone configuration variable.
Action / Attribute Name |
Manda |
Run-time Expression? | Default Value | Object Type | Description |
---|---|---|---|---|---|
<fmt:timeZone> | |||||
value | Yes | Yes | None | String orjava.util. | The time zone where a string value is interpreted as a time zone ID. |
<fmt:set | |||||
value | Yes | Yes | None | String orjava.util. | The time zone where a string value is interpreted as a time zone ID. |
var | No | No | None | String | Name of exported scoped variable to hold expression value. The type of scoped variable is java.util. . |
scope | No | No | page | String | Scope that the created scoped variable var exists in. |
<fmt:timeZone>
Top
The <fmt:timeZone>
action allows us to specify the time zone in which time information is to be formatted or parsed in its body content.
The following code snippets shows the syntax of the <fmt:timeZone>
tag.
// Specify time zone
<fmt:timeZone value="timeZone" >
body content
</fmt:timeZone>
<fmt:setTimeZone>
Top
The <fmt:setTimeZone>
tag allows us to store the specified time zone in either a scoped variable or the time zone configuration variable.
The following code snippets shows the syntax of the <fmt:setTimeZone>
tag.
// Store time zone
<fmt:setTimeZone value="timeZone" [var="varName"] [scope="{page|request|session|application}"]>
Time Zone II8n
ExamplesTop
The following code shows examples of using general purpose tags within the Core
tag library.
<%@taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
<%-- Get time --%>
<jsp:useBean id="now" class="java.util.Date">
<%-- Specify time zone US Eastern Standard Time and output current date --%>
<fmt:timeZone value="EST" >
Current Full Date and Time EST: <fmt:formatDate value="${now}" type="both"
dateStyle="full" timeStyle="full">
</fmt:timeZone>
The following screnshot shows the results of running the above JSP code.
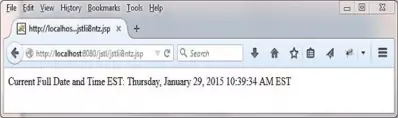
Lesson 4 Complete
In our third lesson on JSTL tag libraries we looked at the Ii8n
tag library.
What's Next?
In our fourth lesson on JSTL tag libraries we look at the Database
tag library.