Using HTML FormsS2C Home « Using HTML Forms
Allowing users to enter information, whether to place an order, enquire about something on the site or for many other reasons is fundamental to web applications and sites often contain many such forms. In this lesson we learn how to create HTML forms, interrogate field values passed back to our servlets from HTML forms and how to action an appropriate response to form input. It is not an intention of this lesson to validate form input or persist form information as we will be covering these subjects in later lessons.
If you need to brush up on the various elements contained within HTML forms there are several lessons and references covering these in the HTML section of the site. The rest of the lesson assumes you are comfortable with HTML form elements and so we will not be discussing these in any great detail. What we do need to know are the values passed from elements within a HTML form to our servlets when the user submits the form and we will be discussing this shortly.
The first thing we need to do when using HTML forms is to send the HTML form to the user for data input and this is easily achieved by placing the HTML form construction code within the doGet()
method. This way when the user requests the resource we can present them with a HTML form for data entry. After user entry we can then process the HTML form using the doPost()
method. This
is achieved, using a form of the syntax below the next paragraph, within the HTML form sent to the user via the doGet()
method.
Using the doPost()
method for validation of user entry means we can separate our coding into both methods rather than having everything in the doGet()
method which makes for
cleaner code. The added benefit of using the doPost()
method is that information from the HTML form gets submitted in the request body thus ensuring that any sensitive information is not
visible in the passed URL.
<form action="servletPath" method="post">"
...
</form>
Although it not the intention of this site to teach HTML syntax there are a number of points that should be raised about the above syntax with regards to our servlets/JSPs:
- The
action
attribute can be left out or an empty string.
Omitting this attribute or puttingaction=""
will send the submitted form to the same URL used for the request. - If a path is specified in the
action
attribute, this can point to either a static or dynamic resource. - The
method
attribute is optional and will default toget
, can be in upper or lower case and must be a valid HTTP method.
If themethod
attribute is left out then your servlet must contain adoGet()
method or you will get an exception.
Form Request ParametersTop
Knowing what is passed to our servlets on submission, when a user has interacted with particular HTML form elements, is vital to coding our user responses and we will talk about this before we code our
first HTML form. Values entered within a HTML form are sent to the server as request parameters which we can retrieve using methods of the ServletRequest
object. The following table lists
the various HTML form elements we can interact with and how we can get to their values using ServletRequest
methods.
Form Element | ServletRequest Method |
Notes |
---|---|---|
input (checkbox) | getParameter(name) | If a checkbox is checked then "on" is sent to the server. If a checkbox is unchecked then nothing is sent to the server and method returns null . |
input (text/hidden/ password/textarea) | getParameter(name) | The value of the input field is sent to the server. If the input field is empty then an empty string is sent to the server. |
input (radio button) | getParameter(name) | If a radio button is selected then the value of that button is sent to the server. If a radio button is not selected then nothing is sent to the server and method returns null . |
input (multiple elements with same name) | getParameter(name) | All values are sent to the server as an array of String objects.This method will retrieve the last one on the form. This method will retrieve all of them. |
select (single value) | getParameter(name) | If an option is selected then the value of that option is sent to the server. If no options are selected then the displayed option is sent to the server. |
select (multi value) | getParameterValues(name) | If multiple options are selected then the value of these options are sent to the server as an array of String objects.If no options are selected then nothing is sent to the server and method returns null . |
Creating a HTML Form ServletTop
What we are going to do for our first servlet that uses a HTML form is create a very basic form for ordering a take away. When the servlet is first actioned we will present the user with a form where
they enter their name, address, meal selection and delivery method. When the submit
button is pressed we will display some information pertaining to user entry.
Folder SetupTop
The first thing we are going to do in our setup is to make a base folder called _ServletsInt
in the root directory of our hard drive which we can put all web applications for this section into.
Lets create a folder for the servlets for this section, in Windows this would be:
double click My Computer icon
double click C:\ drive (or whatever your local drive is)
right click the mouse in the window
from the drop down menu select New
click the Folder option and call the folder _ServletsInt and press enter.
Within the _ServletsInt
folder we will create separate folders for each web application we use and our first web application will be called foodorder
Within the foodorder
folder we will create separate folders to hold our DD, source files and our compiled byte code and these will be called dd
, src
and classes
. We
don't need view
and lib
folders for the early web applications and so we won't bother creating these until we need them.
Within the src
folder create a subfolder called controller
, we don't need a model
folder for the web applications in this section.
So after creating these folders your directory structure should look something like the following screenshot:
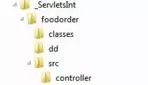
Coding FoodOrderServlet
Top
We are now ready to code up our servlet that presents a form for user entry and then displays information about what has been entered. The following code is very similar to the servlets we have written
up to now. The major difference is we are using the doGet()
to create a user form and a doPost()
to display information from the form as a response to the user. We also take a
first look at using some of the ServletRequest
parameter methods for retrieving information from forms:
Cut and paste the following code into your text editor and save it in the c:\_ServletsInt\foodorder\src\controller directory as FoodOrderServlet.java
.
package controller;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class FoodOrderServlet extends HttpServlet {
private static final long serialVersionUID = 871964L;
@Override
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Create a form for a user to enter takeaway details on
response.setContentType("text/html");
PrintWriter writer = response.getWriter();
writer.print("<html><head><title>The Take Away</title></head>" +
"<body><h1>Food Order Form</h1><form action='' method='post'>" +
"<fieldset style='background-color:silver;border:1px solid black;" +
"padding:5px;text-align:left;width:600px;'>" +
"<legend>Customer Information and Food Options</legend>" +
"<table><tr><td>Name:</td><td><input name='name'/></td></tr>" +
"<tr><td>Address:</td>" +
"<td><input name='addr' style='width:300px;'/></td></tr>" +
"<tr><td></td><td><input name='addr' style='width:300px;'/></td></tr>" +
"<tr><td></td><td><input name='addr' style='width:300px;'/></td></tr>" +
"<tr><td></td><td><input name='addr' style='width:300px;'/></td></tr>" +
"<tr><td></td><td><input name='addr' style='width:300px;'/></td></tr>" +
"<tr><td>Starter (4.99):</td>" +
"<td><input type='radio' name='starter' value='Prawn Crackers'/>Prawn Crackers" +
"<input type='radio' name='starter' value='Chicken Tikka'/>Chicken Tikka" +
"<input type='radio' name='starter' value='Spring Roll'/>Spring Roll</td></tr>" +
"<tr><td>Main (7.99):</td>" +
"<td><input type='radio' name='main' value='Prawn Curry'/>Prawn Curry" +
"<input type='radio' name='main' value='Chicken Curry'/>Chicken Curry" +
"<input type='radio' name='main' value='Shrimp Curry'/>Shrimp Curry</td></tr>" +
"<tr><td>Curry Spice:</td><td><select name='spice'><option>Mild</option>" +
"<option>Medium</option><option>Hot</option></select></td></tr>" +
"<tr><td>Promotional:</td>" +
"<td><input type='checkbox' name='promo1' value='Menu' id='menu'/>" +
"<label for='menu'>Menu</label></td></tr>" +
"<tr><td></td>" +
"<td><input type='checkbox' name='promo2' value='Discount Vouchers' id='vouch'/>" +
"<label for='vouch'>Discount Vouchers</label></td></tr>" +
"<tr><td></td>" +
"<td><input type='checkbox' name='promo3' value='Collect' id='coll'/>" +
"<label for='coll'>Collect</label></td></tr>" +
"<tr><td><input type='submit' value='Submit'></td>" +
"<td><input type='reset'/></td></tr>" +
"</table<fieldset></form></body></html>");
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
float cost = 0.0f; // Food Cost
// Create a reply to takeaway details entered
response.setContentType("text/html");
PrintWriter writer = response.getWriter();
writer.print("<html><head><title>Order Information</title></head>" +
"<body><h1>Order Information Summary</h1><form action='' method='post'>" +
"<fieldset style='background-color:silver;border:1px solid black;" +
"padding:5px;text-align:left;width:600px;'>" +
"<legend>Customer Order Summary</legend>" +
"<table><tr><td><strong>Customer Information</strong></td><td></td></tr>" +
"<tr><td>Name:</td><td>" + request.getParameter("name") + "</td></tr>" +
"<tr><td>Address:<br><br><br><br><br></td><td>");
String[] addrLines = request.getParameterValues("addr");
if (addrLines != null) {
for (String addrLine : addrLines) {
writer.println(addrLine + "<br>");
}
}
writer.println("</td></tr>");
writer.println("<tr><td><strong>Order Information</strong></td><td></td></tr>" +
"<tr><td>Starter:</td>");
if (request.getParameter("starter") == null) {
writer.print("<td>You didn't order a starter</td></tr>");
} else {
writer.print("<td>" + request.getParameter("starter") + "</td></tr>");
cost = cost + 4.99f;
}
writer.println("<tr><td>Main:</td>");
if (request.getParameter("main") == null) {
writer.print("<td>You didn't order a main</td></tr>");
} else {
writer.print("<td>" + request.getParameter("main") + "<br></td></tr>");
cost = cost + 7.99f;
}
writer.println("<tr><td>Curry Spice:</td>");
writer.print("<td>" + request.getParameter("spice") + "</td></tr>");
writer.println("<tr><td>Bill:</td>");
if (cost == 0) {
writer.print("<td>You didn't order anything</td></tr>");
} else {
writer.print("<td>Your bill comes to: " + cost + "</td></tr>");
}
writer.println("<tr><td></td><td></td></tr>");
writer.print("<tr><td><strong>Promotional Information</strong></td><td></td></tr>");
if (request.getParameter("promo1") == null) {
writer.print("<td>You didn't request a menu</td></tr>");
} else {
writer.print("<td>Your menu is in the bag with your food</td></tr>");
}
if (request.getParameter("promo2") == null) {
writer.print("<td>You didn't request any discount vouchers</td></tr>");
} else {
writer.print("<td>Your discount vouchers are in the bag with your food</td></tr>");
}
if (request.getParameter("promo3") == null) {
writer.print("<td>If you ordered food, delivery in next 30 minutes</td></tr>");
} else {
writer.print("<td>You will get 2.00 off your bill when you collect your food</td></tr>");
}
writer.print("</table<fieldset></form></body></html>");
}
}
As you can see from the code writing even basic servlets requires a lot of writer.print()
statements to enclose our HTML which can be a very laborious process. How we can write code like this much more elegantly is explained in the JSP section of the site. JSP pages are not a replacement for servlets however and they can be thought of more as complementing the processing we do in servlets.
Compiling FoodOrderServlet
Top
Open your command line editor:
Change to directory c:\_ServletsInt\foodorder\src\controller
Compile FoodOrderServlet.java
using the java compiler with the -cp
and -d
options as below, making sure you change apache-tomcat-6.0.37
to wherever you downloaded Tomcat to.
javac -cp c:\apache-tomcat-6.0.37\lib\servlet-api.jar -d ..\..\classes FoodOrderServlet.java
The following screenshot shows that we get a clean compile and also the FoodOrderServlet
class now compiled into the classes\controller
directory.
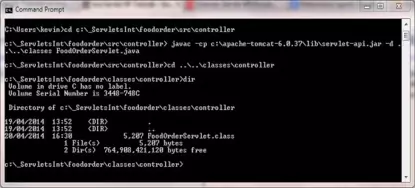
Coding The DDTop
There are no DD entries for this application that we haven't seen before.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee" version="2.5">
<servlet>
<servlet-name>FoodOrder</servlet-name>
<servlet-class>controller.FoodOrderServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>FoodOrder</servlet-name>
<url-pattern>/takeaway</url-pattern>
</servlet-mapping>
</web-app>
Save the web.xml
file in the DD
folder.
Tomcat DeploymentTop
Go to your Tomcat installation which in my case is:
C:\apache-tomcat-6.0.37\webapps\
Within the webapps
folder create a folder for our web application called foodorder
Within the foodorder
folder create the WEB-INF
folder.
Copy the web.xml
file and the classes
directory and contents from our development environment into the WEB-INF
folder.
After creating these folders and copying the files from development your Tomcat directory structure within the webapps\foodorder
folder should look something like the following screenshot:
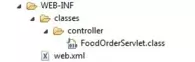
Testing Our ServletTop
Open a command prompt and change the directory to the location of our Tomcat bin directory and then type in startup
(Windows 7) or startup.sh
(Linux/Unix/OSX).
Press Enter and the Tomcat server should open up in a new window.
You can also start up Tomcat by double-clicking the startup
batch file within your Tomcat /bin
folder.
With Tomcat up and running type the following into your web browser address bar and press enter:
http://localhost:8080/foodorder/takeaway
The web browser should be directed to the FoodOrderServlet
servlet class within Tomcat, execute and produce a screen that looks like the following:
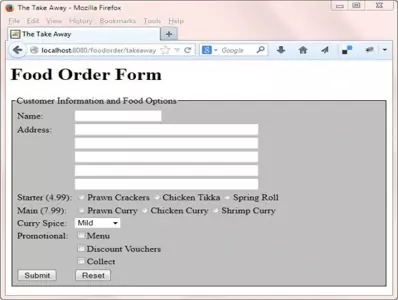
As you can see the response from the doGet()
method has returned a form for a user to fill in.
The following screenshot shows information entered into various fields on the form:
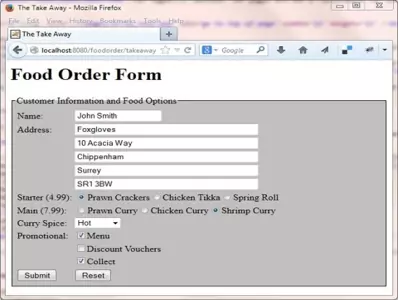
This last screenshot shows the response from the doPost()
method to the information we entered above after pressing the Submit
button:
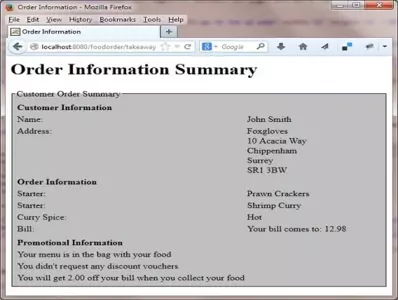
Lesson 1 Complete
In this lesson we learnt how to create HTML forms, how to extract information from them and the rules about what gets passed from the form.
What's Next?
In the next lesson we start the first of three tutorials on session management.