Attributes & ScopeS2C Home « Attributes & Scope
In the Request & Response - One Thread Per Request section we looked at how the container creates a new java thread every time a servlet request is received. What this means is that any attributes we create will exist for the duration of the request in question and so request attribute scope is for a single request/response.
In the last lesson we looked at using a HttpSession
as a session management technique and how we can attach and retrieve attributes from a HttpSession
using methods within the
HttpSession
interface. The attributes exist as long as the HttpSession
is active and so we can think of these attributes as having session scope and living through multiple requests/responses.
In the ServletConfig & ServletContext - The ServletContext Object section we looked at the ServletContext
object and how initialisation
parameters of this type are application wide. Context attributes also have application scope and can live through multiple requests/responses as well as mutiple sessions. Context attributes remain
until removed, or the application is redeployed or the server is shutdown.
So we have three scopes for our servlets these being from narrowest to broadest, request, session and context; there is a fourth scope which applies to a page and we will discuss that when we look at JSPs and the pageContext Implicit Object. Lets look at each scope in much greater detail so we can be sure about the lifetime of each scope and the attributes within it.
Request ScopeTop
The request scope and the attributes within it are available after a servlet enters its service()
method and remain in scope until the servlet exits its service()
method. For
a simple servlet the request scope can be very short but generally we override one of the doXXX()
methods and control is passed to it. The doXXX()
method can in turn call
other methods, but of course the service()
method is still on the top of the stack and so the request scope is still alive. Eventually other methods are popped off the stack and we return
to the service()
method which will exit and get popped off the stack. It is at this point that our request scope and any attributes within it become unobtainable and go out of scope.
If there are any attributes that you don't want to lose that are within request scope then you will need to move these to a broader scope, such as session, before exiting the service()
method.
This doesn't mean you can save a reference to the request scope, within session scope, and then get back your request object on the next entry to the service()
method though. The
container will generally recycle request objects because it's more efficient than garbage collecting them, so as soon as your service()
method ends, the request object will be put back in a
pool for reuse by other requests, which may or may not be from you.
So what constitutes request scope in terms of objects? Well request scope is defined by an instance of a request object which is generally derived from the HttpServletRequest
type as we are
using the HTTP protocol. Of course as we saw in earlier lessons you can override GenericServlet
if you are using another protocol, in which case the request object will be derived from the ServletRequest
type.
The following slideshow shows the request object being created and the request scope. Press the button below to step through the slideshow:
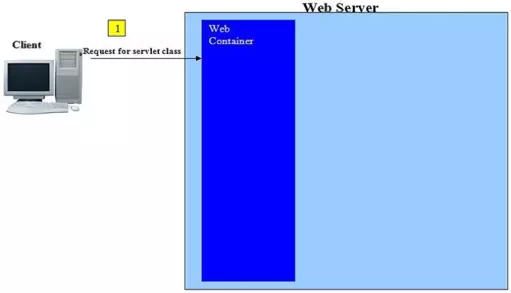
Our client has clicked on a link that points to a resource that is a servlet rather than a static HTML page, so the web server directs the HTTP request to our web container.
Session ScopeTop
Whenever a user visits a web application for the first time, if we require sessions, then the container uses the HttpSession
interface to create a session object to use between an HTTP client
and an HTTP server. This is achieved using the overloaded getSession()
method of the HttpSession
interface in one of the following ways:
HttpSession session = HttpServletRequest.getSession();
HttpSession session = HttpServletRequest.getSession(true);
The two methods above do exactly the same thing, create a session object if none exists, or return an existing session object, so the shorthand version is generally used.
Using the false
value with the getSession(boolean)
overload, which will return an existing session object, but only if one exists already:
HttpSession session = HttpServletRequest.getSession(false);
Notice how we are using the HttpServletRequest
interface to get our session. There is no way to get a session object using the ServletRequest
interface, because as far as the
EE5 Servlets API is concerned sessions belong in the realm of HTTP only. If for any reason you need sessions using another protocol then you will have to write the interfaces to achieve this yourself.
So after a servlet enters its service()
method and the request object is created we generally override one of the doXXX()
methods and control is passed to it. It's within
the doXXX()
method that we create or return our session object using the getSession()
method. The container keeps track of session objects using a unique session identifier
that identifies each individual session. Therefore when the service()
method eventually gets popped off the stack the container still has a reference to the session object. On subsequent
requests from a client the getSession()
method will then retrieve the session object using the unique session identifier. It is this way we can enter into a conversational dialect with
the client using sessions.
If a web application is distributed across several JVMs then by definition, each JVM will have its own session objects as well.
Any attributes we store using the session object can be retrieved on future requests and therefore we can retain state with the client for the lifetime of the session object. Sessions can be terminated
in a variety of ways using methods within the HttpSession
interface or the DD as discussed in Session Management Part 3 - Terminating A Session.
So what constitutes session scope in terms of objects? Well session scope is defined by an instance of a session object which is derived from the HttpSession
type. We use the request object,
which is derived from the HttpServletRequest
type to create or get the session object.
The following slideshow shows the request object being created and this being used to create a session object and how we retrieve the session object on future requests. Press the button below to step through the slideshow:
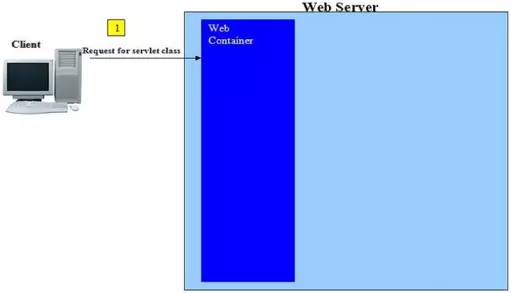
Our client has clicked on a link that points to a resource that is a servlet rather than a static HTML page, so the web server directs the HTTP request to our web container.
Context ScopeTop
Context scope, which is also known as application scope, is the broadest of the scopes available and attributes created within this scope remain from web application deployment until removed, the web application is undeployed, or the web server is shutdown.
Each web application has its own context and if the application is distributed across several JVMs then by definition, each JVM will have its own context as well.
So what constitutes context scope in terms of objects? Well context scope is defined by an instance of a context object which is derived from the ServletContext
type. We use the getServletContext()
method of the GenericServlet
type to access the context object.
The following slideshow shows the context object being created and the context scope. Press the button below to step through the slideshow:
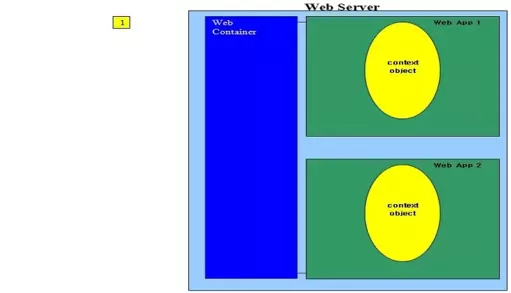
The web server is started and the web container loads up two web applications and creates a context object for each.
Attributes and ParametersTop
Before we look at the attribute methods for each scope it's worth clarifying the difference between the attributes we discuss in this lesson and the initialisation parameters we used in earlier lessons.
- Parameters are set via declarations within the DD.
Attributes are set using the appropriatesetAttribute(String name, Object value)
method. - We use
getInitParameter(String name)
method to retrieve a parameter and this returns aString
type.
We usegetAttribute(String name)
to retrieve an attribute and this returns anObject
type which will then have to be cast. - We have servlet initialisation parameters but no session parameters.
We have no servlet attributes but we do have session attributes.
Attribute OverviewTop
All the methods used to store, retrieve and remove attributes have the same names regardless of scope. The only difference in the methods, is that those concerned with session scope can all throw a
java.lang.IllegalStateException
exception if called on an invalidated session. The table below shows these methods, their respective scopes and the interfaces the methods reside in:
Method | Request Scope | Session Scope | Context Scope |
---|---|---|---|
Interface | ServletRequest | HttpSession | Context |
Object getAttribute( String name) | Returns value of specified name attribute, or null if no attribute with specified name found. |
Returns value of bound object with specified name for this session, or null if bound object not found. | Returns value of specified name attribute, or null if no attribute with specified name found. |
Enumeration getAttribute | Returns an Enumeration containing names of all the attributes available to this request, or an empty Enumeration if request has none. |
Returns an Enumeration containing attribute names of all objects bound to this session, or an empty Enumeration if session has none. | Returns an Enumeration containing all attribute names available within this servlet context. |
removeAttribute( String name) | Remove attribute with specified name from this request. | Removes bound object with specified name from this session. |
Removes attribute with specified name from the servlet context. |
setAttribute | Store attribute with specified name in this request |
Binds object to this session using specified name . | Binds an object to a specified name attribute in this servlet context. |
Lesson 5 Complete
In this lesson we looked at attributes and the different scopes they can be used in.
What's Next?
In the next lesson we look at multithreading and how attributes in the different scopes are affected.