Session Management Part 2S2C Home « Session Management Part 2
In the last lesson we looked at URL rewriting and the use of hidden fields to achieve conversational state within our applications. Both of these methods of session management are mainly suitable for applications with small page volume as the work involved increases with each new page we add to an application. In our second lesson on session management we look at cookies, the first of two tracking techniques that are more flexible and work with web applications regardless of page volume.
Cookie OverviewTop
Most users are familiar with the term 'cookie', but what exactly is a cookie? A cookie is a small amount of information sent to the browser, which in Java terms is sent by a servlet as part of the response. The browser will then pass the cookie back, via the request object, for this or any other resource that reside on this server and these mechanics are repeated while the cookie is active. All browsers are expected to support twenty cookies for each server, with upto a maximum of three hundred cookies in total. Although it can vary by browser, a cookie's size can be limited to four kilobytes.
Cookies are generally stored on the client's hard drive but can also be stored in memory for the duration of the browser session. Cookies are created using key/value pairs and then embedded into the request
object as HTTP headers, thus making their transferral back to the server totally automatic and handled via the HTTP protocol. There is even a java class called Cookie
which allows us some control over the creation, lifespan and other attributes of a cookie.
The table below shows the declarations of some of the more common methods in the Cookie
class, which we cover on the site:
Method Declaration | Description |
---|---|
Getters | |
int getMaxAge() | Returns maximum age of a cookie specified in seconds. This defaults to -1 indicating the cookie will persist until the browser is shutdown. |
java.lang.String getName() | Returns the name of the cookie. |
java.lang.String getValue() | Returns the value of the cookie. |
Setters | |
void setMaxAge(int expireInNoSeconds) | Sets the maximum age of the cookie in seconds as specified in expireInNoSeconds . Setting this value to 0 tells the browser to delete the cookie. |
Use the links in the table above to go to example code of the method in question. For more information on these and the other methods in the Cookie
object follow the link to the official site at the end of the lesson.
Before we write a servlet to demonstrate cookies, let's take a look at the advantages and disadvantages of using this session management technique.
Advantages
Cookies can be used with very little work from the developer.
Cookies can be used for web applications with small, or large page volumes without any increase in workload or maintenance.
Disadvantages
The major disadvantage with using cookies is we are totally reliant on the user not disabling cookies in their browser settings.
Cookies ExampleTop
Within the _ServletsInt
folder create a new folder for the web application we will create in this lesson and call it cookies
Within the cookies
folder create separate folders to hold our DD, source files and our compiled byte code and called dd
, src
and classes
. Within the
src
folder create a subfolder called controller
.
So after creating these folders your directory structure should look something like the following screenshot:
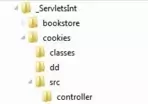
Coding OptionsServlet
Top
We are now ready to code up a servlet that uses cookies as its session management technique. On initial entry to the OptionsServlet
class below the doGet()
method will be
invoked and will display a banner and a form for user entry. After user entry and form submission the doPost()
method will be invoked and display any options the user entered within the form
whilst adding cookies for the form entries where appropriate. If the radio button entries have not been filled in by the user then we add cookies to the response for these entries with setMaxAge(0)
.
This effectively deletes these cookies as there is no delete method for removing our cookies.
Cut and paste the following code into your text editor and save it in the c:\_ServletsInt\cookies\src\controller directory as OptionsServlet.java
.
package controller;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class OptionsServlet extends HttpServlet {
private static final long serialVersionUID = 871964L;
public static final String BANNER =
"<div style='background-color:silver;border:1px solid black;padding:10px;width:340px;'>" +
"<a style='padding:25px;' href='displayOptions'>Display Options</a>" +
"<a style='padding:25px;' href='famousQuotes'>Famous Quotes </a></div>";
@Override
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// Create a form with display options
resp.setContentType("text/html");
PrintWriter writer = resp.getWriter();
writer.println("<html><head><title>Display Options</title></head><body>" +
BANNER + "<form action='' method='post'>" +
"<fieldset style='background-color:silver;border:1px solid black;" +
"padding:5px;text-align:left;width:350px;'>" +
"<legend>Settings</legend>" +
"<table><tr><td>Text Colour:</td><td>" +
"<input type='radio' name='colour' value='red'/>Red" +
"<input type='radio' name='colour' value='blue'/>Blue" +
"<input type='radio' name='colour' value='green'/>Green</td></tr>" +
"<tr><td>Background Colour:</td>" +
"<td><input type='radio' name='bc' value='silver'/>Silver" +
"<input type='radio' name='bc' value='orange'/>Orange" +
"<input type='radio' name='bc' value='yellow'/>Yellow</td></tr>" +
"<tr><td>Text Style:</td><td><select name='style'><option>bold</option>" +
"<option>italic</option><option>normal</option></select></td></tr>" +
"<tr><td><input type='submit' value='Submit'></td>" +
"<td><input type='reset'/></td></tr>" +
"</table<fieldset></form></body></html>");
}
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// Create a table of user display options
resp.setContentType("text/html");
PrintWriter writer = resp.getWriter();
writer.println("<html><head><title>Display Settings For Quotes</title></head><body>" +
BANNER + "<h2>Display Settings For Quotes</h2><table>");
if (req.getParameter("colour") != null) {
// Add cookie
String colour = req.getParameter("colour");
writer.print("<tr><td>You set text colour to: " + colour + "</td></tr>");
resp.addCookie(new Cookie("textColour", colour));
} else {
writer.print("<tr><td>You didn't set a text colour</td></tr>");
// Delete cookie if present
Cookie cookie = new Cookie("textColour", "");
cookie.setMaxAge(0);
resp.addCookie(cookie);
}
if (req.getParameter("bc") != null) {
// Add cookie
String bc = req.getParameter("bc");
writer.print("<tr><td>You set background colour to: " + bc + "</td></tr>");
resp.addCookie(new Cookie("backgroundColour", bc));
} else {
writer.print("<tr><td>You didn't set a background colour</td></tr>");
// Delete cookie if present
Cookie cookie = new Cookie("backgroundColour", "");
cookie.setMaxAge(0);
resp.addCookie(cookie);
}
String style = req.getParameter("style");
writer.print("<tr><td>Text style was set to: " + style + "</td></tr>");
resp.addCookie(new Cookie("textStyle", style));
writer.print("</table></body></html>");
}
}
Compiling OptionsServlet
Top
Open your command line editor:
Change to directory c:\_ServletsInt\cookies\src\controller
Compile OptionsServlet.java
using the java compiler with the -cp
and -d
options as below, making sure you change apache-tomcat-6.0.37
to wherever you downloaded Tomcat to.
javac -cp c:\apache-tomcat-6.0.37\lib\servlet-api.jar -d ..\..\classes OptionsServlet.java
The following screenshot shows that we get a clean compile and also the OptionsServlet
class now compiled into the classes\controller
directory.
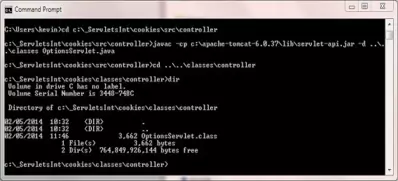
Coding The DDTop
There are no DD entries for this application that we haven't seen before.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee" version="2.5">
<servlet>
<servlet-name>display</servlet-name>
<servlet-class>controller.OptionsServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>display</servlet-name>
<url-pattern>/displayOptions</url-pattern>
</servlet-mapping>
</web-app>
Save the web.xml
file in the DD
folder.
Tomcat DeploymentTop
Go to your Tomcat installation which in my case is:
C:\apache-tomcat-6.0.37\webapps\
Within the webapps
folder create a folder for our web application called cookies
Within the cookies
folder create the WEB-INF
folder.
Copy the web.xml
file and the classes
directory and contents from our development environment into the WEB-INF
folder.
After creating these folders and copying the files from development your Tomcat directory structure within the webapps\cookies
folder should look something like the following screenshot:
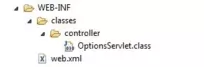
Testing Our ServletTop
Open a command prompt and change the directory to the location of our Tomcat bin directory and then type in startup
(Windows 7) or startup.sh
(Linux/Unix/OSX).
Press Enter and the Tomcat server should open up in a new window.
You can also start up Tomcat by double-clicking the startup
batch file within your Tomcat /bin
folder.
With Tomcat up and running type the following into your web browser address bar and press enter:
http://localhost:8080/cookies/displayOptions
The web browser should be directed to the OptionsServlet
servlet class within Tomcat and execute it. On initial entry to the OptionsServlet
class below the doGet()
method will be invoked and will display a banner and a form for user entry that looks like the following screenshot:
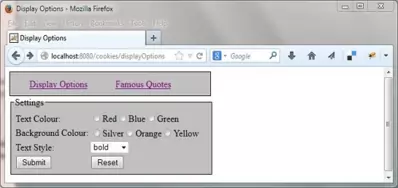
After user entry and form submission the doPost()
method will be invoked and display any options the user entered within the form
whilst adding cookies for the form entries where appropriate. The following screenshot shows the user options that were selected:
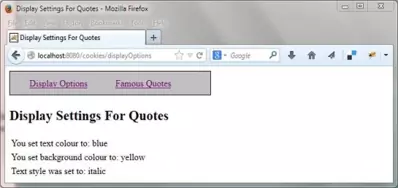
This last screenshot below was taken using the firebug console and looking at the Cookies tab. Within this tab we expanded the cookies which are all session cookies as we never added a maximum age, so all these cookies disappear when the session ends:
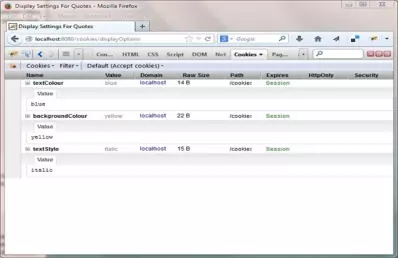
Coding QuotesServlet
Top
This next servlet will display some quotes and use any cookie values that were set within the OptionsServlet
class to format the quotes. The QuotesServlet
class below is fairly
strightforward and just uses the cookie values to style the look of the quotes.
Cut and paste the following code into your text editor and save it in the c:\_ServletsInt\cookies\src\controller directory as QuotesServlet.java
.
package controller;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class QuotesServlet extends HttpServlet {
private static final long serialVersionUID = 871964L;
private static final String BANNER =
"<div style='background-color:silver;border:1px solid black;padding:10px;width:340px;'>" +
"<a style='padding:25px;' href='displayOptions'>Display Options</a>" +
"<a style='padding:25px;' href='famousQuotes'>Famous Quotes </a></div>";
@Override
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// Create a form with display options
resp.setContentType("text/html");
PrintWriter writer = resp.getWriter();
writer.println("<html><head><title>Display Options</title></head><body>");
writer.println("<html><head><title>Famous Quotes</title></head><body>" +
OptionsServlet.BANNER + "<h2>Famous Quotes</h2>");
StringBuilder divStyle = new StringBuilder();
StringBuilder divStyle2 = new StringBuilder();
Cookie[] cookies = req.getCookies();
if (cookies != null) {
// loop through cookies for quote styling
for (Cookie cookie : cookies) {
String name = cookie.getName();
String value = cookie.getValue();
if (name.equals("backgroundColour")) {
divStyle.append("background-color:" + value + ";");
}
if (name.equals("textColour")) {
divStyle2.append("color:" + value + ";");
}
if (name.equals("textStyle")) {
if (value.equals("bold")) {
divStyle2.append("font-weight:900;");
} else {
divStyle2.append("font-style:" + value + ";");
}
}
}
}
writer.print("<div style='" + divStyle.toString() + "'><table>");
writer.print("<tr><td style='" + divStyle2.toString() +
"'>If music be the food of love, play on!</td></tr>");
writer.print("<tr><td style='" + divStyle2.toString() +
"'>A horse, a horse, my kingdom for a horse!</td></tr>");
writer.print("<tr><td style='" + divStyle2.toString() +
"'>To be or not to be, that is the question.</td></tr>");
}
}
Compiling QuotesServlet
Top
Open your command line editor:
Change to directory c:\_ServletsInt\cookies\src\controller
Compile QuotesServlet.java
using the java compiler with the -cp
and -d
options as below, making sure you change apache-tomcat-6.0.37
to wherever you downloaded Tomcat to.
javac -cp c:\apache-tomcat-6.0.37\lib\servlet-api.jar;..\..\classes -d ..\..\classes QuotesServlet.java
The following screenshot shows that we get a clean compile and also the QuotesServlet
class now compiled into the classes\controller
directory.
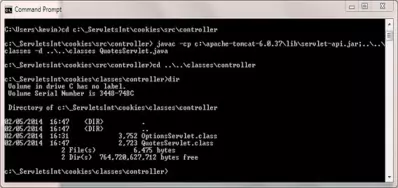
Updating The DDTop
Add the DD entries below to the web.xml
file for this lesson.
<servlet>
<servlet-name>quotes</servlet-name>
<servlet-class>controller.QuotesServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>quotes</servlet-name>
<url-pattern>/famousQuotes</url-pattern>
</servlet-mapping>
Save the web.xml
file in the DD
folder.
Tomcat DeploymentTop
Go to the web application for this lesson which in my case is:
C:\apache-tomcat-6.0.37\webapps\cookies\
Copy the web.xml
file and the classes
directories and contents from our development environment into the WEB-INF
folder.
After creating these folders and copying the files from development your Tomcat directory structure within the webapps\cookies
folder should look something like the following screenshot:
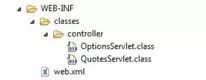
Testing Our ServletTop
Open a command prompt and change the directory to the location of our Tomcat bin directory and then type in startup
(Windows 7) or startup.sh
(Linux/Unix/OSX).
Press Enter and the Tomcat server should open up in a new window.
You can also start up Tomcat by double-clicking the startup
batch file within your Tomcat /bin
folder.
With Tomcat up and running type the following into your web browser address bar and press enter:
http://localhost:8080/cookies/famousQuotes
The web browser should be directed to the QuotesServlet
servlet class within Tomcat and execute it. In my case the options I set earlier were used and a screen that looks like the following was produced:
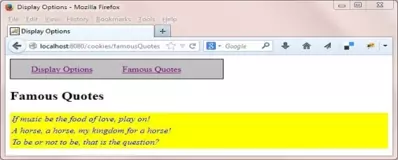
As we are not setting a duration for the cookies they disappear and need to be reentered for the quotes to change apart from the textStyle
cookie which will always get recreated on reentry
to the Display Options
screen. This is because the select
type always passes across a value unlike the radio
button type.
Java DocumentationTop
The following link will take you to the online version of documentation for the Servlet API Documentation .
Take a look at the documentation for the Cookie
object we have discussed, which you can find by clicking on the javax.servlet.http
package in the top left pane and then
clicking on the Cookie
object in the lower left pane.
Lesson 3 Complete
In this lesson we looked at the use of cookies as a session management technique.
What's Next?
In the next lesson we look at session management using the HttpSession
object.