ServletConfig & ServletContextS2C Home « ServletConfig & ServletContext
In this lesson we take a much closer look at the ServletConfig
and ServletContext
objects that every servlet gets access to after initialisation. Both of these objects allow us to access
initialisation parameters using methods within the javax.servlet.GenericServlet
abstract class.
We mentioned in the Servlet Overview lesson that the javax.servlet.GenericServlet
abstract class defines a generic, protocol-independent servlet and implements the javax.servlet.Servlet
and javax.servlet.ServletConfig
interfaces. You will probably never have a requirement to extend the javax.servlet.GenericServlet
abstract class unless you are working with a protocol other than
HTTP. It's much more likely that you will extend the abstract javax.servlet.http.HttpServlet
HTTP protocol-specific subclass. However in this lesson we will be extending the
javax.servlet.GenericServlet
abstract class in our code examples and the reason for this is two-fold:
- By extending the
javax.servlet.GenericServlet
abstract class we can focus on the methods of this class before we have to worry about protocol specific HTTP methods. - The methods within the
javax.servlet.GenericServlet
that don't override thejavax.servlet.Servlet
interface and do not pertain to logging are to do with either getting references to theServletConfig
andServletContext
objects, or retrieving values from these objects, which are the subjects of this lesson.
The ServletConfig
InterfaceTop
Before we talk about the ServletConfig
object it's worth just looking at the methods within the ServletConfig
interface. The table below shows the declarations of all the methods in the ServletConfig
interface:
Method Declaration | Description |
---|---|
java.lang.String | Returns a String containing value of specified name initialization parameter, or null if the parameter does not exist. |
java.util.Enumeration getInitParameterNames() | Returns a java.util.Enumeration of String objects containing the servlet's initialization parameters names, or an empty java.util.Enumeration if servlet has none. |
ServletContext getServletContext() | Returns a reference to the ServletContext in which the caller is executing. |
java.lang.String getServletName() | Returns name of this servlet instance. |
Use the links in the table above to go to example code of the method in question. For more information on these and the other methods in the ServletConfig
interface follow the link to the official site at the end of the lesson.
The ServletConfig
ObjectTop
We went into great detail in the The Servlet LifeCycle lesson about the various stages in the life of a servlet. If you remember we cannot do any initialisation of a servlet until
the no-args constructor has completed and the container turns our normal object into a servlet object. Any attempts to initialise the servlet using other parts of the application will fail and is the reason why we
have separate instantiation and initialisation steps. Once instantiation has completed we have a fully fledged servlet object waiting to be initialized to its default state which means that our servlet gets access to
two other objects, these being the ServletConfig
object which we will talk about now and the ServletContext
object which we will talk about later in the lesson
Each servlet gets one ServletConfig
object and this is where we can put configuration information for deployment rather than in a constructor. We configure initialisation parameters for the ServletConfig
object via the DD. This is achieved using the <init-param> sub-level element of the top-level
<servlet> element, examples of which we will look at when we come to code the DD for this lessons servlets.
Extending GenericServlet
Example 1Top
Extending the GenericServlet
class has several advantages over implementing the Servlet
interface directly. Firstly you have to override all the Servlet
interface methods when
you directly implement this interface, regardless of whether you are using them within your code, whereas the GenericServlet
class provides concrete implementations for all Servlet
methods.
If we are implementing the Servlet
interface directly and want to access the ServletConfig
object from within a servlet, then we have to override the init(ServletConfig config)
method and create an instance variable to store the ServletConfig
object. When we extend the GenericServlet
class, the init(ServletConfig config)
method is run automatically and
stores a local copy of the ServletConfig
object which can be retrieved later using the getServletConfig()
method, so we don't have to create one ourselves. After running, the init(ServletConfig config)
method delegates any further processing to the no-args init()
method. So override the convenience no-args init()
method, rather than the init(ServletConfig config)
method, if you have initailisation processing to do.
The GenericServlet
class also provides several methods that allow us to access application and servlet parameters coded declaratively within the DD which we can use instead of having to hard code values into our servlets.
Coding The DDTop
We spoke about the development and deployment environments in the Our First Servlet lesson so we won't go over the details again. Within the _ServletsJSP
folder create a
new folder for the web application we will create in this lesson and call it parms
.
Within the parms
folder create separate folders to hold our DD, source files and our compiled byte code and called dd
, src
and classes
. Within the src
folder create a subfolder called controller
.
So after creating these folders your directory structure should look something like the following screenshot:
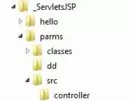
With this DD we introduce the <init-param> sub-level element of the <servlet> top-level element.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee" version="2.5">
<servlet>
<servlet-name>GenServlet1</servlet-name>
<servlet-class>controller.GenericServletDemo1Servlet</servlet-class>
<init-param>
<param-name>email</param-name>
<param-value>alf@jsptutor.com</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>GenServlet1</servlet-name>
<url-pattern>/gen1</url-pattern>
</servlet-mapping>
</web-app>
Save the web.xml
file in the DD
folder.
We have already seen and explained the top-level <servlet> element in the Our First Servlet lesson, what we haven't encountered before is its <init-param> sub-level element.
The <init-param> sub-level element is optional and can appear many times within the top-level
<servlet> element. Within <init-param>
the <description> sub-level element is optional and the <param-name>
and <param-value> sub-level elements are both mandatory, must appear only once and represent a key/value pair. The values
within these elements can be retrieved using methods of the ServletConfig
interface which are implemented within the GenericServlet
class. Examples of using these methods are shown in the
servlet coding examples within this lesson.
Coding The ServletTop
We are now ready to code up a servlet that extends the javax.servlet.GenericServlet
class, so cut and paste the following code into your text editor and save it in the c:\_ServletsJSP\parms\src\controller directory.
package controller;
// I/O Imports
import java.io.IOException;
import java.io.PrintWriter;
// Servlet Imports
import javax.servlet.GenericServlet;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
public class GenericServletDemo1Servlet extends GenericServlet {
private static final long serialVersionUID = 871964L;
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// Get init parameters
ServletConfig servletConfig = getServletConfig();
String email = servletConfig.getInitParameter("email");
ServletContext servletContext = getServletContext();
String applspec = servletContext.getInitParameter("applspec");
// Create response
response.setContentType("text/html");
PrintWriter writer = response.getWriter();
writer.print("<html><head></head><body>" +
"ServletConfig parameters: " + "<br>Email: " + email +
"<br>ServletContext parameters: " + "<br>Application Spec: " + applspec +
"</body></html>");
}
}
As you can see from the above code the only Servlet
interface method we are now overriding is service
although of course you could override any of the others if required. Within the service
method we try to extract initialisaton parameters from the ServletConfig
and ServletContext
objects. Notice how both these object methods have the same name getInitParameter()
and
how we try to extract the value using the key. We then out put a response using some HTML.
Compiling The ServletTop
Open your command line editor:
Change to directory c:\_ServletsJSP\parms\src\controller
Compile GenericServletDemo1Servlet.java
using the java compiler with the -cp
and -d
options as below, making sure you change apache-tomcat-6.0.37
to wherever you donwloaded Tomcat to.
javac -cp c:\apache-tomcat-6.0.37\lib\servlet-api.jar -d ..\..\classes GenericServletDemo1Servlet.java
The following screenshot shows that we get a clean compile and also the GenericServletDemo1Servlet
class now compiled into the classes\controller
directory.
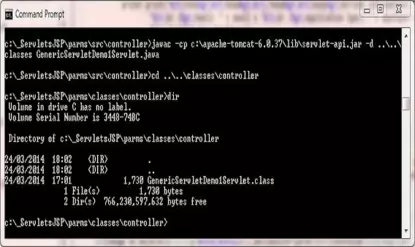
Tomcat DeploymentTop
The first thing we are going to do is go to the webapps
directory of our Tomcat installation which in my case is:
C:\apache-tomcat-6.0.37\webapps\
Within the webapps
folder create a folder for our web application called parms
Within the parms
folder create the WEB-INF
folder.
Copy the web.xml
file and the classes
directory and contents from our development environment into the WEB-INF
folder.
After creating these folders and copying the files from development your Tomcat directory structure within the webapps\parms
folder should look something like the following screenshot:
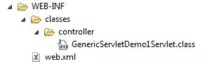
Testing Our ServletTop
Open a command prompt and change the directory to the location of our Tomcat bin directory and then type in startup
(Windows 7) or startup.sh
(Linux/Unix/OSX).
Press Enter and the Tomcat server should open up in a new window.
You can also start up Tomcat by double-clicking the startup
batch file within your Tomcat /bin
folder.
With Tomcat up and running type the following into your web browser address bar and press enter:
http://localhost:8080/parms/gen1
The web browser should have directed you to the GenericServletDemo1Servlet
servlet class within Tomcat, execute and produce a screen that looks like the following:
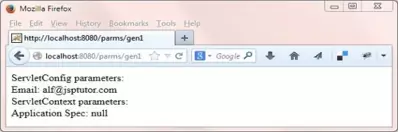
As you can see the value from the ServletConfig
initialisation parameter is displayed. The message for the ServletContext
initialisation parameter displays null
as
we haven't created this in the DD.
The ServletContext
InterfaceTop
Before we talk about the ServletContext
object it's worth just looking at some of the methods within the ServletContext
interface. The table below shows the declarations of some of the more common methods in the ServletContext
interface we use on the site:
Method Declaration | Description |
---|---|
java.lang.Object | Returns attribute with specified name , or null if no attribute with specified name found. |
java.util.Enumeration getAttributeNames() | Returns an java.util.Enumeration containing all attribute names available within this servlet context. |
java.util.Enumeration getInitParameterNames() | Returns a java.util.Enumeration of String objects containing the servlet context initialization parameters names, or an empty java.util.Enumeration if servlet context has none. |
RequestDispatcher | Returns a RequestDispatcher object that works as a wrapper for the resource located at specified path . |
void removeAttribute(java.lang.String name) | Removes the attribute with specified name from the servlet context. |
void setAttribute(java.lang.String name, | Binds an object to a specified name attribute in this servlet context. |
Use the links in the table above to go to example code of the method in question. For more information on these and the other methods in the ServletContext
interface follow the link to the official site at the end of the lesson.
The ServletContext
ObjectTop
The ServletContext
object is application wide and we only get one of these objects per application. If you have parameters that apply to the entire application then the ServletContext
object is the place to store them. This is achieved via the DD using the <context-param> top-level
element in conjunction with its <param-name> and <param-value>
sub-level elements. We will talk about these a bit later when we come to update the DD.
Extending GenericServlet
Example 2Top
We saw in the first example of extending GenericServlet
how to use the getInitParameter()
method of the ServletConfig
and ServletContext
objects, even
though we hadn't set up any parameters for the latter. In both cases we tried to obtain the value using a key, but what if we had multiple initialisation parameters for either of these objects? Luckily
for us both objects also have a getInitParameterNames()
method which returns an enumeration so we can cycle through all the parameters with relative ease. In this example we will code multiple
parameters in the DD for both the ServletConfig
and ServletContext
objects and obtain them within our servlet using the getInitParameterNames()
method.
Updating The DDTop
We will be updating the DD we created earlier in this lesson and introducing a new DD element, the <context-param> top-level element.
<?xml version="1.0" encoding="UTF-8"?>
<web-app xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee" version="2.5">
<context-param>
<param-name>applspec</param-name>
<param-value>Servlets</param-value>
</context-param>
<context-param>
<param-name>container</param-name>
<param-value>Tomcat</param-value>
</context-param>
<servlet>
<servlet-name>GenServlet1</servlet-name>
<servlet-class>controller.GenericServletDemo1Servlet</servlet-class>
<init-param>
<param-name>email</param-name>
<param-value>alf@jsptutor.com</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>GenServlet1</servlet-name>
<url-pattern>/gen1</url-pattern>
</servlet-mapping>
<servlet>
<servlet-name>GenServlet2</servlet-name>
<servlet-class>controller.GenericServletDemo2Servlet</servlet-class>
<init-param>
<param-name>Tutorials</param-name>
<param-value>Servlets</param-value>
</init-param>
<init-param>
<param-name>Section</param-name>
<param-value>2.5 Basics</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>GenServlet2</servlet-name>
<url-pattern>/gen2</url-pattern>
</servlet-mapping>
</web-app>
Save the web.xml
file in the DD
folder.
The <context-param>
ElementTop
The top-level <context-param> element is optional, contains the declaration of a web application's servlet context initialization parameters and can appear in the DD mutiple times.
Within <context-param> the <description>
sub-level element is optional and can appear many times and the <param-name>
and <param-value> sub-level elements are both mandatory, must appear only once and represent a
key/value pair. The values within these elements can be retrieved using methods of the ServletContext
interface which are implemented within the GenericServlet
class. Examples
of using these methods are shown in the servlet coding examples within this lesson.
Coding The ServletTop
In the following servlet example we once again extend the javax.servlet.GenericServlet
class. Cut and paste the following code into your text editor and save it in the c:\_ServletsJSP\parms\src\controller directory.
package controller;
// Collection Imports
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
// I/O Imports
import java.io.IOException;
import java.io.PrintWriter;
// Servlet Imports
import javax.servlet.GenericServlet;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
public class GenericServletDemo2Servlet extends GenericServlet {
private static final long serialVersionUID = 871964L;
private Map<String, String> initParamsConfigMap = new HashMap<String, String>();
private Map<String, String> initParamsContextMap = new HashMap<String, String>();
@Override
public void init() throws ServletException {
// Get ServletConfig init parameters
Enumeration<String> initParams = getServletConfig().getInitParameterNames();
while (initParams.hasMoreElements()) {
String initParamName = initParams.nextElement();
String initParamValue = getServletConfig().getInitParameter(initParamName);
initParamsConfigMap.put(initParamName, initParamValue);
}
// Get servletContext init parameters
Enumeration<String> initParams2 = getServletContext().getInitParameterNames();
while (initParams2.hasMoreElements()) {
String initParamName = initParams2.nextElement();
String initParamValue = getServletContext().getInitParameter(initParamName);
initParamsContextMap.put(initParamName, initParamValue);
}
}
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// Create response
String s ="";
Iterator<Map.Entry<String, String>> iter = initParamsConfigMap.entrySet().iterator();
while (iter.hasNext()) {
Map.Entry<String, String> entry = iter.next();
String key = entry.getKey();
String value = entry.getValue();
s = s + key + ": " + value + "<br>";
}
String t ="";
Iterator<Map.Entry<String, String>> iter2 = initParamsContextMap.entrySet().iterator();
while (iter2.hasNext()) {
Map.Entry<String, String> entry = iter2.next();
String key = entry.getKey();
String value = entry.getValue();
t = t + key + ": " + value + "<br>";
}
response.setContentType("text/html");
PrintWriter writer = response.getWriter();
writer.print("<html><head></head><body>" +
"ServletConfig parameters: " + "<br>" + s +
"<br>ServletContext parameters: " + "<br>" + t +
"</body></html>");
}
}
In this servlet example we are overriding the init()
and service()
lifecycle methods. Within the init()
method we enumerate over the getServletConfig
and getServletContext
objects using the getInitParameterNames()
method and store the results in some HashMap
objects. Within the service()
lifecycle
method we create a response and write out our stored getServletConfig
and getServletContext
object initialisation parameters from the HashMap
objects.
Compiling The ServletTop
Open your command line editor:
Change to directory c:\_ServletsJSP\parms\src\controller
Compile GenericServletDemo2Servlet.java
using the java compiler with the -cp
and -d
options as below, making sure you change apache-tomcat-6.0.37
to wherever you downloaded Tomcat to.
javac -cp c:\apache-tomcat-6.0.37\lib\servlet-api.jar -d ..\..\classes GenericServletDemo2Servlet.java
Tomcat DeploymentTop
Go to the webapps\parms\WEB-INF
directory of our Tomcat installation which in my case is:
C:\apache-tomcat-6.0.37\webapps\parms\WEB-INF
Copy the web.xml
file and the classes
directory and contents from our development environment into the WEB-INF
folder.
After creating these folders and copying the files from development your Tomcat directory structure within the webapps\parms
folder should look something like the following screenshot:
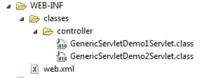
Testing Our ServletTop
Open a command prompt and change the directory to the location of our Tomcat bin directory and then type in startup
(Windows 7) or startup.sh
(Linux/Unix/OSX).
Press Enter and the Tomcat server should open up in a new window.
You can also start up Tomcat by double-clicking the startup
batch file within your Tomcat /bin
folder.
With Tomcat up and running type the following into your web browser address bar and press enter:
http://localhost:8080/parms/gen2
The web browser should have directed you to the GenericServletDemo2Servlet
servlet class within Tomcat, execute and produce a screen that looks like the following:
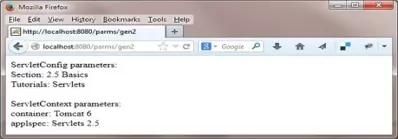
As you can see the values from the ServletConfig
and ServletContext
initialisation parameters are displayed. In fact if you run the first servlet we wrote in this lesson again,
the GenericServletDemo1Servlet
servlet class, you will see that the null
value for the ServletContext
initialisation parameter has been replaced as in the screenshot below.
With Tomcat up and running type the following into your web browser address bar and press enter:
http://localhost:8080/parms/gen1
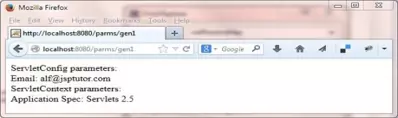
Java DocumentationTop
The following link will take you to the online version of documentation for the Servlet API Documentation .
Take a look at the documentation for the HttpSession
interface we have discussed, which you can find by clicking on the javax.servlet
package in the top left pane and then
clicking on the ServletConfig
or ServletContext
interfaces in the lower left pane.
Lesson 7 Complete
In this lesson we looked at the ServletConfig
and ServletContext
objects that every servlet gets access to.
What's Next?
Now we have the basic environment set up and before we start working with servlets we need to have an understanding of the HTTP protocol and how information is passed to and from a web server. In this lesson we take a look at HTTP, the methods used and other useful information.