JSP Implicit ObjectsS2C Home « JSP Implicit Objects
We know from studying the The Servlet LifeCycle lesson that all servlets have access to ServletConfig & ServletContext objects and we have learnt about a JSP being translated into a servlet which means that JSP servlets also have access to these objects. We then examined the container generated _jspService() lifecycle method which gives us access to the HttpServletRequest & HttpServletRequest objects. We can also access session scoped attributes after creating a HttpSession object.
When creating JSP pages we have access to all the above objects and several more, all of which are known collectivelty as implicit objects. The table below shows all the implicit objects available to JSP authors and links to the lessons in which the various types involved are discussed:
Implicit Object | Type | Lesson |
---|---|---|
All JSP Pages | ||
application | javax.servlet. | Servlets Basics - ServletConfig & ServletContext |
config | javax.servlet. | Servlets Basics - ServletConfig & ServletContext |
out | javax.servlet.jsp. | This lesson |
page | java.lang.Object | Java - OO Concepts - The Object Superclass |
pageContext | javax.servlet.jsp. | This lesson |
request | javax.servlet.http. | Servlets Basics - Request & Response |
response | javax.servlet.http. | Servlets Basics - Request & Response |
session | javax.servlet.http. | Servlets Intermediate - Session Management Part 3 |
Error JSP Pages Only | ||
exception | java.lang. | This lesson |
Apart from the exception
which is only available in JSP error pages all the other implicit objects are always available for use within scriptlets and scriptlet expressions through scripting variables that
are declared implicitly at the beginning of the JSP page. We have access to these objects via boilerplate code that gets generated at translation time when our JSP page gets translated into Servlet implementation
code. The following code snippet highlights the implicit objects being created and initialized at the start of the _jspService() method and is taken from translation of the first.jsp
JSP page and
is done using the Tomcat container and will differ for other containers. The translated code for Tomcat can be found within a subdirectory of the work
directory within your Tomcat installation. On my machine the full path is:
C:\apache-tomcat-6.0.37\work\Catalina\localhost\firstjsp\org\apache\jsp\
...
public void _jspService(HttpServletRequest request, HttpServletResponse response)
throws java.io.IOException, ServletException {
PageContext pageContext = null;
HttpSession session = null;
ServletContext application = null;
ServletConfig config = null;
JspWriter out = null;
Object page = this;
JspWriter _jspx_out = null;
PageContext _jspx_page_context = null;
try {
response.setContentType("text/html");
pageContext = _jspxFactory.getPageContext(this, request, response,
null, true, 8192, true);
_jspx_page_context = pageContext;
application = pageContext.getServletContext();
config = pageContext.getServletConfig();
session = pageContext.getSession();
out = pageContext.getOut();
...
application
Implicit ObjectTop
The application
implicit object is of type javax.servlet.servletContext
and saves you the trouble of creating your own application scoped variable. You can use the application
implicit object anywhere you would use the ServletContext
object in a regular Servlet such as setting application wide atributes.
The following code snippet shows some application
implicit object use:
<%-- Using application Implicit Object --%>
<% application.setAttribute("name", "joe"); %>
<%= application.getAttribute("name") %>
For more information on the javax.servlet.servletContext
interface and the methods available take a look at the ServletContext
Interface section.
config
Implicit ObjectTop
The config
implicit object is of type javax.servlet.servletConfig
and can be used to access ServletConfig
methods for such things as getting initialisation parameters.
The following code snippet shows some config
implicit object use:
<%-- Using config Implicit Object --%>
<%= config.getInitParameter("email") %>
For more information on the javax.servlet.ServletConfig
interface and the methods available take a look at the ServletConfig
Interface section.
out
Implicit ObjectTop
The out
implicit object is of type javax.servlet.jsp.JspWriter
and not java.io.PrintWriter
as you might expect, although the javax.servlet.jsp.JspWriter
class does
emulate many of the features of the java.io.PrintWriter
class. The main reason that the out
implicit object uses javax.servlet.jsp.JspWriter
is for that classes buffering capabilities
which are functionally similar to those of the java.io.BufferedWriter
class.
The following code snippet shows some out
implicit object use:
<%-- Using application Implicit Object --%>
<%! String strArray[] = {"one", "aa", "c", "rt", "je"}; %>
<% out.print("Value of position 5 (index 4) : " + strArray[4]); %>
page
Implicit ObjectTop
The page
implicit object is of type java.lang.Object
and is a reference to the JSP page itself and so is not generally used by us programmers to get to any methods.
For more information on java.lang.Object
go to the Java - OO Concepts - The Object
Superclass.
pageContext
Implicit ObjectTop
The pageContext
implicit object is of type javax.servlet.jsp.PageContext
and is the container's implementation of the JSP page in question. The pageContext
implicit object
allows us to access all the regular Servlet objects via the getServletConfig
, getServletContext
, getServletRequest
and getServletResponse
methods. Although these objects
can be more readily accessed via their implicit objects, these methods allow Servlet objects to be accessed using the pageContext
in tandem with the Expression Language as we will discover in the Expression Language 2.1 section.
The pageContext
implicit object also allows access to a new scope that comes with JSP pages and which is known unsuprisingly as page scope. Page scope is the narrowest of the four scopes available and
attributes stored within this scope are only available within the same JSP page itself. For a refresher on using the request, session and context scopes see the Servlets - Attributes and Scope lesson.
The value of scope
held within the PageContext
type can be one of the following constants: PAGE_SCOPE
, REQUEST_SCOPE
, SESSION_SCOPE
or APPLICATION_SCOPE
.
You can also work with attributes from page scope, or one of the other scopes, using one of the overloaded attribute methods. The following code snippet shows signatures for the overloaded getAttribute()
method:
// Page scope attribute
public abstract Object getAttribute(String name)
// Named scope attribute
public abstract Object getAttribute(String name, int scope)
If no scope is specified then attributes from the page scope will be actioned. The following code snippet shows some pageContext
implicit object use:
<%-- Using pageContext Implicit Object --%>
<%
pageContext.setAttribute("name", "charlie", PageContext.APPLICATION_SCOPE);
pageContext.setAttribute("name", "marlie");
out.print("Application attr: " + pageContext.getAttribute("name", PageContext.APPLICATION_SCOPE));
out.print(" | Page attr: " + pageContext.getAttribute("name"));
%>
request
and response
JSP Implicit ObjectsTop
The request
and response
implicit objects are actually passed via parameters to the _jspService() method and are of types
javax.servlet.http.HttpServletRequest
and javax.servlet.http.HttpServletResponse
respectively in the vast majority of cases.
Of course there is nothing to stop you as a coder from creating a JSP page for a protocol other than HTTP by using the javax.servlet.jsp.HttpJspPage
interface, that extends the javax.servlet.jsp.JspPage
interface, which extends the javax.servlet.Servlet
interface. So if for any reason you wanted to write non-HTTP JSP pages, you would implement the javax.servlet.jsp.JspPage
interface for
the protocol required and this would still get translated into a Servlet. In this scenario your request
& response
implicit objects are still passed via parameters to _jspService(),
but would be of types javax.servlet.ServletRequest
and javax.servlet.ServletResponse
respectively.
The following code snippet shows some request
& response
implicit object use:
<%-- Using request Implicit Object --%>
<%= request.getHeader("user-agent") %>
<%-- Using response Implicit Object --%>
<%= response.containsHeader("content-type") %>
For more information on the javax.servlet.http.HttpServletRequest
interface and the methods available take a look at the ServletRequest
Overview
and HttpServletRequest
Overview sections.
For more information on the javax.servlet.http.HttpServletResponse
interface and the methods available take a look at the ServletResponse
Overview
and HttpServletResponse
Overview sections.
session
Implicit ObjectTop
The session
implicit object is of type javax.servlet.http.HttpSession
and can be used to access HttpSession
methods to do all the things you would generally do when tracking sessions. Unlike
normal Servlets where we have to explicitly create session objects for our servlets this is done implicitly and so every page always comes with a HttpSession
object. If your page doesnt require sessions
then you have to turn off session creation using a page directive.
The following code snippet shows some session
implicit object use:
<%-- Using session Implicit Object --%>
<% session.setAttribute("name", "joe"); %>
<%= session.getAttribute("name") %>
<% session.invalidate(); %>
The following code snippet shows how we stop creation of the session
implicit object for a page using the page directive. Running the code will cause an exception as the
session
implicit object is never created.
<%@ page session="false" %>
<%-- Next method will cause error as session never created --%>
<%= session.isNew() %>
For more information on the javax.servlet.http.HttpSession
interface and the methods available take a look at the HttpSession
Interface section.
exception
Implicit ObjectTop
The exception
implicit object is of type java.lang.Throwable
which is the superclass of all exceptions and can be read about at the java Tutorial site at the following link
Java - Flow Control - Exception Overview. Unlike the other implicit objects, exception
is only available in pages that
are designated as error pages and have the isErrorPage
page directive set. This directive tells the translator to include the exception
implicit object in the generated servlet for the
page and is shown in the following code snippet:
<%@ page isErrorPage="true" %>
Pages wishing to use the error page do not call it directly but include the errorPage
page directive pointing to the error page required. The URL path can begin with a forward slash making it relative to
the context root of the application, or not making it relative to the JSP page. The following code snippet shows an example of using the errorPage
page directive:
<%@ page errorPage="/errorjsp/exampleErrorPage.jsp" %>
Coding An Error PageTop
To see the mechanics of how error pages work we will create a couple of JSP pages, one that will produce an error and one that will handle that error, but before we do this lets set up a folder to put the JSPs in.
Within the _JSP
folder create a folder for our web application which we will call errorjsp
Within the errorjsp
folder we will create a view
folder to hold our JSPs.
So after creating these folders your directory structure should look something like the following screenshot:
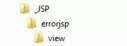
Coding bad.jsp
Top
First we will create a simple JSP page that causes an exception when it tries to access a non-existant session and has a page directive pointing to an error page. Note that we have commented out the
errorPage
page directive.
<%@ page session="false" %>
<%-- <%@ page errorPage="error.jsp" %> --%>
<%-- Next method will cause ArithmeticException: / by zero --%>
<% int a = 5/0; %>
Saving bad.jsp
Ok, with Notepad or a similar text editor cut and paste the above code.
Click file from the toolbar and then click the save option.
In the Save in: tab at the top make sure you are pointing to the C:\_JSP\errorjsp\view
folder.
In the File name: text area type bad.jsp.
Change the Save as type: option to All Files
as we don't want to end up with a .txt
extension and then click the Save button and close the Notepad.
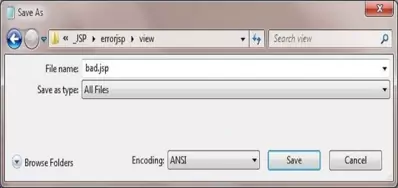
The following screenshot shows what would happen if we were to deploy and run the bad.jsp
page, not a very user-friendly experience.
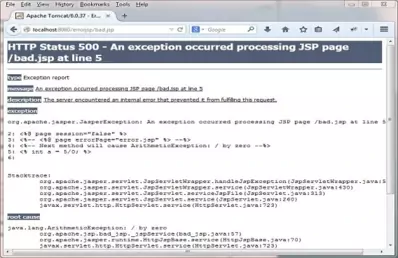
Edit the bad.jsp
code to uncomment the errorPage
page directive as shown below and save the file .
<%@ page session="false" %>
<%@ page errorPage="error.jsp" %>
<%-- Next method will cause error.jsp page to be called --%>
<% int a = 5/0; %>
Coding error.jsp
Top
Lets now code an error page that bad.jsp
will call if we get an error.
<%@ page isErrorPage="true" %>
<!DOCTYPE html>
<html>
<head><title>JSP Error Page</title></head>
<body>
<h1>An error has occured</p>
<p>The error message is: <%= exception.getMessage() %></p>
<p>Please contact support on xxx-xxx-xxxx</p>
</body>
</html>
Saving error.jsp
Ok, with Notepad or a similar text editor cut and paste the above HTML.
Click file from the toolbar and then click the save option.
In the Save in: tab at the top make sure you are pointing to the C:\_JSP\errorjsp\view
folder.
In the File name: text area type error.jsp and then click the Save button and close the Notepad.
Change the Save as type: option to All Files
as we don't want to end up with a .txt
extension and then click the Save button and close the Notepad.
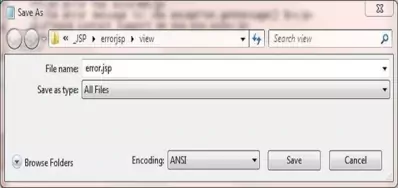
Tomcat DeploymentTop
Go to your Tomcat installation which in my case is:
C:\apache-tomcat-6.0.37\webapps\
Within the webapps
folder create a folder for our web application called errorjsp
Copy the bad.jsp
and error.jsp
files from our development environment into the errorjsp
folder.
Testing Our Error JSPTop
Open a command prompt and change the directory to the location of our Tomcat bin directory and then type in startup
(Windows 7) or startup.sh
(Linux/Unix/OSX).
Press Enter and the Tomcat server should open up in a new window.
You can also start up Tomcat by double-clicking the startup
batch file within your Tomcat /bin
folder.
With Tomcat up and running type the following into your web browser address bar and press enter:
http://localhost:8080/errorjsp/bad.jsp
The web browser should be directed to the bad.jsp
JSP within Tomcat, execute and then call the error.jsp
page producing a screen that looks like the following:
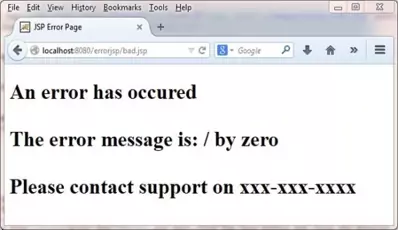
As you can see this is more user-friendly and can be tailored to fit in with the rest of the web application.
Lesson 4 Complete
In this lesson we looked at implicit objects which allow us to access the Servlet and JSP objects created by the container.
What's Next?
In the next lesson we take a much closer look at the page and include directives.