Servlet OverviewS2C Home « Servlet Overview
We mentioned in the first lesson of the section how we can think of a servlet as a Java class which we can use to extend the capabilities of an application server, and how servlets can respond to any types of request, but are commonly used to extend applications hosted by web servers using a web container such as Tomcat. We also stated that servlets give us the ability to serve dynamic web content to users from a container within our web server and are generally used with the HTTP protocol. What we didn't do was explain how all this was achieved and in this lesson we put this to rights by starting our in depth investigation of servlets by looking at the java interfaces and classes we need to create our own servlets.
Servlet HierarchyTop
Servlets are essentially Java classes, they have to be compiled into bytecode and get instantiated in exactly the same way as any other java class. What makes a servlet different from a normal Java class then I hear
you ask? Well servlets implement the javax.servlet.Servlet
interface 1 either directly, by writing a class that implements the javax.servlet.Servlet
interface,
or indirectly by extending a class that implements the javax.servlet.Servlet
interface. You will probably never need to directly implement the javax.servlet.Servlet
interface, although you
can see an example of doing this in the Our First Servlet lesson.
We will be using the javax.servlet.GenericServlet
abstract class to implement the javax.servlet.Servlet
interface which defines methods for our servlets behaviour. Once again its extremely
unlikely that you will need to extend this class, but you can see examples of doing this in the ServletConfig & ServletContext lesson.
As we are using HTTP as our protocol of choice we will take advantage of the servlet API and extend the javax.servlet.http.HttpServlet
abstract class, which extends the javax.servlet.GenericServlet
abstract class, with our own customized class for the business processes required. The diagram below illustrates the servlet class hierarchy we have just discussed:
1.As a point of interest classes that are packaged within the javax
package are java extensions, which basically means they are not part of the Standard Edition (SE). We discussed that
servlets are part of the Enterprise Edition (EE) in the Java EE5 & Servlets lesson.
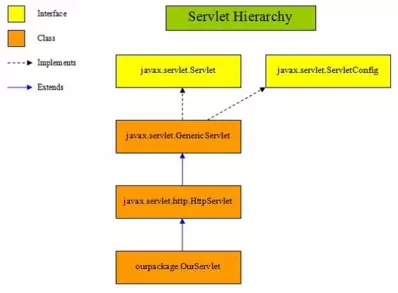
In the following sections of the lesson we look at some of the methods and discuss in a bit more detail the Servlet
interface and GenericServlet
and HttpServlet
classes shown in the diagram above.
Servlet
Methods OverviewTop
A servlet has to implement the javax.servlet.Servlet
interface to be a servlet and the interface has five methods that we need to override if we directly implement the javax.servlet.Servlet
interface. These methods are overridden for us if we extend the javax.servlet.GenericServlet
abstract class. You can see an example of doing this in the Our First Servlet lesson.
The table below shows the declarations of all the methods in the javax.servlet.Servlet
interface:
Method Declaration | Description |
---|---|
Discussed in The Servlet Lifecycle lesson | |
void init(ServletConfig config) | Invoked by the container the first time the servlet is requested. Where you write initialization code required for a servlet if you extend the Servlet class. |
void service(ServletRequest req, ServletResponse resp) | Invoked by the container each time the
servlet is requested. This is where you can write the business code for a servlet if you extend the Servlet class. |
void destroy() | Invoked by container when servlet is going to be destroyed. Where you write tidy-up code for a servlet if you extend the Servlet class. |
Discussed in Our First Servlet lesson | |
ServletConfig getServletConfig() | Returns the ServletConfig object containing initialization and startup parameters for a servlet. |
java.lang.String getServletInfo() | Returns information about a servlet. |
We will go through the three lifecycle methods in much greater detail in The Servlet Lifecycle lesson. We will briefly talk about the java.lang.String getServletInfo()
and the ServletConfig getServletConfig()
methods in the Our First Servlet lesson. Use the links in the table above to go to example code of the method in question. For more information on any of the methods listed in this lesson follow the link to the official documentation below.
Java DocumentationTop
Java comes with very rich documentation which includes extensive text devoted to servlets. The following link will take you to the online version of documentation for the Servlet API Documentation .
Take a look at the documentation for the Servlet
class which you can find by scrolling down the lower left pane and clicking on Servlet. You will go back to this documentation time and time again so
I suggest adding this link to your browser's favourites toolbar for fast access.
GenericServlet
Methods OverviewTop
The javax.servlet.GenericServlet
abstract class defines a generic, protocol-independent servlet and implements the javax.servlet.Servlet
and javax.servlet.ServletConfig
interfaces. Although we can write a servlet to extend GenericServlet
, it's much more likely we extend javax.servlet.http.HttpServlet
, the HTTP protocol-specific subclass which is what we do in the
vast majority of code examples on the site. You can see examples of extending the GenericServlet
abstract class in the ServletConfig & ServletContext lesson.
The table below shows the declarations of all the methods in the GenericServlet
class:
Method Declaration | Description |
---|---|
Discussed in The Servlet Lifecycle lesson | |
public void init(ServletConfig config) | Invoked by the container the first time the servlet is requested. Delegates writing of initialisation code to no-args init() . |
public abstract void | Invoked by the container
each time the servlet is requested. This method is declared abstract, so protocol specfic subclasses such as HttpServlet must override it. . |
public void destroy() | Invoked by container when servlet is going to be destroyed. Where you write tidy-up code for a servlet. |
Discussed in ServletConfig & ServletContext lesson | |
public void init() throws ServletException | Convenience method that can be overridden to avoid having to call super.init(config) .Where you write initialization code required for a servlet. |
public java.lang.String | Returns a String object containing value of named initialization parameter, or null if parameter does not exist. |
public java.util.Enumeration | Returns the servlet's initialization parameter names as an Enumeration of String objects, or
an empty Enumeration if there are no initialization parameters. |
public ServletConfig getServletConfig() | Returns the ServletConfig object containing initialization and startup parameters for a servlet. |
public ServletContext getServletContext() | Returns a reference to the ServletContext in which a servlet is running. |
Not discussed | |
public java.lang.String getServletInfo() | Returns information about the servlet. |
public java.lang.String getServletName() | Returns the name of this servlet instance. |
void log(java.lang.String message) | Writes specified message to a servlet log file. |
void log | Writes specified message and stack trace for a given Throwable exception to a servlet log file. |
We will go through the three lifecycle methods in much greater detail in The Servlet Lifecycle lesson and look at most of the other methods in the ServletConfig & ServletContext lesson. Use the links in the table above to go to example code of the method in question. For more information on any of the methods listed in this lesson follow the link to the official documentation above.
HttpServlet
Methods OverviewTop
Subclass the javax.servlet.http.HttpServlet
abstract class to create a HTTP servlet for a Web site. Any subclass of HttpServlet
must override at least one method. The table below shows the
declarations of all the methods in the HttpServlet
class:
Method Declaration | Description |
---|---|
Discussed in The service() Lifecycle Method | |
protected void | Called by server, via protected service() method to permit a servlet to handle a HTTP DELETE request. |
protected void | Called by server, via protected service() method to permit a servlet to handle a HTTP GET request. |
protected void | Receives a HTTP HEAD request from the protected service() method and handles the request. |
protected void | Called by server, via protected service() method to permit a servlet to handle HTTP OPTIONS request. |
protected void | Called by server, via protected service() method to permit a servlet to handle a HTTP POST request. |
protected void | Called by server, via protected service() method to permit a servlet to handle a HTTP PUT request. |
protected void | Called by server, via protected service() method to permit a servlet to handle a HTTP TRACE request. |
protected void | Receives standard HTTP requests from the public service() method and dispatches them to the doXXX() methods defined in this class. |
void service(ServletRequest req, Response resp) | Dispatches client requests to the protected service() method. |
Not discussed | |
protected long | Returns the time, in milliseconds since midnight January 1, 1970 GMT , when the HttpServletRequest object was last modified. |
All the above methods are discussed in The service() Lifecycle Method section of The Servlet Lifecycle lesson. Use the links in the table above to go to example code of the method in question. For more information on any of the methods listed in this lesson follow the link to the official documentation above.
Lesson 4 Complete
In this lesson we looked at an overview of the servlet hierarchy and the classes and methods therein.
What's Next?
In the next lesson we look at the servlet lifecycle, from the loading and instantiation of a servlet to its eventual destruction.