Advanced EnumerationsS2C Home « Advanced Enumerations
So far we have only looked at basic enumerations, now it's time to examine some more advanced features of enumerations:
package com.server2client;
/*
Enumeration of soups
*/
enum Soup {
TOMATO("vegetable"), CHICKEN("meat"), PRAWN("seafood");
String type;
/*
enum constructor
*/
Soup(String type) {
this.type = type;
}
/*
get the 'type' instance variable
*/
String getType() {
return this.type;
}
}
Here we create an enumeration using the enum
keyword but we give each of the enumeration constants TOMATO
, CHICKEN
and PRAWN
a value. This means we need a constructor to instantiate this type and we also supply a method to get the value of the type
instance variable. Let's write a new test class to test our enhanced Soup
enum.
package com.server2client;
/*
Test Class5 for Soup
*/
public class SoupTest5 {
public static void main (String[] args) {
for (Soup s : Soup.values()) {
System.out.println("We have " + s + " soup in our list, which is a "
+ s.getType() + " soup.");
}
}
}
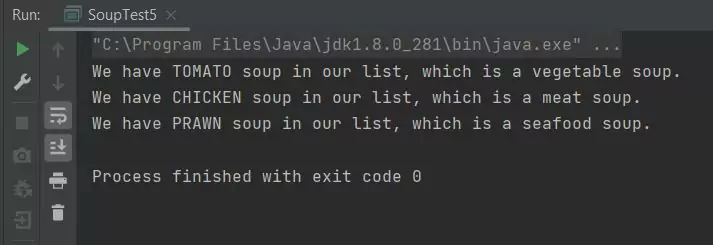
SoupTest5
class.The above screenshot shows the output of running our SoupTest5
class. We iterate through the Soup
enum constants using the predefined values()
method and an enhanced for loop.
Within the loop we extract the type
instance variable for each enum constant and print both off in a message.
Constant Specific Class Bodies Top
We can also supply constant specific class bodies for our enums which are used to override methods within the enum class. We will expand our Soup
enum to illustarte this:
package com.server2client;
/*
Enumeration of soups
*/
enum Soup {
TOMATO("vegetable") { // Constant-specific class body
public String starRating() {
return "5 star rated";
}
},
CHICKEN("meat"),
PRAWN("seafood") { // Constant-specific class body
public String starRating() {
return "3 star rated";
}
};
String type;
/*
enum constructor
*/
Soup(String type) {
this.type = type;
}
String getType() {
return this.type;
}
/*
default star rating
*/
String starRating() {
return "not rated yet";
}
}
Here we create an enumeration using the enum
keyword but we give each of the enumeration constants TOMATO
, CHICKEN
and PRAWN
a value. We also use some constant specific class bodies for our enums which we are using to override the starRating()
method within the enum class. Lets write a new test class for our expanded enum.
package com.server2client;
/*
Test Class6 for Soup
*/
public class SoupTest6 {
public static void main (String[] args) {
for (Soup s : Soup.values()) {
System.out.println("We have " + s + " soup in our list, which is a "
+ s.getType() + " soup and is " + s.starRating());
}
}
}
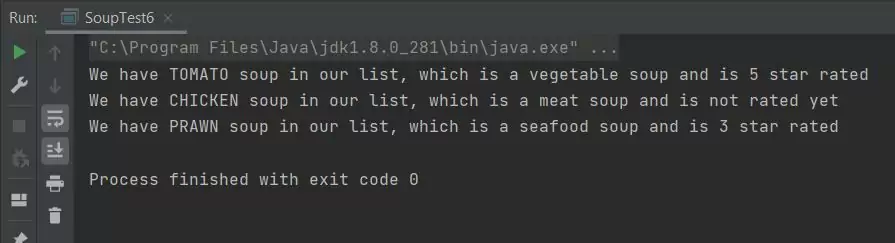
SoupTest6
class.The above screenshot shows the output of running our SoupTest6
class. We iterate through the Soup
enum constants using the predefined values()
method and an enhanced for loop. Within the loop we extract the type
instance variable for each enum constant and also get a star rating either from the constant specific class body or the default starRating()
method if no constant specific class body exists as is the case for the CHICKEN
enum constant.
Enum Singletons Top
Singletons are classes that are instantiated exactly once and with the introduction of enumerated types in Java 5 we got a new way of making a singleton. We create an enum singleton with a single enumeration constant as follows:
package com.server2client;
/*
Enumeration example of a singleton
*/
public enum ThereCanBeOnlyOne {
INSTANCE
}
Following is a test class for our enum singleton.
package com.server2client;
/*
Test Class for ThereCanBeOnlyOne
*/
public class ThereCanBeOnlyOneTest {
public static void main (String[] args) {
ThereCanBeOnlyOne me = ThereCanBeOnlyOne.INSTANCE;
System.out.println("Our instance " + me);
ThereCanBeOnlyOne me2 = ThereCanBeOnlyOne.INSTANCE;
System.out.println("Our instance " + me2);
}
}
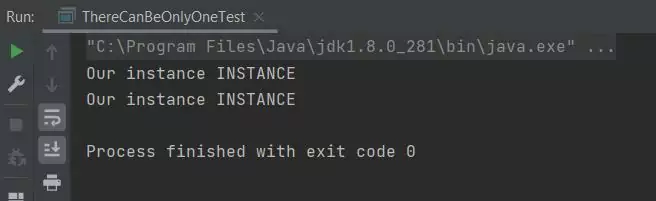
ThereCanBeOnlyOneTest
class.The above screenshot shows the output of running our ThereCanBeOnlyOneTest
class. We are not creating two instances of the ThereCanBeOnlyOneTest
class, just returning the same enum singleton each
time into named variables. This is because enumeration constants are implicitly declared as public static
members of the enumerated class, so by having just a single element enum type
we are ensuring that only one instance is ever created and therefore immutable.
Related Quiz
Objects & Classes Quiz 16 - Advanced Enumerations
Lesson 16 Complete
In this lesson we studied some advanced concepts of enumerations which were introduced in Java5.
What's Next?
That's it for the Objects & Classes section! Up until now we haven't really worried about our data and how we expose it to the outside world. That is all about to change as we look at encapsulation.