Instance Variables & ScopeS2C Home « Instance Variables & Scope
Java comes with three kinds of scope and we name variables according to the scope they reside in as detailed in the table below. In this lesson we focus on instance variable scope.
Variable | Scope | Lifetime |
---|---|---|
static | Static variables apply to the class as a whole and are declared within the class but outside a method. | Exists for as long as the class it belongs to is loaded in the JVM .See the Static Members lesson for more information. |
instance | Instance variables apply to an instance of the class and are declared within the class but outside a method. | Exists for as long as the instance of the class it belongs to. |
local | Local variables apply to the method they appear in. | Exists until the method it is declared in finishes executing. See the Method Scope lesson for more information. |
Declaring Instance Variables Top
in Java we declare variables anywhere within a block of code and instance variables are defined within a class but outside any methods. Instance variables can be declared anywhere within class scope, but by convention they are declared before any methods of the class and we will adhere to this.
Lets return to our simple Cat
class we coded earlier in the Defining A Class section of the Class Structure & Syntax lesson.
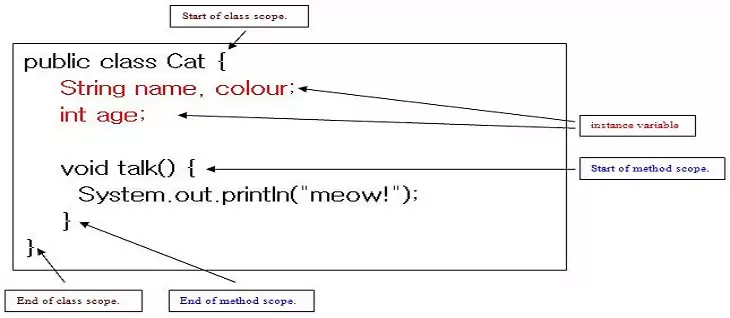
What is not apparent from the diagram is the difference between instance and local variables. Local variables always need to be initialized before use, instance variables are assigned a default value unless explicitly assigned one.
Instance variables are assigned a default value according to their type:
- object -
null
- boolean -
false
- char -
/u0000
- integer types (
byte
,short
,int
andlong
) -0
- floating-point types (
float
,double
) -0.0
To prove this lets create an instance of our Cat
class and display its instance variables:
package com.server2client;
/*
Test Class3 for Cat
*/
public class TestCat3 {
public static void main (String[] args) {
Cat moggy = new Cat(); // Create a Cat instance
System.out.println("Our " + moggy.colour + " cat called " + moggy.name + " is " + moggy.age);
}
}
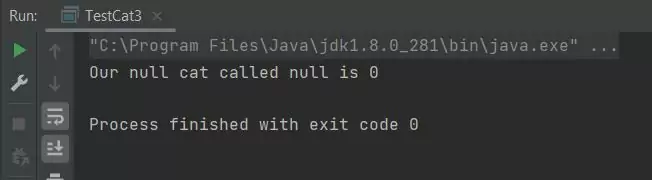
TestCat3
class.The above screenshot shows the output of running our TestCat3
class. We created a Cat
instance and displayed the values of its instance variables. The String
objects values default to null
and the int
primitive defaults to 0
.
Looking At Object State Top
As mentioned earlier in this section each time we create a new instance of the class, that instance gets its own set of instance variables. The variables are completely unique to each instance and the values held within each
instance are known as object state. Objects live on the Heap
and as instance variables represents an Object State and so they also reside on the
Heap
within the appropriate instance of their object type. Lets take a closer look at what this actually means with our simple Cat
class. Using the following slide show just press the buttons to step through it.
Lets look at some code to illustrate the above scenario:
package com.server2client;
/*
Test Class4 for Cat
*/
public class TestCat4 {
public static void main (String[] args) {
Cat moggy = new Cat();
Cat tigger = new Cat();
Cat tabby = new Cat();
moggy.name = "Henry";
moggy.colour = "black";
moggy.age = 4;
tigger.name = "Kitty";
tigger.colour = "grey";
tigger.age = 15;
tabby.name = "Felix";
tabby.colour = "white";
tabby.age = 8;
System.out.println("Our " + moggy.colour + " cat called " + moggy.name + " is " + moggy.age);
System.out.println("Our " + tigger.colour + " cat called " + tigger.name + " is " + tigger.age);
System.out.println("Our " + tabby.colour + " cat called " + tabby.name + " is " + tabby.age);
}
}
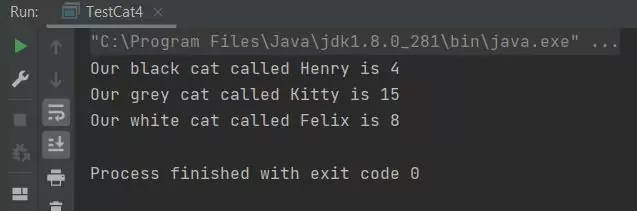
TestCat4
class.The assignments of the reference variables happen as in the slide show and we print out the instance variables for each instance.
Instance Variable Scope Top
In our discussion on the Heap
in the last lesson we mentioned that as long as we have a reference to an object then it exists on the
Heap
. Objects are eligible for removal from the Heap
by the garbage collector when their scope ends and there are no live references to them, or they become
unreachable, or the reference to the object is set to null
. So what does this mean for our instance variables? Well as the instance variables are unique to an instance of their class it means when no reference
variables point to that instance it and any instance variables attached to it go out of scope. So the lifetime of instance variables, aka their scope, are implicitly tied to the object they belong to, when the object dies so does its state.
Related Quiz
Objects & Classes Quiz 8 - Instance Variables & Scope
Lesson 8 Complete
This lesson was about instance variables, how to use them and their scope.
What's Next?
In the next lesson we learn about final instance variables.