Class Structure & SyntaxS2C Home « Class Structure & Syntax
In the last lesson on Array Examples & Exceptions we introduced some new concepts such as instantiating an instance of a class using the new
operator and using a class member via anArray.length
. We will expand on these topics
as we go through this section but firstly lets take a look at the structure and syntax of a class.
A Look At Class Structure Top
A class is made up of members that form a blueprint for an object and its behaviour. Before we go into more details lets look at a diagram showing the generic form of a class:
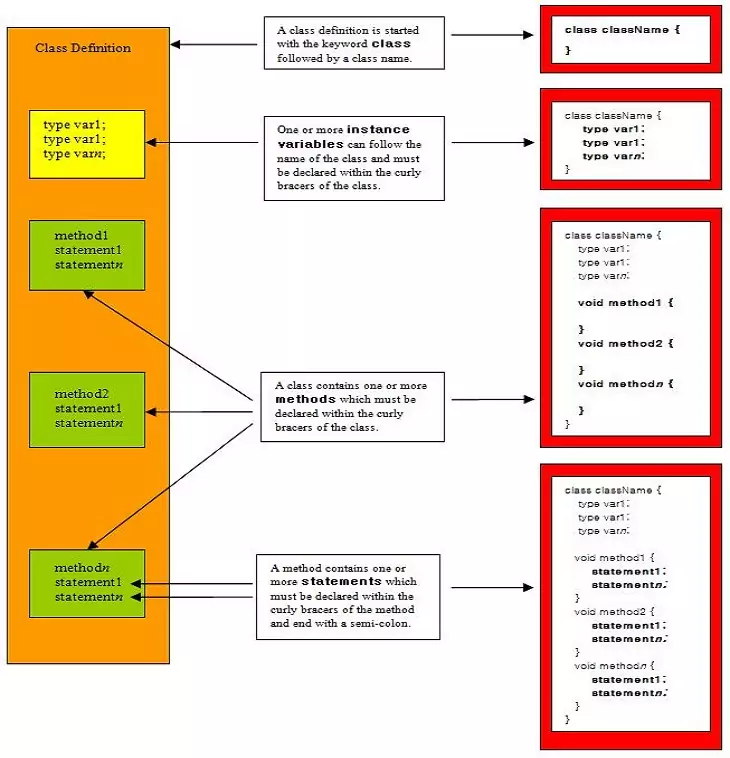
So using the diagram above we can note down a few points about a Java class:
- We define a class using the
class
keyword followed by the name of the class and a set of curly bracers. - We can specify one or more instance variables within the curly bracers of the class and each must end with a semi-colon.
- We can specify one or more methods within the curly bracers of the class, after any instance variables, with each method also having a set of curly bracers.
- The
void
keyword preceding the name of each method specifies that the method doesn't return any value. This is known as a return type and is mandatory. - We can specify one or more statements within the curly bracers of each specified method and each must end with a semi-colon.
There is more to classes than the points raised above but for now this is more than enough to be thinking about. The reason variables defined within a class, and outside any method within the class, are called instance variables is that they belong to instances of a class. If we think of an object as an instance of a class, then the instance variables belong to individual instances (objects) of the class. So each time we create a new instance (object) of the class, that instance gets its own set of instance variables. The variables are completely unique to each instance and the values held within each instance are known as object state. So getting back to our class structure we can say that a class is not an object but is used to construct an object instance.
Defining A Class Top
In the previous section we created a lot of classes that contained a main()
method to focus on programming features of Java and so the classes weren't created for a specific purpose. As we mentioned at the start
of this section a well designed class should define a logical entity that fits a particular requirement within the application as a whole. To highlight what we mean by this lets look at the syntax of a simple class that has
a particular purpose in mind.
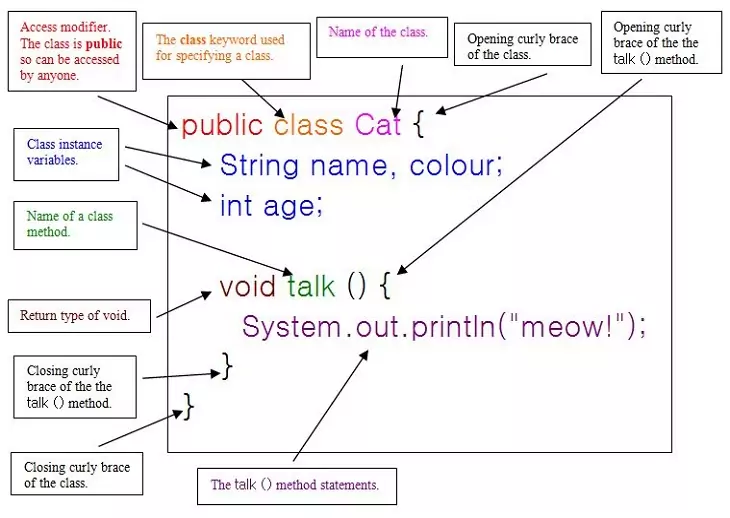
The simple Cat
class above has four members, the three instance variables name
, colour
and age
and the method talk()
. The three instance variables name
,
colour
and age
are the things we know about a Cat
object (object state). The talk()
method is something a Cat
object does (object behaviour).
Ok lets code up this simple class.
package com.server2client;
/*
A Cat Class
*/
public class Cat {
String name, colour;
int age;
void talk() {
System.out.println("meow!");
}
}
Once the class is coded we need a way to test it. Time for our first test class using our trusty old friend the main()
method. So lets create a test class to make sure our Cat
class works:
Running the TestCat
class produces the following output:
package com.server2client;
/*
Test Class for Cat
*/
public class TestCat {
public static void main (String[] args) {
Cat moggy = new Cat(); // Create a Cat
instance
moggy.name = "Tigger"; // Use dot notation to populate cats name
moggy.colour = "Orange"; // Use dot notation to populate cats colour
moggy.age = 12; // Use dot notation to populate cats age
System.out.println("Our " + moggy.colour + " cat called " + moggy.name + " is " + moggy.age);
moggy.talk(); // Use dot notation to execute the talk()
method
}
}
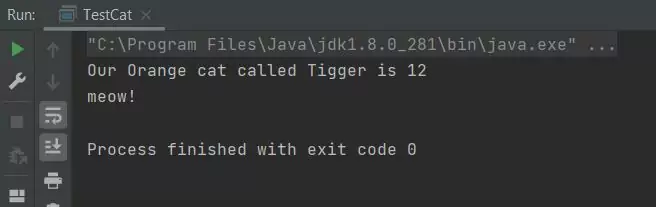
TestCat
class.The above screenshot shows the output of running our TestCat
class. We created a Cat
instance called "moggy" and then gave our cat a name, colour and age using dot notation. Finally we called the
talk()
method to make our cat meow.
Related Quiz
Objects & Classes Quiz 3 - Class Structure & Syntax
Lesson 3 Complete
In this lesson we looked at class structure and syntax within our Java programs.
What's Next?
In the next lesson we look at Java reference variables.