Static OverviewS2C Home « Static Overview
We have used the static keyword throughout the lessons so far, everytime we use the main()
method. When we use the static
keyword preceding our identifiers we are telling the compiler that this is a class member. What do we mean by this? Well class members apply to the class itself and are independent of any instances of the class, in fact our static members know nothing about the instances within our class. We can also use the static
keyword to initiate a block of code on class load.
The following diagram shows a static variable, static initializer block and a static method:
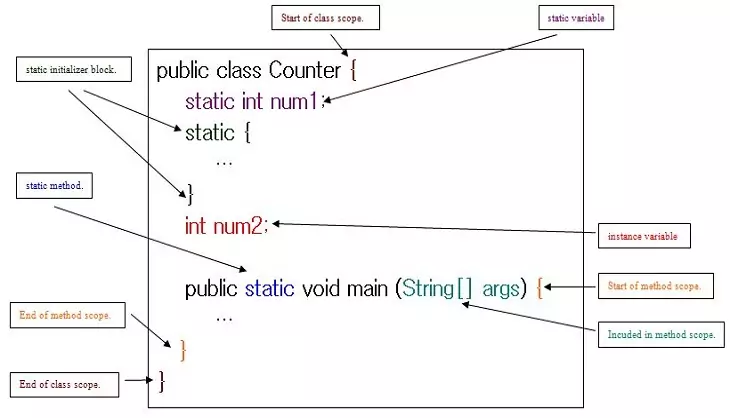
In the first of three lessons on statics we investigate static variables, how we use them and their scope. In the next lesson we look at static methods before finishing our examinations of statics by looking at Java constants.
Static Variables Top
Java comes with three kinds of scope and we name variables according to the scope they reside in as detailed in the table below. In this section we focus on static variables and their scope.
Variable | Scope | Lifetime |
---|---|---|
static | Static variables apply to the class as a whole and are declared within the class but outside a method. | Exists for as long as the class it belongs to is loaded in the JVM . |
instance | Instance variables apply to an instance of the class and are declared within the class but outside a method. | Exists for as long as the instance of the class it belongs to. See the Instance Variables & Scope lesson for more information |
local | Local variables apply to the method they appear in. | Exists until the method it is declared in finishes executing. See the Method Scope lesson for more information |
Static variables are initialized as soon as a class is loaded and before any instances of the class are instantiated.
Like instance variables, static variables are assigned a default value according to their type:
- object -
null
- boolean -
false
- char -
/u0000
- integer types (
byte
,short
,int
andlong
) -0
- floating-point types (
float
,double
) -0.0
Because static variables are independent of any instance they can be used by all instances of the class. To demonstrate what we mean by this lets write some code that keeps count of the instances we create.
package com.server2client;
/*
A Donkey Class
*/
public class Donkey {
int count;
public Donkey() {
count++; // Increment a counter
System.out.println("Our donkey count = " + count);
}
}
Lets write a new test class for our Donkey
.
package com.server2client;
/*
Test Class for Donkey
*/
public class DonkeyTest {
public static void main (String[] args) {
Donkey dan = new Donkey();
Donkey sam = new Donkey();
Donkey liam = new Donkey();
}
}
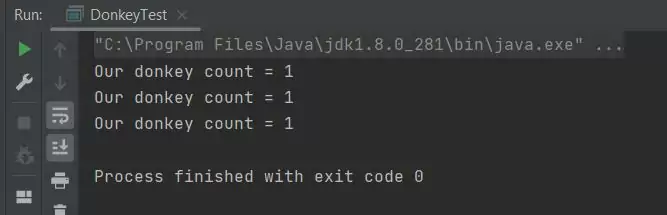
DonkeyTest
class.The above screenshot shows the output of running our DonkeyTest
class. We created three Donkey
instances and printed out a total after each. The total is always 1 as each time we create a new instance of
Donkey
we are creating a new count
variable as this is an instance variable. Lets revamp our Donkey
class and make the instance variable into a static variable:
package com.server2client;
/*
A Donkey Class
*/
public class Donkey {
static int count; // We made this static
public Donkey() {
count++; // Increment a counter
// Prefix statics with the class name
System.out.println("Our donkey count = " + Donkey.count);
}
}
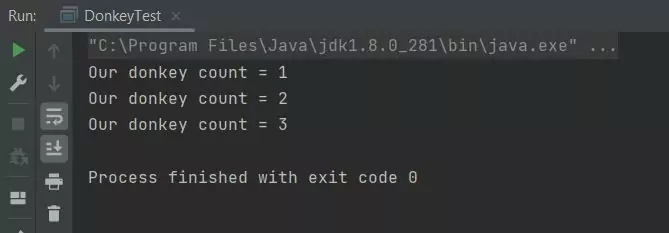
DonkeyTest
class.The above screenshot shows the output of running our DonkeyTest
class. We created three Donkey
instances and printed out a total after each. The total now gets gets incremented each time we create a new
instance of Donkey
just like we want. Static variables apply to the whole class and are initialized when the class is loaded. There is only one copy of each static variable in a class which is shared by all
instances of that class. We prefix statics with the class name to avoid ambiguity and to highlight the fact that this is a static.
Related Quiz
Objects & Classes Quiz 12 - Static Overview
Lesson 12 Complete
In this lesson we investigated what the static
keyword means and how to use static variables.
What's Next?
In the next lesson we look at static methods and how to use them within our Java classes.