Arithmetic OperatorsS2C Home « Arithmetic Operators
Symbols used for mathematical and logical manipulation that are recognized by the compiler are commonly known as operators in Java. In the first of five lessons on operators we look at the arithmetic operators available in Java.
Arithmetic Operators Overview Top
We are familiar with most of the arithmetic operators in the table below from everyday life and they generally work in the same way in Java. The arithmetic operators work with all the Java numeric primitive
types and can also be applied to the char
primitive type.
Operator | Meaning | Example | Result | Notes |
---|---|---|---|---|
+ | Addition | int b = 5; b = b + 5; | 10 | |
- | Subtraction | int b = 5; b = b - 5; | 0 | |
/ | Division | int b = 7; b = 22 / b; | 3 | When used with an integer type, any remainder will be truncated. |
* | Multiplication | int b = 5; b = b * 5; | 25 | |
% | Modulus | int b = 5; b = b % 2; | 1 | Holds the remainder value of a division. |
++ | Increment | int b = 5; b++; | 6 | See below. |
-- | Decrement | int b = 5; b--; | 4 | See below. |
Increment And Decrement Operators
Both these operators can be prepended (prefix) or appended (postfix) to the operand they are used with. When applied to an operand as part of a singular expression it makes no difference whether the increment and decrement operators are applied as a prefix or postfix. With larger expressions however, when an increment or decrement operator precedes its operand, Java will perform the increment or decrement operation prior to obtaining the operand's value for use by the rest of the expression. When an increment or decrement operator follows its operand, Java will perform the increment or decrement operation after obtaining the operand's value for use by the rest of the expression. Lets look at some code to illustrate how this works:
package com.server2client;
/*
Increment And Decrement Operators
*/
public class IncDec {
public static void main (String[] args) {
byte a = 5;
byte b = 5;
// Singular Expressions
a++;
++b;
System.out.println("a = " + a);
System.out.println("b = " + b);
// Larger Expressions
a = 1;
b = a++;
System.out.println("b using a postfix = " + b);
a = 1;
b = ++a;
System.out.println("b using a prefix = " + b);
}
}
Running the IncDec
class produces the following output:
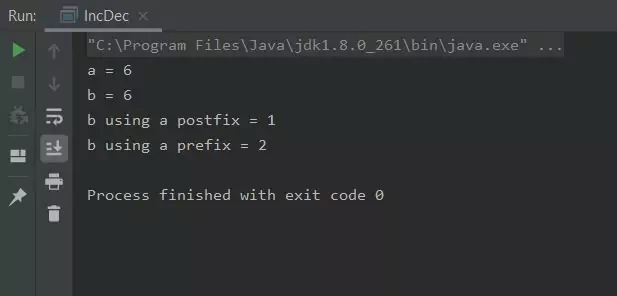
IncDec
class.As you can see with the last two lines of output, the postfix increment is applied after the expression is evaluated, whereas the prefix increment is applied before.
Related Quiz
Fundamentals Quiz 6 - Arithmetic Operators Quiz
Lesson 7 Complete
In this lesson we looked at the arithmetic operators used in Java.
What's Next?
In the next lesson we look at the relational & logical operators used in Java.