Code Structure & SyntaxS2C Home « Code Structure & Syntax
Now we have the basic environment set up we can take a look at the code structure of a Java source file and look at some syntax. In this lesson we do this and then run our very first Java program.
A Look At Java Code Structure Top
A Java source file is made up of a class with the code for the class going inside the class definition. The name of the file that we store the source in, which is also known as a compilation unit, should be the same as the class it contains including any capitalisation when the class is public. The reason for this is the Java compiler will use the name of the public class within our program to create the bytecode version of our source file. Before we go into more details of this lets take a look at the structure of a Java source file using a diagram.
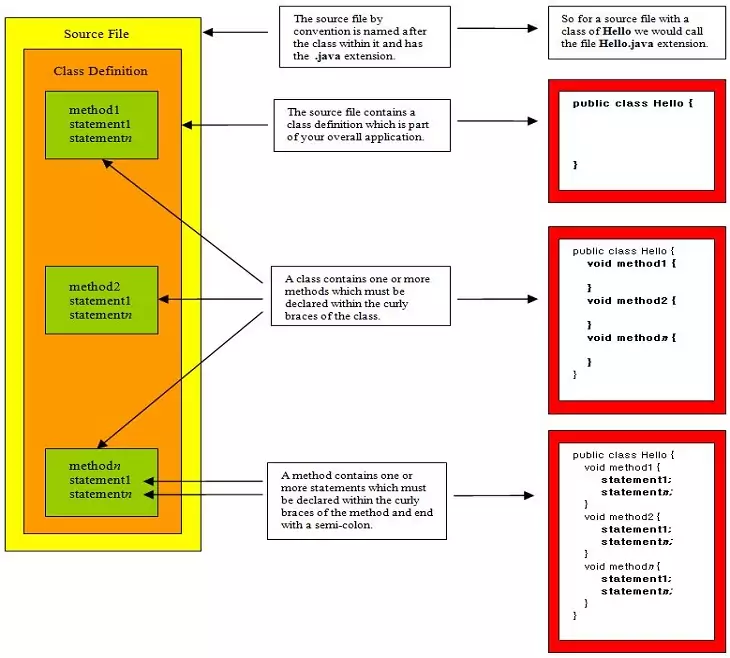
So using the diagram above we can note down a few points about a Java source file:
- If we want to create a class called Hello then by convention and best practice we should save our source file as Hello.java . Always capitalize the first letter of each word used for the class name.
- The
public
keyword preceding the name of the class is an access modifier and in this case means the class can be accessed by anyone.
Only one public class per source file is allowed and if present is what we use as the identifer when we come to compile our source file into bytecode.
If there is no public class present we can call a source file any valid identifier we choose.
An empty source file with nothing in it will also compile without error. - The source file will then contain the
class
keyword followed by the name of the class and a set of curly braces. - We can specify one or more methods within the curly braces of the class, with each method also having a set of curly braces.
- The
void
keyword preceding the name of each method specifies that the method doesn't return any value. This is known as a return type and is mandatory. - We can specify one or more statements within the curly braces of each specified method and each must end with a semi-colon.
There is more to source files than the points raised above but for now this is more than enough to think about.
As a side note Java has a set of coding standards for how to set out your code. Of course with the restrictions of screen sizes the site can't always adhere to these standards. Also in some tests we put more than one statement on a line which is what you will get if you sit the certification. Use the following link Code Conventions for the Java Programming Language to get a copy of coding standards to get into the habit of using them.
The Java main()
Method Top
A Java application is a class or collection of classes that interact with each other to produce the desired results. So how does the application know which class to start off with? Well within our suite of classes one of the classes needs to have a special method called main()
which is used to run the application from the command line. This is achieved by typing the java
command on the command line followed by the name of the class containing the main()
method. Lets take a look at the syntax of the main()
method and see how it fits into a class.
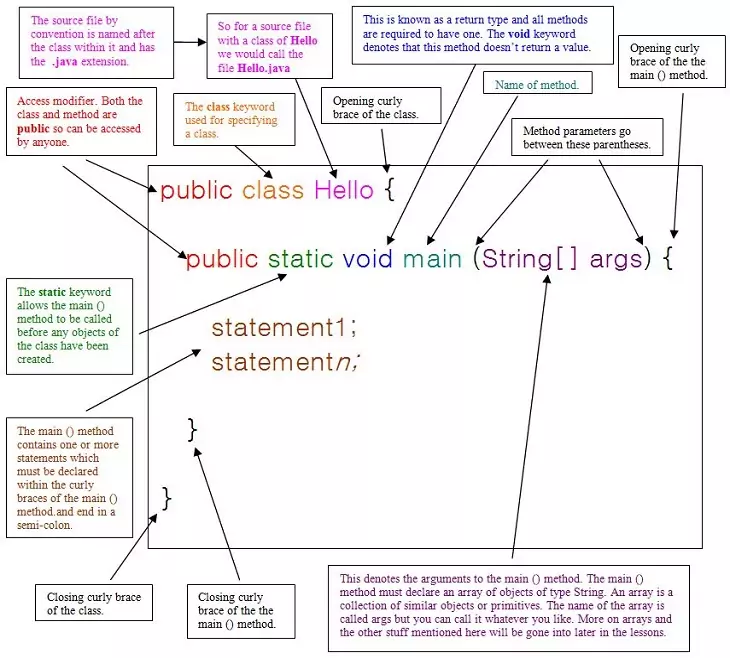
There's a lot of information contained within the diagram above so take your time looking at it and getting a feel for the syntax and how it all hangs together. We will cover things like access modifier, objects and such in later lessons so we don't overload too much at the moment.
Choosing an IDE Top
I am sure you are itching to get into some coding now, but first lets select an IDE, the most popular are Eclipse, IntelliJ IDEA and NetBeans although there are many others.
You can download Eclipse at Download Eclipse and select 'Eclipse IDE for Java Developers' and your operating system.
You can download NetBeans at Download NetBeans and select 'Find out More', click on latest release and your operating system.
You can download IntelliJ IDEA at IntelliJ IDEA and select Ultimate or Community and your operating system. The Ultimate Edition is on a limited trial after which you have to pay unless your a student or working on an open source project.
The choice is yours but for these lessons we will be using IntelliJ IDEA Ultimate Edition. Setting up projects for other IDEs is fairly similar and is explained in the documentation for the IDE in question.
Creating a New Project Top
Lets create a project for our Fundamentals section files, in IntelliJ IDEA this would be Create A New Project (for your very first project) or File -> New -> Project from the top toolbar thereafter. Select the JDK
that was downloaded and press Next as shown in the following screenshot:
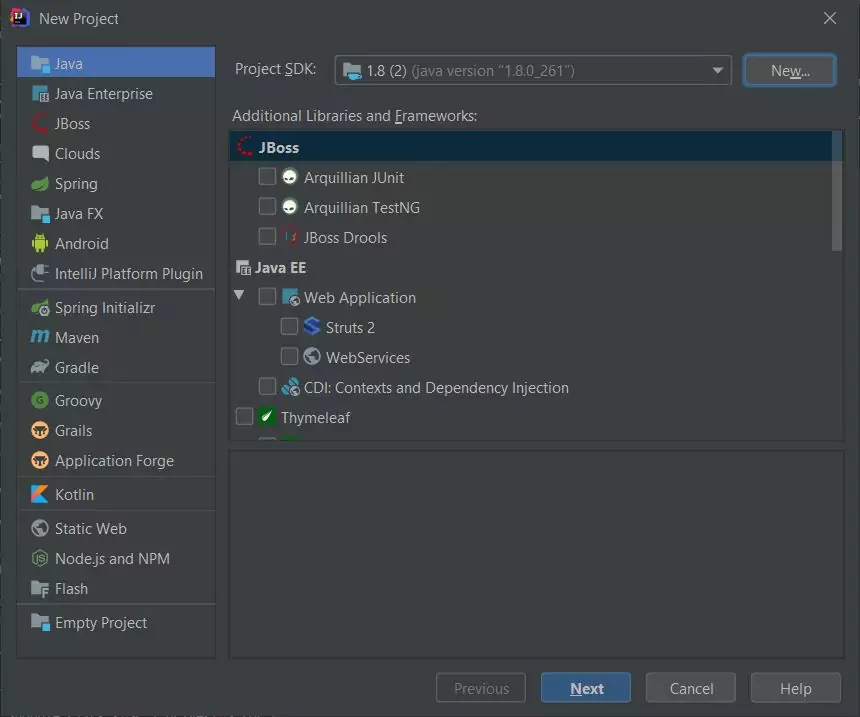
Click Create project from template and press Next as shown in the following screenshot:
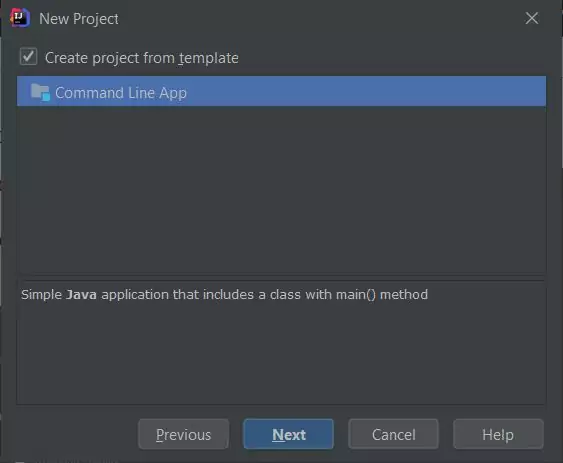
Fill in the details as shown in the following screenshot, changing the location to your choice and press Finish:
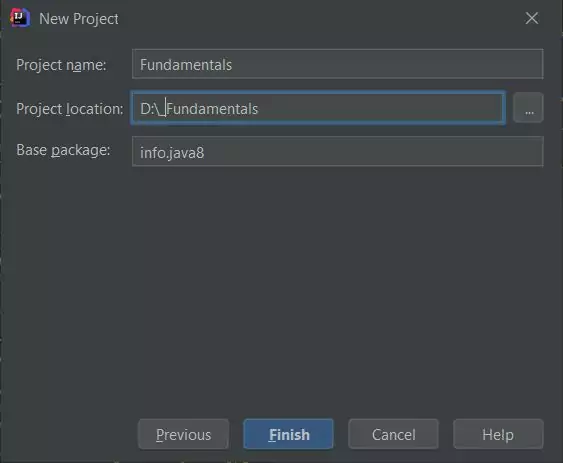
Our First Program Top
IntelliJ has created a Main class which we could use but instead we will create a new class called Hello. Either click on the info.java8 package then File -> New -> Java Class from the top toolbar or right click on the info.java8 package then File -> New -> Java Class. this will bring up the following screenshot:
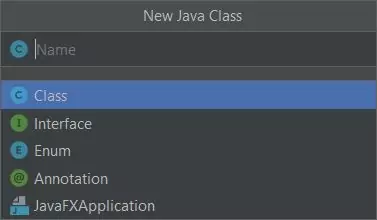
Hello
class.Type in Hello and press enter to create our Hello.java
class and then copy and paste the following code in between the braces
// We need a main() method to begin execution of our code
public static void main (String[] args) {
System.out.println("Hello World");
}
Your class code should now be as shown in the following screenshot:
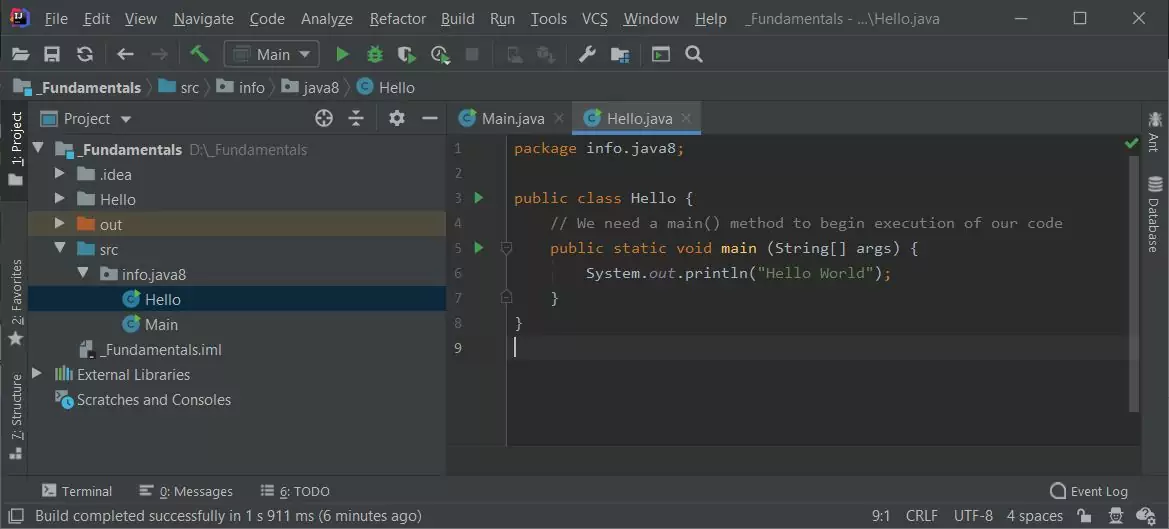
Hello
class.Click on one of the green arrows in the editor and run Hello.main()
. You will see a line of text saying "Hello World" in the run panel as shown in the following screenshot:
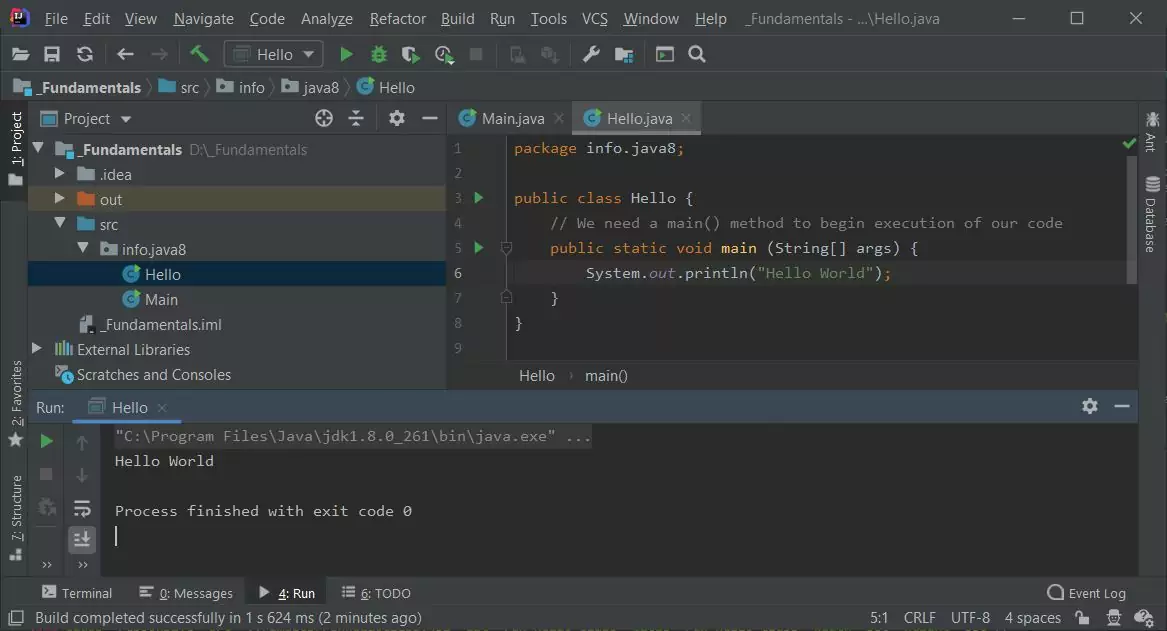
Hello
class.This text is output from the program with the statement System.out.println("Hello World");
. We will go more into syntax like System.out.println
when we look at the Java I/O Overview lesson in the Input & Output section. For now all you need to know is that System
is a predefined class that provides access to the underlying system and out
is the output stream connected to the console. The println()
method displays the message passed to it on a new line. So when joined together (known as chaining) as in our example a message is passed to the console and appears on a new line.
The /* block comment */
allows us to enter comments over multiple lines. The // comment
allows us to put a comment before/after code or on a single line.
Related Quiz
Fundamentals Quiz 1 - Code Structure & Syntax Quiz
Lesson 2 Complete
In this lesson we looked at the syntax and structure of Java and ran our first Java program.
What's Next?
In the next lesson we look at the primitive variable data types available in Java.