Java VariablesS2C Home « Java Variables
So what is a variable? Well a variable allows us to store some information that we may want to manipulate or use. The variable is like a container where we put our information for use and retrieval.
Java comes with two types of variables to choose from, primitives and reference. In this lesson we look at variable syntax, an overview of the primitive variable data types available in Java, naming rules, keywords and Java literals.
We will go into much more detail about individual primitive types in the Primitives - boolean
& char
data types and Primitives - Numeric data types lessons.
We discuss Reference Variables in the Objects & Classes section.
The primitive specified determines which operations are allowed on it and thus operations valid for one primitive type may be invalid for another.
Variable Syntax Top
Java is a strongly typed language and as such cares about the type of the variables we declare. So what does this statement actually mean? Well whenever you declare a variable in Java, you have to give it a type, or the compiler complains when you come to compile your code. You also have to name the variable and there are rules on naming.
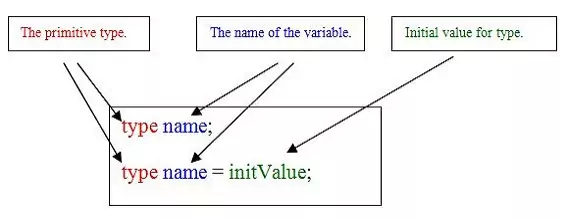
Primitive Types Top
We will go into much more detail about individual primitive types in the Primitives - boolean
& char
data types and Primitives - Numeric data types lessons, for now here's a table of the various primitive types and information regarding each:
Primitive Type | Meaning | Bit Width | Range |
---|---|---|---|
boolean and char | |||
boolean | true/false values | JVM specific | true or false |
char | Character | 16 | 0 to 65535 - ( |
signed numeric integers | |||
byte | 8-bit integer | 8 | -128 to 127 |
short | Short integer | 16 | -32,768 to 32,767 |
int | Integer | 32 | -2,147,483,648 to 2,147,483,647 |
long | Long integer | 64 | -9,233,372,036,854,775,808 to
|
signed floating point | |||
float | Single-precision float | 32 | ≈ ±3.40282347E+38F (6-7 significant decimal digits) |
double | Double-precision float | 64 | ≈ ±1.79769313486231570E+308 (15 significant decimal digits) |
Naming Rules and Keywords Top
These rules apply to all variables, as well as classes and methods (collectively known as identifiers)
- Must start with a letter, the underscore symbol (_) or the dollar sign ($). A name can't start with a number or any other symbol.
- You can use numbers after the first letter, you just can't start with it.
- You cannot use keywords or names recognized by the compiler. See the table below for the words you can't use.
java Keywords | ||||
---|---|---|---|---|
abstract | assert | boolean | break | byte |
case | catch | char | class | const * |
continue | default | do | double | else |
enum | extends | final | finally | float |
for | goto * | if | implements | import |
instanceof | int | interface | long | native |
new | package | private | protected | public |
return | short | static | strictfp | super |
switch | synchronized | this | throw | throws |
transient | try | void | volatile | while |
Keywords marked with an asterisk (*) are not used.
Although not a rule as such it's also important to know that identifiers are case-sensitive.
Naming Rules Test
Try this to test your knowledge of naming rules. We will go into the types as we go through the lesson but you should be able to pick out the invalidly named identifiers from the rules above.
Statement | Valid? | |
---|---|---|
boolean a = true; | yes no | This passes all the rules so is a valid identifier |
char 1char = 'O'; | yes no | Starts with a numeric so invalid |
char enum = 'a'; | yes no | Uses a reserved word so invalid |
int $num = true; | yes no | This passes all the rules so is a valid identifier |
long a123 = 234; | yes no | This passes all the rules so is a valid identifier |
short this = 2; | yes no | Uses a reserved word so invalid |
byte _aByte2 = 'a'; | yes no | This passes all the rules so is a valid identifier |
boolean &b = 'a'; | yes no | Doesnt start with letter, $ or _ so invalid |
Java Literals Top
Java literals are values that are represented in their recognizable everyday form and we have been using them within our coding throughout the lessons so far. The table below gives examples of literals for each primitive type used in this lesson and introduces a few we haven't come across yet.
Type | Examples |
---|---|
boolean | |
boolean | true or false |
char | |
char | 16, 'G', 'a' (numeric or a single character enclosed in single quotes) |
signed integers | |
byte | Negative or positive integers in the range -128 to 127 |
short | Negative or positive integers in the range -32,768 to 32,767 |
int | Negative or positive integers in the range -2,147,483,648 to 2,147,483,647 |
long | Negative or positive integers in the range -9,233,372,036,854,775,808 to 9,233,372,036,854,775,807 Append a l or L to the number or it will be treated as type int .12l, -123456L |
signed floating point | |
float | Append a f or F to the number or it will be treated as type double .25.23f , -1234.56F |
double | 12.34 , -56.72456 |
hexadecimal constants | |
byte, short, int, long | Can be used with any integer type as long as it is in range for that type.0xF , 0xFA |
octal constants | |
byte, short, int, long | Can be used with any integer type as long as it is in range for that type.05 , 012 |
string literals | |
"hello", "welcome back" (enclosed in double quotes) The following are escape character constants that are required for rendering or printing and use the backslash symbol \ to signify the escape sequence.\' Escape a single quote\" Escape a double quote\\ Escape a backslash\b Escape a backspace\f Escape a form feed\n Escape a new line\r Escape a carriage return\t Escape a tab\ooo Escape an octal constant where ooo is the octal constant\uhhhh Escape a hexidecimal constant where hhhh is the hexidecimal constant |
Related Quiz
Fundamentals Quiz 2 - Java Variables Quiz
Lesson 3 Complete
In this lesson we looked at variable syntax, an overview of the primitive variable data types available in Java, naming rules, keywords and Java literals.
What's Next?
In the next lesson we take a much closer look at the boolean
and char
primitive variable data types.