switch
ConstructS2C Home « switch
Construct
In this lesson we take our second look at the conditional statements available in Java. Conditional statements allow us to evaluate an expression and execute some code dependant upon the outcome. There are two conditional statements we can use with Java, the switch
Construct covered here and the if
Construct which we covered in the last lesson along with the tenary ?:
operator. The choice of which conditional statement to use really depends on the expression being evaluated.
The switch
Construct can be used instead of the multiple if....else
construct when we are doing a multiway test on the same value. When this scenario arises the switch
Construct is often a more efficient and elegant way to write our code.
Only certain data types can be used with the switch
Construct as listed in the table below:
Data Type | Java Version |
---|---|
byte , short , int and char | Any version. |
Byte , Short , Integer and Character | These Wrapper classes can be used from Java 5 onwards. |
enum | enum types can be used from Java 5 onwards. |
String | The String type can be used from Java 7 onwards. |
The Switch
Construct Top
The switch
Construct is where we put the expression we are going to evaluate. Each case
constant is evaluated against the switch
expression and the statements within the case
are processed on a match. We have the option to break
from the switch
after this if required. We can also code an optional default
statement, which can act as a kind of catch all and is normally placed, at the end, after the case
statements although this isn't mandatory.
Construct | Description |
---|---|
switch | |
switch (expression) { |
expression can be of type char , byte , short , int or String .constant1 must be a literal of a type compatible with the
switch expression .Execute statements1 on match.Optional break from switch Construct.
constant2 must be a literal of a type compatible with the switch expression .Execute statements2 on match.Optional break from switch Construct.constantN must be a literal of a type compatible with the switch expression .Execute statementsN on match.Optional break from switch Construct. Optional default statement (catch all).Execute defaultStatements .Optional break from switch Construct. |
Construct | Description |
---|---|
nested switch | |
switch (expression1) { [break;] ... [default] defaultStatements [break;] |
This is perfectly legal. |
Switch
without break
Top
As mentioned the break
statement is optional, so what happens when statement execution within a case
is not ended by a break
statement? Well in this scenario all statements following
the matching case
will be executed until a break
statement or the end of the switch
construct is encountered. Lets see some code examples to clarify this:
package com.server2client;
/*
switch Statement (no break)
*/
public class SwitchNoBreak {
public static void main (String[] args) {
int intValue = 11;
// A switch where first case matches.
switch (intValue) {
case 11:
System.out.println("11 matched");
case 22:
System.out.println("22 matched");
case 33:
System.out.println("33 matched");
default:
System.out.println("Default1 no case matches");
}
int intValue2 = 3;
// A switch where third case matches.
switch (intValue2) {
case 1:
System.out.println("1 matched");
case 2:
System.out.println("2 matched");
case 3:
System.out.println("3 matched");
default:
System.out.println("Default2 no case matches");
}
String str = "c";
// A switch where default not at end.
switch (str) {
case "a":
System.out.println("a matched");
default:
System.out.println("Default3 no case matches");
case "b":
System.out.println("b matched");
case "c":
System.out.println("c matched");
}
}
}
Running the SwitchNoBreak
class produces the following output:
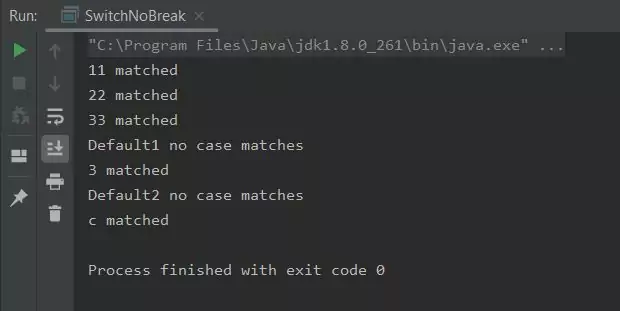
SwitchNoBreak
class.You should see several lines of output with the same values as the screenshot above. In the first example there are no break
statements in the switch
construct at all. So after hitting the matched case, processing
continues with the next case and proceeds through each case in the switch
construct as well as the default
statement.
In the second example processing continues after the matched case (3) and proceeds through the default
statement as there is no break
statement.
In the third example processing continues after the matched case ("c") but doesn't process the default
statement as this is higher up in the construct.
Switch
with break
Top
Lets examine some code to see how the switch
Construct works with break
statement:
package com.server2client;
/*
switch Statement (break impact)
*/
public class SwitchBreak {
public static void main (String[] args) {
int intValue = 33;
// A switch where a case matches.
switch (intValue) {
case 11:
System.out.println("11 matched");
break;
case 22:
System.out.println("22 matched");
break;
case 33:
System.out.println("33 matched");
break;
default:
System.out.println("Default1 as no case matches");
}
int intValue2 = 4;
// A switch where no case matches.
switch (intValue2) {
case 1:
System.out.println("1 matched");
break;
case 2:
System.out.println("2 matched");
break;
case 3:
System.out.println("3 matched");
break;
default:
System.out.println("Default2 as no case matches");
}
}
}
Running the SwitchBreak
class produces the following output:
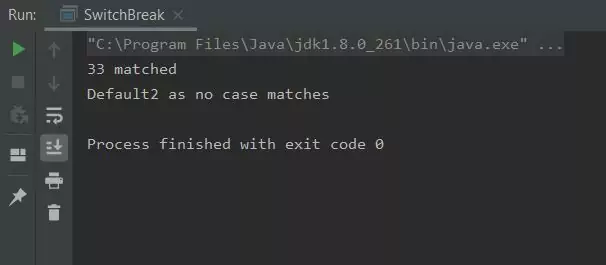
SwitchBreak
class.You should see 2 lines of output with the same values as the screenshot above. Its worth mentioning the break
statement and what it does. The break
statement causes the program flow to exit from
the switch
Construct and resume at the next statement following the switch
construct. The default
statement is also optional and acts as a bucket to catch anything not covered by the
case
statements; thus the default
statement is generally coded as the last case
within the switch
construct, but can be coded anywhere.
Related Quiz
Fundamentals Quiz 12 - switch
Construct Quiz
Lesson 13 Complete
In this lesson we investigated the switch
conditional statement.
What's Next?
In the next lesson we take our first look at loop statements when we look at the for
construct.