JSP Page AnatomyS2C Home « JSP Page Anatomy
In the Introduction to jsp lesson we wrote our first JSP using HTML and we saw how the HTML was translated into Java implementation code in the JSP Lifecycle lesson. But there is much more to a JSP page than this and in this lesson we look at the anatomy of JSP by investigating the various components that can be used within a JSP page.
A JSP page is made up of template data and/or syntactic elements, each of which can be composed of various components as illustrated by the following diagram:
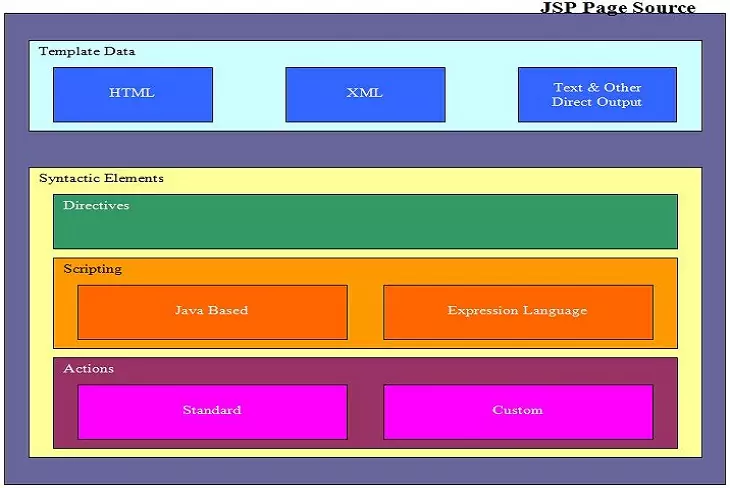
Template DataTop
We have already seen an example of template data when we wrote our first JSP which was entirely composed of HTML. When we inspected the translated code we saw how the container had wrapped our HTML tags within
out.write
statements as shown in the code snippet below:
...
out.write("<!DOCTYPE html>\r\n");
out.write("<html>\r\n");
out.write(" <head><title>Our First JSP</title></head> \r\n");
out.write(" <body>\r\n");
out.write(" <p>We just wrote our first JSP!</p>\r\n");
out.write(" </body>\r\n");
out.write("</html>\r\n");
...
XML & Direct Output Before Translation
The other types of template data are XML and direct output we put into our JSP pages, such as text. But how does other template data such as XML or plain text that we put in our JSP pages get translated? The following code snippet shows some XML and plain text before translation:
// Pre translation
<xml version='1.0'>
<week date='01/01/2012 '><day activity='shop '><loc>London</loc></day></week>
<week date='02/01/2012 '><day activity='drive '><loc>Cardiff</loc></day></week>
<week date='03/01/2012 '><day activity='eat '><loc>Crewe</loc></day></week></xml>
Some text
XML & Direct Output After Translation
Following is how our XML and plain text after translation, it has all been wrapped within out.write
statements just like our HTML tags were. Also notice how our Java comment is treated the same as our
plain text and also wrapped in out.write
statements.
...
out.write("// Pre translation\r\n");
out.write("<xml version='1.0'>\r\n");
out.write("<week date='01/01/2012 '><day activity='shopping '><loc>London</loc></day></week>\r\n");
out.write("<week date='02/01/2012 '><day activity='drive '><loc>Cardiff</loc></day></week>\r\n");
out.write("<week date='03/01/2012 '><day activity='eat '><loc>Crewe</loc></day></week></xml>\r\n");
out.write("\r\n");
out.write("Some text");
...
So all our template data is treated the same way, it gets sent without any translation and just gets wrapped in out.write
statements.
Syntactic ElementsTop
Syntactic elements are anything within a JSP page that need to be translated prior to compilation and are recognized by the translator via a standard sets of characters that mark the beginning an end of the syntactic element. Syntactic elements come in three flavours, these being:
- Directives - Directive elements give the translator instructions on how a JSP page should be translated into a servlet.
- Scripting - Scripting elements incorporate code into a JSP page and can be either Java based or use the Expression Language (EL).
- Actions - Action elements incorporate code into a JSP page to perform some type of operation and can be 'standard' actions that come as part of the container package or 'custom actions which are bespoke.
We will talk briefly about each of the syntactic element types in the next few sections, although each is covered in much more detail in the relevant lesson.
Directives OverviewTop
Directives are input into a JSP page using the directive start tag, followed by the directive name and any directive attributes and terminated with the directive end tag. In total there are six directives in the
jsp specification these being page
, include
, taglib
, tag
, attribute
and variable
.
The following code shows examples of page directives, the whitespace after the @
symbol is optional but aids readability. The comma
symbol is used to delimit attributes.
<%@ page import="java.util.Date, java.util.List" %>
// Same as above but better to use top version
<%@page import="java.util.Date" %>
<%@page import="java.util.List" %>
The page
and include
directives are discussed in the JSP Directives lesson.
The taglib
, tag
, attribute
and variable
directives are discussed in the JavaServer Pages Standard Tag Libraries 1.2 section.
Scripting Elements OverviewTop
There are two kinds of scripting element available for input into a JSP page, these being the traditional Java-based scripting or the newer Expression Language (EL) approach. Both allow us to incorporate dynamic information into a JSP page as well execute presentation logic.
Java-Based ScriptingTop
Java-based scripting comes in four varieties consisting of scriptlets, expressions, declarations and comments. A brief description of each type of Java-based scripting is given below with code examples.
Scriptlets
Scriptlets are blocks of Java code that get translated and placed in the _jspService()
method.
Scriptlets start with <%
and end with %>
.
The following code snippet shows an example of using a scriplet.
<%
if (true) {
out.println("true");
} else {
out.println("false");
}
%>
Expressions
Expressions are evaluated and the result output to the JSP page.
Expressions start with <%=
and end with %>
.
The following code snippet shows an example of using an expression and as you can see there is no semicolon at the end when using expressions.
<%= new java.util.Date() %>
Declarations
Declarations are used to declare vaiables and methods for use in a JSP page.
Declarations start with <%!
and end with %>
.
The following code snippet shows an example of using a declaration.
<%!
public String getDate() {
return java.util.Date();
}
%>
Comments
Comments enclose lines that are completely ignored by the JSP page translator.
Comments start with <%--
and end with --%>
.
The following code snippet shows a commented declaration and expression.
<%-- Method declaration to retrieve todays date --%>
<%!
public java.util.Date getDate() {
return java.util.Date();
}
%>
<%-- Call declared method and output todays date --%>
<%= getDate() %>
Scriptlets, expressions, declarations and comments scripting elements are discussed in the Scripting Elements lesson.
Expression LanguageTop
Expression Language expressions allows us to output elements to JSP pages without the use of Java statements.
Expression Language statements start with {$
and end with }
.
<%
request.setAttribute("bird", "Great crested grebe");
%>
<%-- EL statement enclosed in p HTML tags to output request attribute follows --%>
<p>${bird}</p>
Expression Language syntax is discussed in the Expression Language 2.1 section.
Action Elements OverviewTop
Standard actions and custom actions are the last type of syntactic element and are translated into Java code that performs an operation or invokes a method, much like the other scripting elements we have seen. The major difference with actions is that they are written using XML syntax to achieve the same results as other scripting elements which are Java-based.
The following code shows an example of a standard action using start and end tags and a self closing tag.
<%-- Standard action using start and end tag --%>
<prefix:tagname attr="value"></prefix:tagname>
<%-- Standard action using self closing tag --%>
<prefix:tagname attr="value">
Standard actions are discussed in the JavaBean Standard Actions lesson.
Custom actions are discussed in the JavaServer Pages Standard Tag Libraries 1.2 section.
In this lesson we looked at the anatomy of JSP by investigating the various components that can be used within a JSP page.
What's Next?
In the next lesson we look at implicit objects which allow us to access the Servlet and JSP objects created by the container.