Scripting ElementsS2C Home « Scripting Elements
There are two kinds of scripting elements available for input into a JSP page, these being the traditional Java-based scripting or the newer Expression Language (EL) approach. Both allow us to incorporate dynamic information into a JSP page as well execute presentation logic. We will look at EL syntax in great detail in the Expression Language 2.1 section of the site. In this lesson we look at the different Java-based scripting elements available and how we can use them.
Java-based scripting comes in four varieties consisting of scriptlets, expressions, declarations and comments. We will look at each type of Java-based scripting in the sections below.
ScriptletsTop
Scriptlets are blocks of Java code that get translated and placed in the _jspService()
method.
There are a few basic rules to learn when using scriptlets:
- Scriptlets start with
<%
and end with%>
. - You can have multiple scriptlets within a JSP page.
- Variables defined within a scriplet are visible and usable by other scriplets defined below it.
- Semicolons are required at the end of Java source statements.
The following code shows an example of using two scriplets and using the variable defined in the first scriptlet in the second. Scriptlets can be placed on the same line as their tags although separating the start and end tags from the Java code block makes the synyax more readable.
<!DOCTYPE html>
<html>
<head><title>Using Scriptlets </title></head>
<body>
<h1>JSP Page Using Two Scriptlets</p>
<%
int aVar = 0;
for (int i=0; i<5; i++) {
aVar = i;
out.println("Value of aVar: " + aVar + "<br>");
}
%>
<% out.println("Variable from scriptlet defined above. Value of aVar: " + aVar); %>
</body>
</html>
The following screenshot shows the results of deploying and running the above JSP page:
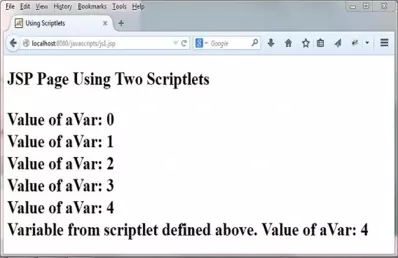
ExpressionsTop
Expressions are evaluated and the result passed to the print()
method of the out
implicit object. Expressions are Java code that get translated and placed in the _jspService()
method.
There are a few basic rules to learn when using expressions:
- Expressions start with
<%=
and end with%>
. - You can have multiple expressions within a JSP page.
- Semicolons are not required and should not be used at the end of the expression.
The following code shows an example of using an expression and as you can see there is no semicolon at the end of the expressions.
<!DOCTYPE html>
<html>
<head><title>Using Expressions </title></head>
<body>
<h1>JSP Page Using An Expression</p>
<%= new java.util.Date() %>
</body>
</html>
The following screenshot shows the results of deploying and running the above JSP page:
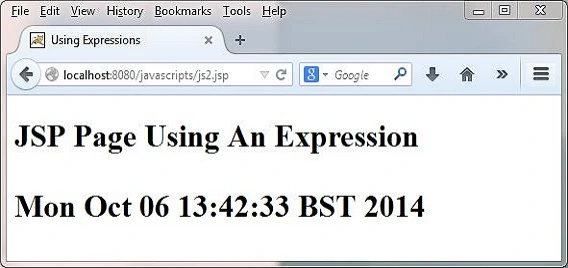
DeclarationsTop
Declarations are used to declare vaiables and methods for use in a JSP page that we don't want translated into the _jspService()
method. This can be any entities that you could legally place within a
servlet such as static variables and methods, instance variables and methods or even inner classes.
Using declarations is also the only way we can override the jspInit()
and jspDestroy()
lifecycle methods.
There are a few basic rules to learn when using declarations:
- Declarations start with
<%!
and end with%>
. - You can have multiple declarations within a JSP page.
- Semicolons are required at the end of Java source statements.
The following code shows an example of using declarations to override the jspInit()
and jspDestroy()
lifecycle methods.
<!DOCTYPE html>
<html>
<head><title>Using Declarations </title></head>
<body>
<h1>JSP Page Overriding jspInit() And jspDestroy() Lifecycle Methods</p>
<%!
public void jspInit() {
System.out.println("Overriding the jspInit() lifecycle method");
}
%>
<%!
public void jspDestroy() {
System.out.println("Overriding the jspDestroy() lifecycle method");
}
%>
</body>
</html>
The following screenshot shows the results of deploying and running the above JSP page:
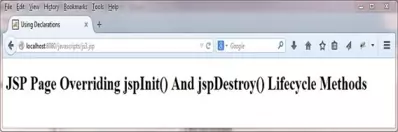
The following screenshot shows the tomcat log:
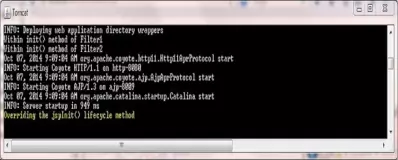
The code below shows the translation of the jspInit()
and jspDestroy()
lifecycle methods for the JSP page above which I called js3.jsp
and that got renamed to js3_jsp.java
and is done using the Tomcat container and will differ for other containers. The translated code for Tomcat can be found within a subdirectory of the work
directory within your Tomcat installation. On my machine the full path is:
C:\apache-tomcat-6.0.37\work\Catalina\localhost\javascripts\org\apache\jsp\
package org.apache.jsp;
import javax.servlet.*;
import javax.servlet.http.*;
import javax.servlet.jsp.*;
public final class js3_jsp extends org.apache.jasper.runtime.HttpJspBase
implements org.apache.jasper.runtime.JspSourceDependent {
public void jspInit() {
System.out.println("Overriding the jspInit() lifecycle method");
}
public void jspDestroy() {
System.out.println("Overriding the jspDestroy() lifecycle method");
}
...
CommentsTop
Comments enclose lines that are completely ignored by the JSP page translator.
There are a few basic rules to learn when using comments:
- Comments start with
<%--
and end with--%>
. - You can have multiple comments within a JSP page.
The following code snippet shows a commented declaration and expression.
<%-- Method declaration to retrieve todays date --%>
<%!
public java.util.Date getDate() {
return java.util.Date();
}
%>
<%-- Call declared method and output todays date --%>
<%= getDate() %>
You can also use HTML comments which are not processed by the container and are sent to the browser as they were typed. This is useful when we want to comment aspects of a JSP page that we want available when viewing the HTML source in a browser.
<!DOCTYPE html>
<html>
<head><title>JSP Page Using include Directive </title></head>
<body>
<h1>Using the <code>include</code> Directive</p>
<p>Some paragraph text</p>
<!-- Following is the included.jspf fragment -->
<%@ include file="included.jspf" %>
<p>Some paragraph text</p>
</body>
</html>
Lesson 6 Complete
In this lesson we looked at scripting elements which are the second type of syntatcic elments available for use within JSP pages.
What's Next?
In the next lesson we look at the jsp standard actions that relate to JavaBeans and how to use them.