Trader View 2S2C Home « Trader View 2
In this lesson we take a second look at the view part of the MVC paradigm and code the second lot of view components for the trader. These view components consist of the ten remaining dynamic JSP components to be coded for the trader:
Trader Dynamic Boot Sale Diary Pages Using JSP
The trader JSP pags are grouped on the facilities page by stock item, boot sale location, trader diary, reporting and mailing. In this section we show the code and screenshots produced from the code for boot sale diary management.
Add Boot Sale Diary Record - AddBSDiary.jsp
Top
The trader needs to be able to add a boot sale diary record and we need to validate the mandatory fields to ensure they are entered before a boot sale diary record is added to the database. The following scriptless JSP code shows how to do this :-
<%-- AddBSDiary.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="displayData" value="">
<c:set var="errorMsg" value="">
<c:set var="thisPage" value="traderfacilities?${pageContext.request.queryString}">
<c:set var="bFormSuccess" value="false">
<%-- Assign values to all the form controls coming in. These values will also
be used to dynamically re-populate the form for re-submission should an error occur. --%>
<c:set var="selectDay" value="${param.selectDay}">
<c:set var="selectMonth" value="${param.selectMonth}">
<c:set var="selectYear" value="${param.selectYear}">
<c:set var="selectName" value="${param.selectName}">
<c:set var="counter" value="${sessionScope.countlocnames}">
<%-- Process the values if user submitted the form. --%>
<c:if test="${pageContext.request.method == 'POST'}">
<%-- Perform validation for required items. --%>
<c:choose>
<c:when test="${selectDay == ''}">
<c:set var="noselectDay" value="<li>You must select a day.</li>"/>
</c:when>
<c:otherwise>
<c:set var="noselectDay" value=""/>
</c:otherwise>
</c:choose>
<c:choose>
<c:when test="${selectMonth == ''}">
<c:set var="noselectMonth" value="<li>You must select a month.</li>"/>
</c:when>
<c:otherwise>
<c:set var="noselectMonth" value=""/>
</c:otherwise>
</c:choose>
<c:choose>
<c:when test="${selectYear == ''}">
<c:set var="noselectYear" value="<li>You must select a year.</li>"/>
</c:when>
<c:otherwise>
<c:set var="noselectYear" value=""/>
</c:otherwise>
</c:choose>
<c:choose>
<c:when test="${selectName == ''}">
<c:set var="noselectName" value="<li>You must select a boot sale name.</li>"/>
</c:when>
<c:otherwise>
<c:set var="noselectName" value=""/>
</c:otherwise>
</c:choose>
<c:set var="error" value="${noselectDay}${noselectMonth}${noselectYear}${noselectName}">
<c:choose>
<c:when test="${!empty error}">
<%-- There are 1 or more error messages, so display them --%>
<c:set var="errorstart" value="<p>Please correct the following error(s) in order to properly submit the form</p><ul>">
<c:set var="errorMsg" value="${errorstart}${error}">
</c:when>
<c:when test="${empty error}">
<%-- No errors, store user entry for later use --%>
<c:set var="bFormSuccess" value="true">
<c:set var="succ1" value="<strong>Boot Sale Day: </strong>${selectDay}">
<c:set var="succ2" value="<br><strong>Boot Sale Month: </strong>${selectMonth}">
<c:set var="succ3" value="<br><strong>Boot Sale Year: </strong>${selectYear}">
<c:set var="succ4" value="<br><strong>Boot Sale Name: </strong>${selectName}">
<c:set var="displayData" value="${succ1}${succ2}${succ3}${succ4}">
</c:when>
</c:choose>
</c:if>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Add Boot Sale Diary</title>
<style type="text/css">
.style5{margin-bottom: 0px;}
</style>
</head>
<body>
<%-- Keep showing the form until bFormSuccess is true --%>
<c:choose>
<c:when test="${!bFormSuccess}">
<form name='form1' action='${thisPage}' method='post'>
<p><strong>Add Boot Sale Diary</strong></p>
<table style="width:100;" class="style2">
<tr><td valign="top">Day</td><td>
<select name="selectDay" >
<c:choose>
<c:when test="${!empty selectDay}">
<option value='${selectDay}'>${selectDay} </option>
<c:forEach var="idx" begin="1" end="31">
<option value="${idx}">${idx}</option>
</c:forEach>
</c:when>
<c:otherwise>
<option value=''>Select Day </option>
<c:forEach var="idx" begin="1" end="31">
<option value="${idx}">${idx}</option>
</c:forEach>
</c:otherwise>
</c:choose>
</select></td></tr>
<tr><td valign="top">Month</td><td>
<select name="selectMonth" >
<c:choose>
<c:when test="${!empty selectMonth}">
<option value='${selectMonth}'>${selectMonth} </option>
<c:forEach var="idx" begin="1" end="12">
<option value="${idx}">${idx}</option>
</c:forEach>
</c:when>
<c:otherwise>
<option value=''>Select Month </option>
<c:forEach var="idx" begin="1" end="12">
<option value="${idx}">${idx}</option>
</c:forEach>
</c:otherwise>
</c:choose>
</select></td></tr>
<tr>
<tr><td valign="top">Year</td><td>
<select name="selectYear" >
<c:choose>
<c:when test="${!empty selectYear}">
<option value='${selectYear}'>${selectYear} </option>
<c:forEach var="idx" begin="2016" end="2031">
<option value="${idx}">${idx}</option>
</c:forEach>
</c:when>
<c:otherwise>
<option value=''>Select Year </option>
<c:forEach var="idx" begin="2016" end="2031">
<option value="${idx}">${idx}</option>
</c:forEach>
</c:otherwise>
</c:choose>
</select></td></tr>
<tr><td valign="top">Boot Sale Location</td>
<td><select name="selectName" style="width: 150px;">
<c:choose>
<c:when test="${!empty selectName}">
<option value='${selectName}'>${selectName} </option>
<c:forEach var="idx" begin="0" end="${counter}">
<option value="${sessionScope.bslocNames[idx]}">${sessionScope.bslocNames[idx]}</option>
</c:forEach>
</c:when>
<c:otherwise>
<option value=''>Select Name </option>
<c:forEach var="idx" begin="0" end="${counter}">
<option value="${sessionScope.bslocNames[idx]}">${sessionScope.bslocNames[idx]}</option>
</c:forEach>
</c:otherwise>
</c:choose>
</select></td></tr>
<tr><td> </td></tr><tr><td style="width: 124px; height: 45px;" valign="top"><input type="submit" name="Button1" type="button" value="Submit"> </td></tr>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
<tr><td> </td></tr><tr><td style="color:red" colspan="2">${errorMsg}</td></tr>
</table>
</form>
</c:when>
<c:when test="${bFormSuccess}">
<c:if test="${selectDay < 10}">
<c:set var="selectDay" value="0${selectDay}">
</c:if>
<c:if test="${selectMonth < 10}">
<c:set var="selectMonth" value="0${selectMonth}">
</c:if>
<c:set var="date" value="${selectDay}/${selectMonth}/${selectYear}">
<jsp:forward page="/processinput" >
<jsp:param name="action" value="AddBSDiary">
<jsp:param name="date" value="${date}">
<jsp:param name="name" value="${selectName}">
<jsp:param name="displayData" value="${displayData}">
</jsp:forward >
</c:when>
</c:choose>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as AddBSDiary.jsp
.
Add Boot Sale Diary Screenshots
The following screenshot shows what the Add Boot Sale Diary page looks like:-
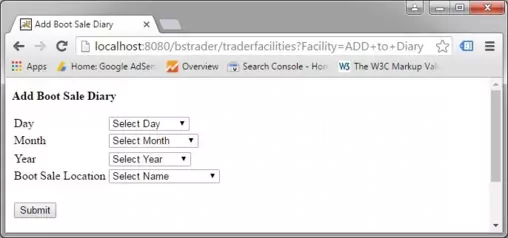
The following screenshot shows the results of adding a boot sale diary:-
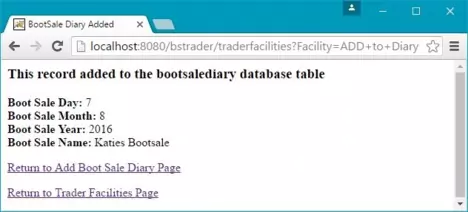
Select Boot Sale Diary Record - SelectBSDiary.jsp
Top
The trader needs to be able to select a boot sale diary record for modification from all boot sale diary records retrieved from the database. The following scriptless JSP code shows how to do this :-
<%-- SelectBSDiary.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="displayData" value="">
<c:set var="errorMsg" value="">
<c:set var="thisPage" value="traderfacilities?${pageContext.request.queryString}">
<c:set var="bFormSuccess" value="false">
<%-- Assign values to all the form controls coming in. These values will also
be used to dynamically re-populate the form for re-submission should an error occur. --%>
<c:set var="selectDate" value="${param.selectDate}">
<c:set var="counter" value="${sessionScope.countbsdDates}">
<%-- Process the values if user submitted the form. --%>
<c:if test="${pageContext.request.method == 'POST'}">
<%-- Perform validation for required items. --%>
<c:choose>
<c:when test="${selectDate == ''}">
<c:set var="noselectDate" value="<li>You must select a date.</li>"/>
</c:when>
<c:otherwise>
<c:set var="noselectDate" value=""/>
</c:otherwise>
</c:choose>
<c:set var="error" value="${noselectDate}">
<c:choose>
<c:when test="${!empty error}">
<%-- There are 1 or more error messages, so display them --%>
<c:set var="errorstart" value="<p>Please correct the following error(s) in order to properly submit the form</p><ul>">
<c:set var="errorMsg" value="${errorstart}${error}">
</c:when>
<c:when test="${empty error}">
<%-- No errors, store user entry for later use --%>
<c:set var="bFormSuccess" value="true">
<c:set var="succ1" value="<strong>Boot Sale Date: </strong>${selectDate}">
<c:set var="displayData" value="${succ1}">
</c:when>
</c:choose>
</c:if>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Select Boot Sale Diary</title>
<style type="text/css">
.style5{margin-bottom: 0px;}
</style>
</head>
<body>
<%-- Keep showing the form until bFormSuccess is true --%>
<c:choose>
<c:when test="${!bFormSuccess}">
<form name='form1' action='${thisPage}' method='post'>
<p><strong>Select Boot Sale Diary Record to modify</strong></p>
<table style="width:100;border:4;" class="style2">
<c:choose>
<c:when test="${counter ge 0}">
<tr><td style="width: 150px" valign="top">Boot Sale Date<br></td>
<td><select name="selectDate" style="width: 150px;">
<option value=''>Select Date </option>
<c:forEach var="idx" begin="0" end="${counter}">
<option value="${sessionScope.bsdDates[idx]}">${sessionScope.bsdDates[idx]}</option>
</c:forEach>
</select></td></tr>
<tr><td> </td></tr><tr><td style="width: 124px; height: 45px;" valign="top"><input type="submit" name="Button1" type="button" value="Submit"> </td></tr>
</c:when>
<c:otherwise>
<c:set var="errorMsg" value="<p>There are no Diary Entries to select!</p>">
</c:otherwise>
</c:choose>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
<tr><td> </td></tr><tr><td style="color:red" colspan="2">${errorMsg}</td></tr>
</table>
</form>
</c:when>
<c:when test="${bFormSuccess}">
<jsp:forward page="/processinput" >
<jsp:param name="action" value="SelectBSDiary">
<jsp:param name="action2" value="MODIFIED Diary">
<jsp:param name="date" value="${selectDate}">
<jsp:param name="displayData" value="${displayData}">
</jsp:forward >
</c:when>
</c:choose>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as SelectBSDiary.jsp
.
Select Boot Sale Diary Screenshot
The following screenshot shows what the Select Boot Sale Diary page looks like:-
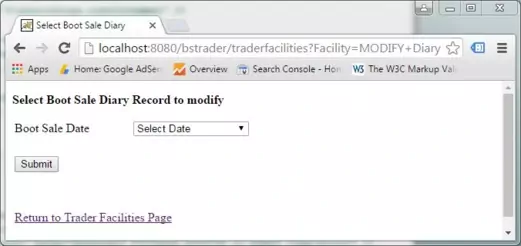
Modify Boot Sale Diary Record - ModifyBSDiary.jsp
Top
The trader needs to be able to modify a boot sale diary record and we need to validate the modified fields to ensure they are entered before a boot sale diary record is added to the database. The following scriptless JSP code shows how to do this :-
<%-- ModifyBSDiary.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="displayData" value="">
<c:set var="errorMsg" value="">
<c:set var="thisPage" value="traderfacilities?${pageContext.request.queryString}">
<c:set var="bFormSuccess" value="false">
<c:set var="counter" value="${sessionScope.countlocnames}">
<c:choose>
<%-- Display the form and values first time in. --%>
<c:when test="${requestScope.first == 'firsttimein'}">
<c:set var="date" value="${requestScope.date}">
</c:when>
<%-- Process the values if user submitted the form. --%>
<c:when test="${pageContext.request.method == 'POST'}">
<%-- Assign values to all the form controls coming in. These values will also be used
to dynamically re-populate the form for re-submission should an error occur. --%>
<c:set var="date" value="${param.date}">
<c:set var="selectName" value="${param.selectName}">
<%-- Perform validation for required items. --%>
<c:choose>
<c:when test="${selectName == ''}">
<c:set var="noselectName" value="<li>You must supply the name for the boot sale.</li>"/>
</c:when>
<c:otherwise>
<c:set var="noselectName" value=""/>
</c:otherwise>
</c:choose>
<c:set var="error" value="${noselectName}">
<c:choose>
<c:when test="${!empty error}">
<%-- If there are 1 or more error messages, display them --%>
<c:set var="errorstart" value="<p>Please correct the following error(s) in order to properly submit the form</p><ul>">
<c:set var="errorMsg" value="${errorstart}${error}">
</c:when>
<c:when test="${empty error}">
<%-- No errors, store user entry for later use --%>
<c:set var="bFormSuccess" value="true">
<c:set var="succ1" value="<strong>Boot Sale Date: </strong>${date}">
<c:set var="succ2" value="<br><strong>Boot Sale Location: </strong>${selectName}">
<c:set var="displayData" value="${succ1}${succ2}">
</c:when>
</c:choose>
</c:when>
</c:choose>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Modify a Boot Sale Diary Record</title>
<style type="text/css">
.style5{margin-bottom: 0px;}
</style>
</head>
<body>
<%-- Keep showing the form until bFormSuccess is true --%>
<c:choose>
<c:when test="${!bFormSuccess}">
<form name='form1' action='${thisPage}' method='post'>
<p><strong>Modify a Boot Sale Diary record</strong></p>
<table style="width:100;" class="style2">
<tr><td valign="top">Boot Sale Date:<br></td><td><input type="text" name="date" value="${date}" readonly="readonly"></td></tr>
<tr><td valign="top">Boot Sale Location</td>
<td><select name="selectName" style="width: 150px;">
<c:choose>
<c:when test="${!empty selectName}">
<option value='${selectName}'>${selectName} </option>
<c:forEach var="idx" begin="0" end="${counter}">
<option value="${sessionScope.bslocNames[idx]}">${sessionScope.bslocNames[idx]}</option>
</c:forEach>
</c:when>
<c:otherwise>
<option value=''>Select Name </option>
<c:forEach var="idx" begin="0" end="${counter}">
<option value="${sessionScope.bslocNames[idx]}">${sessionScope.bslocNames[idx]}</option>
</c:forEach>
</c:otherwise>
</c:choose>
</select></td></tr>
<tr><td> </td></tr><tr><td style="width: 124px; height: 45px;" valign="top"><input type="submit" name="Button1" type="button" value="Submit"> </td></tr>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/traderfacilities?Facility=MODIFY+Diary'>Return to Select Boot Sale Diary Record To Modify</a></td></tr>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
<tr><td> </td></tr><tr><td style="color:red" colspan="2">${errorMsg}</td></tr>
</table>
</form>
</c:when>
<c:when test="${bFormSuccess}">
<jsp:forward page="/processinput" >
<jsp:param name="action" value="ModifyBSDiary">
<jsp:param name="label" value="ModifiedBSDiary">
<jsp:param name="date" value="${date}">
<jsp:param name="name" value="${selectName}">
<jsp:param name="displayData" value="${displayData}">
</jsp:forward >
</c:when>
</c:choose>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as ModifyBSDiary.jsp
.
Modify Boot Sale Diary Screenshots
The following screenshot shows what the Modify Boot Sale Diary page looks like:-
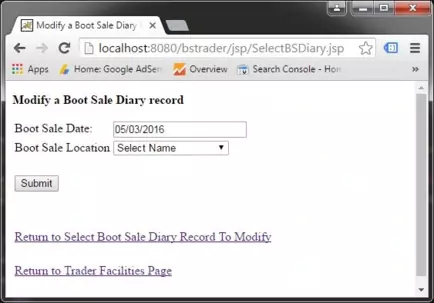
The following screenshot shows what the results of modifying a boot sale diary:-
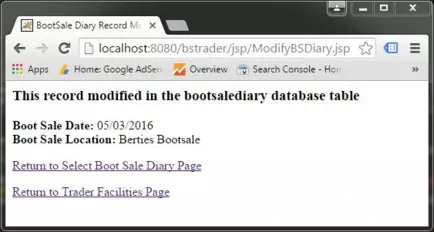
Delete Boot Sale Diary Record(s) - DeleteBSDiary.jsp
Top
The trader needs to be able to delete one or more boot sale diary records from the database. The following scriptless JSP code shows how to do this :-
<%-- DeleteBSDiary.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="displayData" value="">
<c:set var="checked" value="">
<c:set var="errorMsg" value="">
<c:set var="thisPage" value="traderfacilities?${pageContext.request.queryString}">
<c:set var="bFormSuccess" value="false">
<c:set var="postMeth" value="POST">
<%-- Assign values to all the form controls coming in. These values will also
be used to dynamically re-populate the form for re-submission should an error occur. --%>
<c:set var="counter" value="${sessionScope.countbsdDates}">
<%-- Process the values if user submitted the form. --%>
<c:if test="${pageContext.request.method == 'POST'}">
<%-- Perform validation for required items. --%>
<c:forEach var="idx" begin="0" end="${counter}">
<c:choose>
<c:when test="${paramValues.dateKey[idx] != null}">
<c:set var="checked" value="${checked}${paramValues.dateKey[idx]},">
</c:when>
<c:otherwise>
</c:otherwise>
</c:choose>
</c:forEach>
<c:choose>
<c:when test="${checked == ''}">
<c:set var="error" value="<li>You must check at least one entry for deletion.</li>"/>
</c:when>
<c:otherwise>
<c:set var="error" value=""/>
</c:otherwise>
</c:choose>
<c:choose>
<c:when test="${!empty error}">
<%-- There are 1 or more error messages, so display them --%>
<c:set var="errorstart" value="<p>Please correct the following error(s) in order to properly submit the form</p><ul>">
<c:set var="errorMsg" value="${errorstart}${error}">
</c:when>
<c:when test="${empty error}">
<%-- No errors, store user entry for later use --%>
<c:set var="bFormSuccess" value="true">
<c:set var="displayData" value="<strong>Boot Sale Location Record(s) deleted: </strong>${checked}">
</c:when>
</c:choose>
</c:if>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Delete Boot Diary</title>
<style type="text/css">
.style5{margin-bottom: 0px;}
</style>
<body>
<%-- Keep showing the form until bFormSuccess is true --%>
<c:choose>
<c:when test="${!bFormSuccess}">
<form name='form1' action='${thisPage}' method='post'>
<p><strong>Check Boxes to Delete Boot Sale Diary Entries</strong></p>
<table style="width:500px;border:4;" class="style2">
<c:choose>
<c:when test="${counter ge 0}">
<tr><td><b>Check:</b></td><td><b>Boot Sale Date: </b></td><td><b>Boot Sale Name: </b></td></tr>
<c:forEach var="idx" begin="0" end="${counter}">
<tr><td valign="top"><input type="checkbox" name="dateKey" value="${sessionScope.bsdDates[idx]}"></td>
<td style="width: 150px" valign="top"><input type="text" name="name" value="${sessionScope.bsdDates[idx]}" readonly="readonly"></td>
<td style="width: 150px" valign="top"><input type="text" name="addr1" value="${sessionScope.bsdLocNames[idx]}" readonly="readonly"></td>
</c:forEach>
<tr><td> </td></tr><tr><td style="width: 124px; height: 45px;" valign="top"><input type="submit" name="Button1" type="button" value="Submit"> </td></tr>
</c:when>
<c:otherwise>
<c:set var="errorMsg" value="<p>There are no Diary Entries to delete!</p>">
</c:otherwise>
</c:choose>
<tr><td> </td></tr><tr><td colspan="5"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
<tr><td> </td></tr><tr><td style="color:red" colspan="3">${errorMsg}</td></tr>
</table>
</form>
</c:when>
<c:when test="${bFormSuccess}">
<jsp:forward page="/processinput" >
<jsp:param name="action" value="DeleteBSDiary">
<jsp:param name="date" value="${checked}">
</jsp:forward >
</c:when>
</c:choose>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as DeleteBSDiary.jsp
.
Delete Boot Sale Diary(S) Screenshots
The following screenshot shows what the Delete Boot Sale Diary(s) page looks like:-
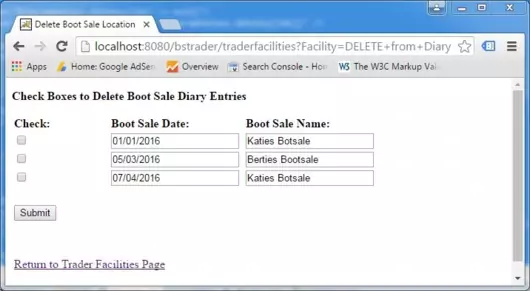
The following screenshot shows the Deletion of one boot sale diary:-
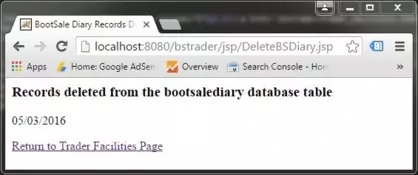
Inventory Report - InventoryReport.jsp
Top
The system should create an inventory report from available stock. The following scriptless JSP code shows how to do this :-
<%-- InventoryReport.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Inventory Report</title>
<body>
<form name='form1' method='get'>
<h2>Inventory Report off all Stock Items</h2>
<table style='width:60%;'>
<tr><td><b>Stock Item Name:</b></td><td><b>Stock Item Category:</b></td>
<td><b>Stock Item Description:</b></td><td><b>Price:</b></td>
<td><b>Stock Level:</b></td><td><b>Stock Reorder Level:</b></td>
<td><b>Sold Last Boot Sale:</b></td></tr>
<c:forEach var="idx" begin="0" end="${sessionScope.countsinames}">
<tr><td valign="top"><input style="width:200px;" type="text" name="name" value="${sessionScope.siStockName[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:150px;" type="text" name="cat" value="${sessionScope.siSelectCat[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:200px;" type="text" name="desc" value="${sessionScope.siStockDesc[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="price" value="${sessionScope.siStockPrice[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="silvl" value="${sessionScope.siStockLevel[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="sireorderlvl" value="${sessionScope.siStockReorderLevel[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="soldlast" value="${sessionScope.siStockSoldLastBootsale[idx]}" readonly="readonly"></td></tr>
</c:forEach>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
</table><br/>
</form>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as InventoryReport.jsp
.
Inventory Report Screenshot
The following screenshot shows what the Inventory Report page looks like:-
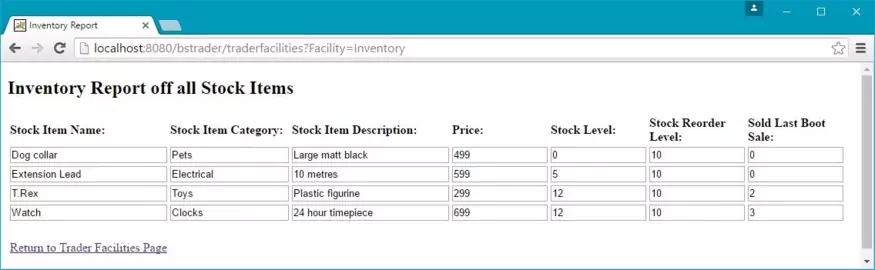
Stock Reorder Report - StockReorderReport.jsp
Top
The system should create a stock reorder report for stock items that are equal or less than a pre-specified stock level for that item. The following scriptless JSP code shows how to do this :-
<%-- StockReorderReport.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="counterSI" value="${sessionScope.countsinames}">
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Stock Reorder Report</title>
<style type="text/css">
.style5{margin-bottom:0px;}
</style>
<body>
<form name='form1' method='get'>
<h2>Stock Reorder Report</h2>
<table class="style1" style='width:28%;'>
<tr><td><b>Stock Item Name:</b></td><td><b>Stock Level:</b></td>
<td><b>Stock Reorder Level:</b></td></tr>
<c:forEach var="idx" begin="0" end="${countsinames}">
<c:if test="${sessionScope.siStockLevel[idx] le sessionScope.siStockReorderLevel[idx]}">
<tr><td valign="top"><input style="width:200px;" type="text" name="name" value="${sessionScope.siStockName[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="silvl" value="${sessionScope.siStockLevel[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="sireorderlvl" value="${sessionScope.siStockReorderLevel[idx]}" readonly="readonly"></td></tr>
</c:if>
</c:forEach>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
</table><br/>
</form>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as StockReorderReport.jsp
.
Stock Reorder Report Screenshot
The following screenshot shows what the Stock Reorder Report page looks like:-
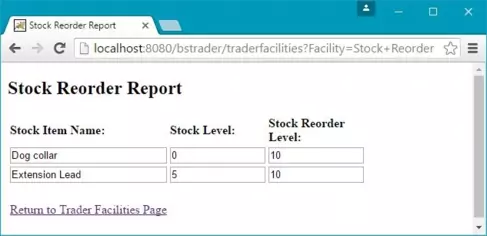
Sales Report - SalesReport.jsp
Top
The system should create a sales report from available stock showing stock sold at last boot sale. The following scriptless JSP code shows how to do this :-
<%-- SalesReport.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="counterSI" value="${sessionScope.countsinames}">
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Sales Report</title>
<style type="text/css">
.style5{margin-bottom: 0px;}
</style>
<body>
<form name='form1' method='get'>
<h2>Sales Report</h2>
<table style='width:19%;'>
<tr><td><b>Stock Item Name:</b></td><td><b>Sold Last Boot Sale:</b></td></tr>
<c:forEach var="idx" begin="0" end="${countsinames}">
<c:if test="${sessionScope.siStockSoldLastBootsale[idx] gt 0}">
<tr><td valign="top"><input style="width:200px;" type="text" name="name" value="${sessionScope.siStockName[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="silvl" value="${sessionScope.siStockSoldLastBootsale[idx]}" readonly="readonly"></td></tr>
</c:if>
</c:forEach>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
</table><br/>
</form>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as SalesReport.jsp
.
Sales Report Screenshot
The following screenshot shows what the Sales Report page looks like:-
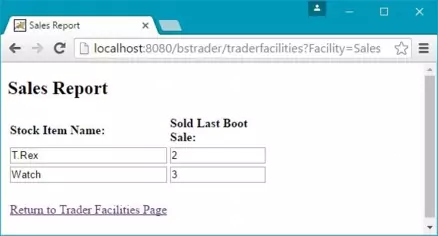
Out Of Stock Report - OutOfStockReport.jsp
Top
The system should create an out of stock report for stock with a level of zero. The following scriptless JSP code shows how to do this :-
<%-- OutOfStockReport.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="counterSI" value="${sessionScope.countsinames}">
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Out Of Stock Report</title>
<style type="text/css">
.style5{margin-bottom: 0px;}
</style>
<body>
<form name='form1' method='get'>
<h2>Out Of Stock Report</h2>
<table class="style1" style='width:28%;'>
<tr><td><b>Stock Item Name:</b></td><td><b>Stock Level:</b></td><td><b>Stock Reorder Level:</b></td></tr>
<c:forEach var="idx" begin="0" end="${countsinames}">
<c:if test="${sessionScope.siStockLevel[idx] eq 0}">
<tr><td valign="top"><input style="width:200px;" type="text" name="name" value="${sessionScope.siStockName[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="silvl" value="${sessionScope.siStockLevel[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:120px;" type="text" name="sireorderlvl" value="${sessionScope.siStockReorderLevel[idx]}" readonly="readonly"></td></tr>
</c:if>
</c:forEach>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
</table><br/>
</form>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as OutOfStockReport.jsp
.
Out of Stock Report Screenshot
The following screenshot shows what the Out of Stock Report page looks like:-
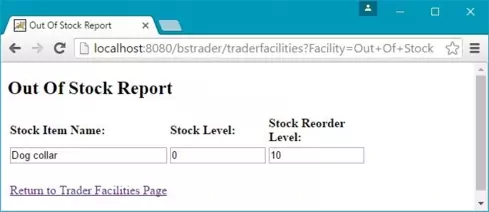
Customer Report - CustomerReport.jsp
Top
The system should create a customer report showing various information about bootsale locations and dates attended as well as stock information for e-mailing to customers. The following scriptless JSP code shows how to do this :-
<%-- CustomerReport.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Customer Feedback</title>
<style type="text/css">
.style1{background-color: orange;border:1px solid black;font-size: medium;}
.style2{border-style: solid;border-width: 1px;}
.style3{text-align: center;font-size: large;}
.style4{font-size: xx-large;}
.style5{background-color: orange;font-size: medium;}
.style6{font-size: medium;}
h2 {margin-top:0px;margin-bottom:10px;font-size:x-large;}
h3 {margin-top:0px;margin-bottom:10px;font-size:large;}
</style>
</head>
<body>
<div style="position:absolute;width:800px;height:112px;z-index: 1; left: 10px; top: 9px" id="layer1" class="style5">
<table class="style2">
<tr><td><img src="http://jsptutor.co.uk/images/carboot.jpg" width="150" height="100"></td>
<td style="width:800px;height:90px" class="style3"><span class="style4">Kevin's Boot Sales</span><br><br>
<em>'Everything You Need From The Boot of a Car'</em></td>
</tr>
</table>
</div>
<div style="position: absolute; width: 150px; height: 679px; z-index: 2; left: 13px; top: 128px" id="layer2">
<div style="position: absolute; width: 621px; height: 673px; z-index: 1; left: 152px; top: 2px" id="layer3">
<form name='form1' method='get'>
<h2>Contact Information:</h2>
<h3>Contact us by email at alf@jsptutor.co.uk</h3>
<h3>Visit Our Website: <a href="/bstrader/home.html">Kevin's Boot Sales</a></h3><br>
<c:if test="${countbsdDates ge 0}">
<h2>Our Diary:</h2>
<table style="border:4;">
<tr><td><b>Date:</b></td><td><b>Boot Sale Name:</b></td><td><b>Boot Sale Address:</b></td></tr>
<c:forEach var="idx" begin="0" end="${sessionScope.countbsdDates}">
<tr><td valign="top" rowspan="3"><input style="width: 110px" type="text" name="dates" value="${sessionScope.bsdDates[idx]}" readonly="readonly"></td>
<td valign="top" rowspan="3"><input style="width: 200px" type="text" name="name" value="${sessionScope.bsdLocNames[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width: 300px" type="text" name="addr1" value="${sessionScope.bsdLocAddr1[idx]}" readonly="readonly"></td></tr>
<tr><td><input style="width: 300px" type="text" name="addr2" value="${sessionScope.bsdLocAddr2[idx]}" readonly="readonly"></td></tr>
<tr><td><input style="width: 300px" type="text" name="addr3" value="${sessionScope.bsdLocAddr3[idx]}" readonly="readonly"></td></tr>
</c:forEach>
</table><br/>
</c:if>
<c:if test="${countbestsellers ge 0}">
<h2>Best Sellers:</h2>
<table style="border:4;">
<tr><td><b>Stock Item Name:</b></td><td><b>Stock Category:</b></td>
<td><b>Stock Description:</b></td><td><b>Price:</b></td></tr>
<c:forEach var="idx" begin="0" end="${countbestsellers}">
<tr><td><input style="width:200px;" type="text" name="siSoldName" value="${sessionScope.siSoldName[idx]}" readonly="readonly"></td>
<td><input style="width:160px;" type="text" name="siSoldCategory" value="${sessionScope.siSoldCategory[idx]}" readonly="readonly"></td>
<td><input style="width:160px;" type="text" name="siSoldDescription" value="${sessionScope.siSoldDescription[idx]}" readonly="readonly"></td>
<td><input style="width:80px;" type="text" name="siSoldPrice" value="${sessionScope.siSoldPrice[idx]}" readonly="readonly"></td></tr>
</c:forEach>
</table><br/>
</c:if>
<c:if test="${countlastinstock ge 0}">
<h2>Last Few Remaining:</h2>
<table style="border:4;">
<tr><td><b>Stock Item Name:</b></td><td><b>Stock Category:</b></td>
<td><b>Stock Description:</b></td><td><b>Price:</b></td></tr>
<c:forEach var="idx" begin="0" end="${countlastinstock}">
<tr><td valign="top"><input style="width:200px;" type="text" name="siLastInStockName" value="${sessionScope.siLastInStockName[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:160px;" type="text" name="siLastInStockCategory" value="${sessionScope.siLastInStockCategory[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:160px;" type="text" name="siLastInStockDescription" value="${sessionScope.siLastInStockDescription[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:80px;" type="text" name="siLastInStockPrice" value="${sessionScope.siLastInStockPrice[idx]}" readonly="readonly"></td></tr>
</c:forEach>
</table><br/>
</c:if>
<c:if test="${countother ge 0}">
<h2>Other Products:</h2>
<table style="border:4;">
<tr><td><b>Stock Item Name:</b></td><td><b>Stock Category:</b></td>
<td><b>Stock Description:</b></td><td><b>Price:</b></td></tr>
<c:forEach var="idx" begin="0" end="${countother}">
<tr><td valign="top"><input style="width:200px;" type="text" name="siLastInStockName" value="${sessionScope.siOPName[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:160px;" type="text" name="siLastInStockCategory" value="${sessionScope.siOPCategory[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:160px;" type="text" name="siLastInStockDescription" value="${sessionScope.siOPDescription[idx]}" readonly="readonly"></td>
<td valign="top"><input style="width:80px;" type="text" name="siLastInStockPrice" value="${sessionScope.siOPPrice[idx]}" readonly="readonly"></td></tr>
</c:forEach>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
</table>
</c:if>
</form>
</div>
</div>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as CustomerReport.jsp
.
Customer Report Screenshot
The following screenshot shows what the Customer Report page looks like:-
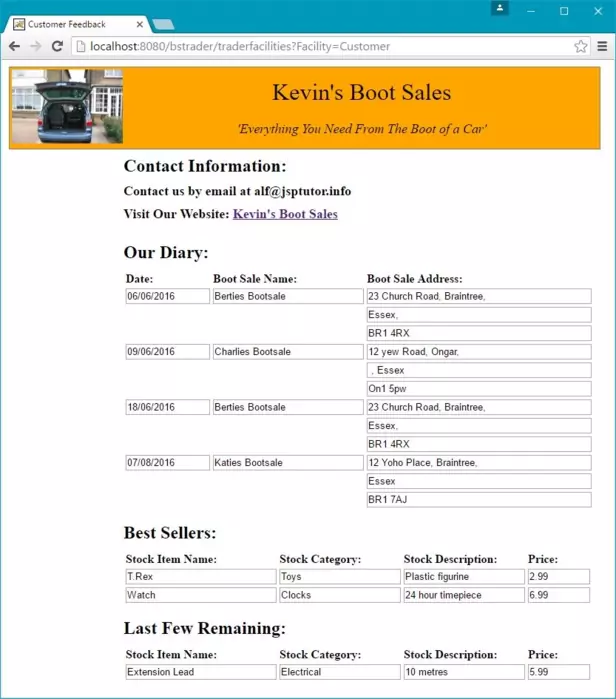
Mass Email - MassEmail.jsp
Top
The system should create a Mass Email page to be sent to all registered users. The following scriptless JSP code shows how to do this :-
<%-- MassEmail.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="displayData" value="">
<c:set var="errorMsg" value="">
<c:set var="thisPage" value="traderfacilities?${pageContext.request.queryString}">
<c:set var="bFormSuccess" value="false">
<c:set var="postMeth" value="POST">
<c:set var="counterRU" value="${sessionScope.countrunames}">
<%-- Assign values to all the form controls coming in. These values will also
be used to dynamically re-populate the form for re-submission should an error occur. --%>
<c:set var="email" value="${param.email}">
<c:set var="subject" value="Latest offers From Kevin's BootSale">
<c:set var="custReport" value="Customer Report to be done">
<c:choose>
<%-- Process the values if user submitted the form. --%>
<c:when test="${pageContext.request.method == 'POST'}">
<%-- Perform validation for required items. --%>
<c:choose>
<c:when test="${email == ''}">
<c:set var="noemail" value="<li>There must be at least one email address.</li>"/>
</c:when>
<c:otherwise>
<c:set var="noemail" value=""/>
</c:otherwise>
</c:choose>
<c:choose>
<c:when test="${custReport == ''}">
<c:set var="nocustReport" value="<li>Run Customer Report before Mass Email.</li>"/>
</c:when>
<c:otherwise>
<c:set var="nocustReport" value=""/>
</c:otherwise>
</c:choose>
<c:set var="error" value="${noemail}${nocustReport}">
<c:choose>
<c:when test="${!empty error}">
<%-- If there are 1 or more error messages, display them --%>
<c:set var="errorstart" value="<p>Please correct the following error(s) in order to properly submit the form</p><ul>">
<c:set var="errorMsg" value="${errorstart}${error}">
</c:when>
<c:when test="${empty error}">
<%-- No errors, store user entry for later use --%>
<c:set var="bFormSuccess" value="true">
<c:set var="succ1" value="<br><strong>Email Address: </strong>${email}">
<c:set var="succ2" value="<br><strong>Subject: </strong>${subject}">
<c:set var="succ3" value="<br><strong>Customer Report: </strong>${custReport}">
<c:set var="displayData" value="${succ1}${succ2}${succ3}">
</c:when>
</c:choose>
</c:when>
<c:when test="${sessionScope.subsequent != null}">
<%-- Populate form second time in. --%>
<c:set var="label" value="Mass Email"/>
<c:forEach var="idx" begin="0" end="${sessionScope.countrunames}">
<c:if test="${sessionScope.ruEmail[idx] ne ''}">
<c:set var="email" value="${email}${sessionScope.ruEmail[idx]},">
</c:if>
</c:forEach>
</c:when>
</c:choose>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Mass Email To All Registered Customers</title>
</head>
<body>
<%-- Keep showing the form until bFormSuccess is true --%>
<c:choose>
<c:when test="${!bFormSuccess}">
<form name='form1' action='${thisPage}' method='post'>
<p><strong>Mass Email To All Registered Customers:</strong></p>
<table style="width:100%;">
<tr><td style="width: 119px" valign="top">Email Addresses:<br></td><td><input type="text" name="email" value="${email}" size="30"/></td></tr>
<tr><td style="width: 119px" valign="top">Subject:<br></td><td><input type="text" name="subject" value="${subject}" size="30" readonly="readonly"/></td></tr>
<tr><td style="width: 119px" valign="top">Customer Report:<br></td><td><input type="text" name="custReport" value="${custReport}" size="30" readonly="readonly"/></td></tr>
<tr><td> </td></tr><tr><td style="width: 124px; height: 45px;" valign="top"><input type="submit" name="Button1" type="button" value="Submit"> </td></tr>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
<tr><td> </td></tr><tr><td style="color:red" colspan="2">${errorMsg}</td></tr>
</table>
</form>
</c:when>
<c:when test="${bFormSuccess}">
<jsp:forward page="/sendemails" >
<jsp:param name="email" value="${email}">
<jsp:param name="subject" value="${subject}">
<jsp:param name="custReport" value="${custReport}">
<jsp:param name="displayData" value="${displayData}">
</jsp:forward >
</c:when>
</c:choose>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as MassEmail.jsp
.
Mass Email Screenshot
The following screenshot shows what the Mass Email page looks like:-
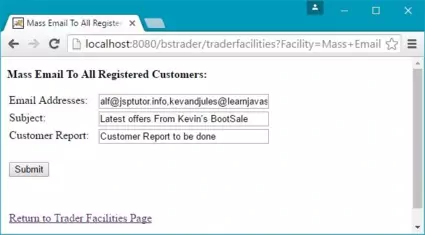
Mass Email Result - Result.jsp
Top
The system should report on the success of mailing to registered users. The following scriptless JSP code shows how to do this :-
<%-- Result.jsp --%>
<%@ page language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<c:set var="email" value="${param.email}">
<c:set var="emailmessage" value="${requestScope.emailmessage}">
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Mass Email Confirmation</title>
</head>
<body>
<p><strong>Mass Email Confirmation</strong></p>
<table style="width:100%;">
<tr><td style="width: 119px" valign="top">Email Recipients:<br></td><td><input type="text" name="email" value="${email}" size="100" readonly="readonly"></td></tr>
<tr><td style="width: 119px" valign="top">Email Message:<br></td><td><input type="text" name="emailmessage" value="${emailmessage}" size="100" readonly="readonly"></td></tr>
<tr><td> </td></tr><tr><td colspan="2"><a href='/bstrader/trader_facilities.html'>Return to Trader Facilities Page</a></td></tr>
</table>
</body>
</html>
Cut and paste the above code into your text editor and save it in the c:\_ServletsJSP_Case_Study\bstrader\view\jsp directory as Result.jsp
.
Lesson 6 Complete
In this lesson we took a second look at the view part of the MVC paradigm and coded the second part of the view components for traders.
What's Next?
In the next lesson we take a final look at the view part of the MVC paradigm and code the view components for the visitor.