.delay()
S2C Home « Effects « .delay()
Function queues.
Description
The .dequeue()
method is used to delay execution of subsequent items in the queue using a duration timer.
- Only subsequent events in a queue are delayed. As an example the no-arguments forms of
.show()
or.hide()
, which do not use the effects queue, will not be delayed. - The default queue is named 'fx' and has certain properties that are not shared with named queues:
- The 'fx' queue will automatically
.dequeue()
the next function on the queue and run it if the queue hasn't started. - When
.dequeue()
is used on a function from the 'fx' queue, it willunshift()
the string 'inprogress', into the [0] location of the array, thus flagging that the 'fx' queue is currently executing. - Being the default, the 'fx' queue is called by
.animate()
and any other function that calls it by default.
- The 'fx' queue will automatically
Syntax
Signature | Description |
---|---|
.delay( duration [, queueName] ) | Delay execution of subsequent items in the queue using a duration timer, optionally using a queue name to identify the queue. |
Parameters
Parameter | Description | Type |
---|---|---|
duration | A string or number determining the time the animation will run for.
| Number |
queueName | A string containing the name of the queue. Defaults to 'fx', which is the standard effects queue. | String |
Return
A jQuery
object.
.delay( duration [, queueName] )
ExamplesTop
Delay execution of subsequent items in the queue using a duration timer, optionally using a queue name to identify the queue.
In the example below when the button is pressed we animate the images below with a delay on the first image.
$(function(){
$('#btn7').on('click', function() {
$('#beef').slideToggle(1000)
.slideToggle("fast")
.delay(1000)
.slideToggle(1000)
.slideToggle("fast");
$('#fish').slideToggle(1000)
.slideToggle("fast")
.slideToggle(1000)
.slideToggle("fast");
});
});
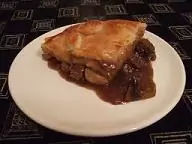
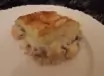
In the example below when the button is pressed we toggle the image below. We then use a delay on the 'pastries' queue before retoggling. As this is not the default 'fx' queue, it needs dequeueing to show the image again, see the description above.
$(function(){
$('#btn8').on('click', function() {
$('#almond').slideToggle("fast")
.delay(1000, 'pastries')
.queue('pastries', function(next) {
$(this).slideToggle(1000);
next();
})
.dequeue('pastries');
});
});
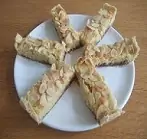
Related Tutorials
jQuery Intermediate Tutorials - Lesson 8 - Controlling Effects