.attr()
S2C Home « Attributes & Properties « .attr()
First element attribute retrieval and matched set attribute setting.
Description
The .attr()
method is used to retrieve an attribute value from the first element within the matched set, or set one or more attribute values within the matched set.
- Returns
undefined
for attribute values that haven't been set. - Do not use the
.attr()
method on plain objects, arrays, the document or window. - Use the
.attr()
method to retrieve or set DOM attributes. - When changing the dynamic state of a DOM element, the
.prop()
method should be used to setdisabled
andchecked
. - When changing the dynamic state of a DOM element, the
.val()
method should be used for getting and setting value. - You cannot change the
type
attribute for the <input
> or <button
> HTML tags in versions 6, 7 and 8 of Internet Explorer.
For this reason jQuery does not allow modification of thetype
attribute and will throw anError
in all browsers.
Syntax
Signature | Description |
---|---|
.attr( attributeName ) | Retrieve an attribute value from the first element within the matched set. |
.attr( attributeName, value ) | Set a single attribute value within the matched set. |
.attr( map ) | Use a map of attribute-value pairs to set multiple attribute values within the matched set. |
.attr( attributeName, function(index, attr) ) | A function returning the attribute value to set. |
Parameters
Parameter | Description | Type |
---|---|---|
attributeName | The attribute name. | String |
value | A value to set the attribute to. | String orNumber |
attributes | A plain JavaScript object of key-value pairs to set. | PlainObject |
function(index, attr) | A function.
|
Function |
Return
Retrieving - A String
object.
Setting - A jQuery
object including the updated attributes within the matched set.
.attr( attributeName )
ExampleTop
Retrieve an attribute value from the first element within the matched set.
In the example below when we press the button we get the value of the class attribute for the table below that has an id of 'table1'.
Table Row 1, Table Data 1 | Table Row 1, Table Data 2 |
Table Row 2, Table Data 1 | Table Row 2, Table Data 2 |
$(function(){
$('#btn1').on('click', function() {
alert('This table element has the following classes: '
+ $('#table1').attr('class') + '.');
});
});
.attr( attributeName, value )
ExamplesTop
Set a single attribute value within the matched set.
In the example below when we press the button we select said button and change its value.
$(function(){
$('#btn2').on('click', function() {
$('#btn2').attr('value', 'Button value changed');
});
});
.attr( attributes )
ExamplesTop
Use a plain JavaScript object of attribute-value pairs to set multiple attribute values within the matched set.
We can set multiple attributes using a 'map' of attribute-value pairs which we pass to the method in object literal format, commonly known as JSON. Take a look at: JavaScript Intermediate - Lesson 7: Object Literals for more information on object literals and JSON.
- The quotes around attribute names are optional when setting multiple attributes, apart from when setting the 'class' attribute. The 'class' attribute must always be surrounded in quotes, as it is a JavaScript reserved word and will be treated as such if not quoted.
In the example below when we press the button, we modify the 'width' and 'height' attributes and add a 'title' attribute to the set of attributes attached to the image. The original HTML for the image is shown below:
<img src="../images/thaigreencurrysmall.jpeg"
alt="a picture of curry" width="200" height="150">
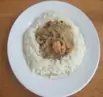
$(function(){
$('#btn3').on('click', function() {
$('#curry').attr({
title: 'Thai Green Curry',
width: '300',
height: '200'
});
});
});
.attr( attributeName, function(index, attr) )
ExampleTop
A function returning the attribute value to set.
In the example below when we press the button we add a 'title' attribute to each image.
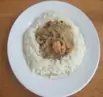
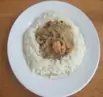
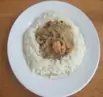
$(function(){
$('#btn4').on('click', function() {
$('.curry').attr('title', function(index, attr) {
return 'Thai Green Curry: ' + index;
});
});
});
Related Tutorials
jQuery Basic Tutorials - Lesson 9 - Working With CSS Attributes