.offset()
S2C Home « Attributes & Properties « .offset()
First element current coordinates retrieval and matched set current coordinates setting.
Description
The .offset()
method is used to retrieve the current coordinates from the first element within the matched set relative to the document, or set the current coordinates within the
matched set relative to the document.
- Use the
.position()
method if you wish to retrieve the current coordinates relative to the offset parent, rather than relative to the document. - You cannot use the
.offset()
method on hidden elements and does not account for margins, padding or borders set on the body element. - When setting coordinates using the
.offset()
method and the element's css position property is currently set tostatic
, it will be set torelative
to allow for this repositioning.
Syntax
Signature | Description |
---|---|
.offset() | Retrieve the current coordinates from the first element within the matched set, relative to the document. |
.offset( coordinates ) | Set the current coordinates within the matched set, relative to the document. |
.offset( function(index, coordinates ) | A function returning the coordinates to set. |
Parameters
Parameter | Description | Type |
---|---|---|
coordinates | A plain JavScript object containing the properties top and left, which are integers indicating the top and left coordinates to set for the elements. | PlainObject |
function(index, coordinates) | A function.
|
Function |
Return
Retrieving - An Object
object containing the properties top and left.
Setting - A jQuery
object including the updated properties within the matched set.
.offset()
ExampleTop
Retrieve the current coordinates from the first element within the matched set, relative to the document.
In the example below we alert the offset of the following image with the id of 'curry'.
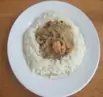
$(function(){
$('#btn22').on('click', function() {
alert('The offset coordinates of the of the above image are: '
+ JSON.stringify($('#curry').offset()) + '.');
});
});
.offset( coordinates )
ExampleTop
Set the current coordinates within the matched set, relative to the document.
In the example below we first get the offset of the image below with the id of 'curry2'. We then add 20 pixels to the 'top' and 'left' properties and reposition the images offset.
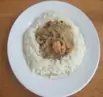
$(function(){
$('#btn23').one('click', function() {
var coords = $('#curry2').offset();
coords.top +=20;
coords.left +=20;
$('#curry2').offset(coords);
});
});
.offset( function (index, coordinates) )
ExampleTop
A function returning the coordinates to set.
In the example below when we press the button we change the offset of the image with an index of 1.
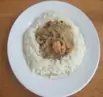
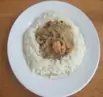
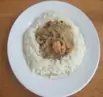
$(function(){
$('#btn24').on('click', function() {
$('#main .curry').offset( function (index, coordinates) {
if (index == 1) {
coordinates.top +=20;
coordinates.left +=20;
}
return $(this).offset(coordinates);
});
});
});
Related Tutorials
jQuery Basic Tutorials - Lesson 11 - Working With Dimension & Position CSS Properties