Quiz SummaryS2C Home « Quiz Summary
In this lesson we summarize the HTML, CSS, JavaScript and jQuery used for our case study.
File Structure
The following diagram shows the file structure used for the interactive quiz.
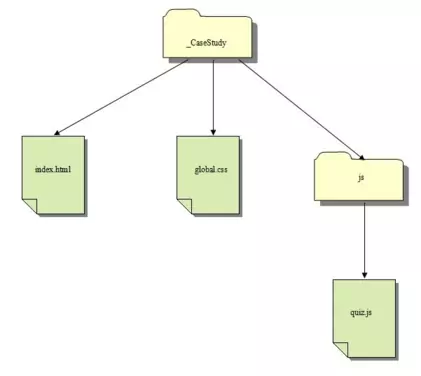
Our Interactive Quiz HTML
Following is the index.html file which contains the complete HTML for our interactive quiz.
<!DOCTYPE html>
<!-- Our HTML/CSS for the HTML skeleton follows -->
<html lang="en">
<head>
<title>JavaScript/jQuery Case Study - Interactive Quiz</title>
<meta name="Description" content="Creating an interactive quiz at https://server2client.com/">
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link href="global.css" rel="stylesheet" type="text/css">
<script src="js/jquery-3.5.1.min.js" type="text/javascript"></script>
<script src="js/quiz.js" type="text/javascript"></script>
</head>
<body>
<div id="main">
<h1>Interactive Quiz</h1>
<div class="questionContainer" style="display: block;">
<div class="question"><strong>Question 1 :</strong> What goes inside a class defintion?</div>
<div class="answers">
<ul><li><label><input type="radio" id="q1-a" name="q1"> Functions</label></li>
<li><label><input type="radio" id="q1-b" name="q1"> Subroutines</label></li>
<li><label><input type="radio" id="q1-c" name="q1"> Methods</label></li>
<li><label><input type="radio" id="q1-d" name="q1"> Procedures</label></li></ul>
</div>
<div class="hide red" id="exp1"> - Methods go inside a class definition.</div>
<div class="btnContainer">
<div class="prev"></div>
<div class="next"><a class="btnNext">Next ++</a></div>
<div class="clear"></div>
</div>
</div>
<div class="questionContainer hide">
<div class="question"><strong>Question 2 :</strong> java
command compiles?</div>
<div class="answers">
<ul><li><label><input type="radio" id="q2-a" name="q2"> true</label></li>
<li><label><input type="radio" id="q2-b" name="q2"> false</label></li></ul>
</div>
<div class="hide red" id="exp2"> - We use the javac command to compile programs.</div>
<div class="btnContainer">
<div class="prev"><a class="btnPrev">-- Prev</a></div>
<div class="next"><a class="btnNext">Next ++</a></div>
<div class="clear"></div>
</div>
</div>
<div class="questionContainer hide">
<div class="question"><strong>Question 3 :</strong> A statement contains methods?</div>
<div class="answers">
<ul><li><label><input type="radio" id="q3-a" name="q3"> true</label></li>
<li><label><input type="radio" id="q3-b" name="q3"> false</label></li></ul>
</div>
<div class="hide red" id="exp3"> - A method contains statements.</div>
<div class="btnContainer">
<div class="prev"><a class="btnPrev">-- Prev</a></div>
<div class="next"><a class="btnShowResult">Finish</a></div>
<div class="clear"></div>
</div>
</div>
<div class="txtStatusBar">Quiz Progress Bar
<span class="errMsg hide"><strong>Please select an answer</strong></span></div>
<div id="progressContainer" style="display: block;">
<div id="progress"></div>
</div>
<div class="hide" id="resultContainer"></div>
</div>
</body>
</html>
Our Interactive Quiz CSS
Following is the global.css file which contains all the CSS for our interactive quiz.
/* Reset browser styles */
h1, h2, p, div, ul, ol, li, code, pre, html, body {
margin: 0;
padding: 0;
font-size: 100%;
font-weight: normal;
}
.txtStatusBar {
font-weight:bold;
margin:5px 0px;
}
/* wrap main content */
#main {
background-color: silver;
border:1px solid black;
float:left;
margin: 10px;
padding: 10px;
position: relative;
width: 730px;
}
/* Quiz heading */
h1 {
font-size: 200%;
font-weight:bold;
text-align: center;
}
/* Question container */
.questionContainer {
background:white;
border:3px double black;
margin:10px 0px;
padding:3px;
width:720px;
}
/* Button container */
.btnContainer {
margin:10px 0 10px 1%;
width:98%;
}
.prev{float:left;}
.prev a, .next a {
background:none repeat scroll 0 0 white;
border:1px solid #000000;
cursor:pointer;
font-size:10px;
font-weight:bold;
padding:2px 5px;
}
.prev a:hover, .next a:hover {
background:none repeat scroll 0 0 #36648B;
color:white !important;
text-decoration:none !important;
}
.next, .btnShowResult {float:right;}
.clear {clear:both;}
/* Progress bar */
.txtStatusBar {
font-weight:bold;
margin:5px 0px;
}
#progressContainer {
background:white;
border:3px double black;
height:25px;
margin:0px;
padding:3px;
width:720px;
}
#progress {
background:none repeat scroll 0 0 #36648B;
height:25px;
width:0;
}
/* Refined layout CSS */
.hide {display:none;}
.answers ul li {list-style:none outside none;}
.clear{clear:both;}
input {
position:relative;
top:2px;
}
#resultContainer {
background:white;
border:3px double black;
margin:10px 0px;
padding:3px;
width:720px;
}
#resultContainer div {line-height:20px;}
/* Error message */
.errMsg {
color:red;
margin:5px 10px;
}
/* Question explanation and score highlight */
.red {color:red;}
.totalScore{font-weight:bold;}
Our Interactive Quiz JavaScript File
Following is the quiz.js file which contains all the JavaScript and jQuery for our interactive quiz.
// JavaScript and jQuery for interactive quiz follows
$(function(){
// highlight question row on mouseover
$('.answers label').css({display:'block',width:'712'});
$('.answers label').mouseover(function(){$(this).css({backgroundColor:'yellow'});});
$('.answers label').mouseleave(function(){$(this).css({backgroundColor:'#fff'});});
var S2CQuiz = {
init: function(){
// next question
$('.btnNext').on('click', function(){
// Make sure a radio box has been checked
if ($('input[type=radio]:checked:visible').length == 0) {
$('.errMsg').show().fadeOut(1200);
return false;
}
$(this).parents('.questionContainer').fadeOut(500, function(){
$(this).next().fadeIn(500);
});
var progBar = $('#progress');
var numq = S2CQuiz.numQuestions();
var progWidth = $('#progressContainer').width() / numq;
progBar.width(progBar.width() + progWidth + 'px');
});
// previous question
$('.btnPrev').on('click', function(){
$(this).parents('.questionContainer').fadeOut(500, function(){
$(this).prev().fadeIn(500);
});
var progBar = $('#progress');
// Extract question number and calculate width
var qString = $(this).parents('.questionContainer').children()
.first().children('strong').text();
var numq = parseInt(qString.substr(9, 2));
numq--;
var progWidth = progBar.width() / numq;
progBar.width(progBar.width() - progWidth + 'px');
});
// last question
$('.btnShowResult').on('click', function(){
// Make sure a radio box has been checked
if ($('input[type=radio]:checked:visible').length == 0) {
$('.errMsg').show().fadeOut(1200);
return false;
}
$('#progress').width(0);
$('#progressContainer').hide();
$('.txtStatusBar').text('Quiz Result');
// Extract users answers to an array and call a function to check them
var arr = $('input[type=radio]:checked');
var ans = S2CQuiz.userAnswers = [];
for (var i = 0; i < arr.length; i++) {
ans.push(arr[i].getAttribute('id'));
}
var results = S2CQuiz.checkAnswers();
// Loop through the 'right' or 'wrong' array extracting the results
var resultSet = '';
var rightCount = 0;
for (var i = 0; i < results.length; i++){
if (results[i] == "right") {
rightCount++;
resultSet += '<div> Question ' + (i + 1) + ' is ' + results[i] + '</div>';
} else {
var expid = '#' + 'exp' + (i + 1);
var exp = $(expid).text();
resultSet += '<div class="red"> Question ' + (i + 1) + ' is ' + results[i]
+ '<strong>' + exp + '</strong></div>';
}
}
var numq = S2CQuiz.numQuestions();
resultSet += '<div class="totalScore">You scored ' + rightCount + '/' + numq + '</div>';
$('#resultContainer').html(resultSet).show();
});
},
answers: { q1: 'c', q2: 'b', q3: 'b' },
// Check answers and output 'right' or 'wrong' to another array
checkAnswers: function() {
var arr = this.answers;
var ans = this.userAnswers;
var resultArr = [];
for (var p in ans) {
var x = parseInt(p) + 1;
var key = 'q' + x;
var flag = "wrong";
if (ans[p] == 'q' + x + '-' + arr[key]) {
flag = "right";
}
else {
flag = "wrong";
}
resultArr.push(flag);
}
return resultArr;
},
// Calcualte number of question
numQuestions: function() {
var arr = this.answers;
var x = 0;
for (var p in arr) {
if (p < 10) {
x = parseInt(p.substr(1, 1));
} else {
x = parseInt(p.substr(1, 2));
}
}
return x;
}
};
S2CQuiz.init();
$('.answers label').css({display:'block',width:'100%'});
$('.answers label').mouseover(function(){$(this).css({backgroundColor:'#36648B'});});
$('.answers label').mouseover(function(){$(this).css({color:'white'});});
$('.answers label').mouseleave(function(){$(this).css({backgroundColor:'#fff'});});
$('.answers label').mouseleave(function(){$(this).css({color:'#000'});});
});
Lesson 7 Complete
In this lesson we summarized the code for the interactive quiz.
Related Tutorials
HTML Basic Tutorials - Lesson 2 - Basic HTML Structure
HTML Intermediate Tutorials - Lesson 2 - HTML Structure - Layout
HTML Intermediate Tutorials - Lesson 1 - Metadata
HTML Advanced Tutorials - Lesson 1 - Scripting
CSS Basic Tutorials - Lesson 8 - Padding & Margins
CSS Basic Tutorials - Lesson 9 - Borders
CSS Intermediate Tutorials - Lesson 1 - Backgrounds
CSS Intermediate Tutorials - Lesson 2 - A Final Look At The Box Model
CSS Intermediate Tutorials - Lesson 7 - Positioning
CSS Advanced Tutorials - Lesson 6 - Layout
JavaScript Basic Tutorials - Lesson 5 - Variables
JavaScript Intermediate Tutorials - Lesson 1 - Arrays
JavaScript Intermediate Tutorials - Lesson 3 - Conditional Statements
jQuery Basic Tutorials - Lesson 6 - Tree Traversal
jQuery Basic Tutorials - Lesson 9 - Working With CSS Attributes
jQuery Basic Tutorials - Lesson 11 - Working With Dimension & Position CSS Properties
jQuery Intermediate Tutorials - Lesson 2 - DOM Insertion, Inside
jQuery Intermediate Tutorials - Lesson 6 - Fading & Sliding Effects
jQuery Intermediate Tutorials - Lesson 7 - Basic & Custom Effects
jQuery Advanced Tutorials - Lesson 1 - Browser & Loading Events
jQuery Advanced Tutorials - Lesson 4 - Event Handler Attachments
What's Next?
That's it for the JavaScript and jQuery case study, hope you learned from it.