Model Part 1 - Stock
InterfaceS2C Home « Model Part 1 - Stock
Interface
In our second lesson of the Model Part 1 section we code up the Stock
interface.
Compiling The Stock
Interface Top
The following Stock
interface will be used in other projects and so must be implemented as is. The interface provides the contract for the CRUD
operations for the Manufacturer file as
well as templates for the search and locking/unlocking mechanisms.
Stocking Goods Limited have also included doc comments for the interface which are written in HTML and can be turned into a document at a later date using the javadoc tool. Stocking Goods Limited have also requested that we provide doc comments for all class, field, constructor and method declarations.
Cut and paste the following code into your text editor and save it in the c:\_Case_Study\src\model directory.
package model;
/**
* An interface implemented by classes that provide use of the Manufacturer file.
*
*/
public interface Stock {
/**
* Creates a new record in the Manufacturer file which could be a deleted
* entry. Inserts the given Manufacturer data, and returns the record
* number of the new record.
*
* @param manufacturerData An array of Manufacturer data fields.
*
* @return The record number of the Manufacturer record created.
* @throws DuplicateKeyException Record already exists.
*/
public long createRecord(String[] manufacturerData) throws DuplicateKeyException;
/**
* Reads a record from the Manufacturer file and returns a String
* array where each element is a Manufacturer field value.
*
* @param recNo A record number denoting the byte location in the
* Stock file where this Manufacturer record starts.
*
* @return A String
array containing the fields of the
* Manufacturer record.
*
* @throws RecordNotFoundException Indicates the record was not found.
*/
public String[] readRecord(long recNo) throws RecordNotFoundException;
/**
* Modifies the fields of a Manufacturer record. The new value for field n
* appears in manufacturerData[n]. Throws a SecurityException if the record
* is locked with a number other than lockRecord.
*
* @param recNo The record number of the product record to read.
* @param manufacturerData A String
array of Manufacturer fields.
* @param lockRecord The lock value.
*
* @throws RecordNotFoundException Indicates the record was not found.
* @throws SecurityException Indicates a lock record mismatch.
*/
public void updateRecord(long recNo, String[] manufacturerData, long lockRecord)
throws RecordNotFoundException, SecurityException;
/**
* Deletes a record, making the record number and associated disk storage
* available for reuse. Throws SecurityException if the record is locked
* with a number other than lockRecord.
*
* @param recNo The record number of the product record to read.
* @param lockRecord The lock value.
*
* @throws RecordNotFoundException Indicates the record was not found.
* @throws SecurityException Indicates a lock record mismatch.
*/
public void deleteRecord(long recNo, long lockRecord)
throws RecordNotFoundException, SecurityException;
/**
* Returns an array of record numbers that match the specified search. Field n
* in the Manufacturer file is described by searchCriteria[n]. A null value in
* searchCriteria[n] matches any field value. A non-null value in
* searchCriteria[n] matches any field value that begins with searchCriteria[n].
*
* @param searchCriteria The search criteria for retrieving records.
*
* @return A long
array of manufacturer record numbers
* matching the search criteria.
*/
public long[] findBySearchCriteria(String[] searchCriteria);
/**
* Locks a record so that it can only be updated or deleted by this client.
* Returned value is a number that must be used when the record is unlocked,
* updated, or deleted. If the specified record is already locked by a
* different client, the current thread goes into a wait state until the
* record is unlocked.
*
* @param recNo The record number of the Manufacturer record to lock.
*
* @return The record number of the locked Manufacturer record.
* @throws RecordNotFoundException Indicates the record was not found.
*/
public long lockRecord(long recNo) throws RecordNotFoundException;
/** Releases the lock on a record. Lock number must be the same as the number
* returned when the record was locked; otherwise throws SecurityException.
*
* @param recNo The record number of the Manufacturer record to unlock.
*
* @return A long
value denoting the generated lock number.
*
* @throws SecurityException Indicates a lock record mismatch.
*/
public void unlock(long recNo, long lockRecord) throws SecurityException;
}
Compiling Our Source File With the -d
Option
Open your command line editor:
Change to directory cd c:\_Case_Study\src\model
Compile Stock.java
using the java compiler with the -d
option
javac -d ..\..\classes Stock.java
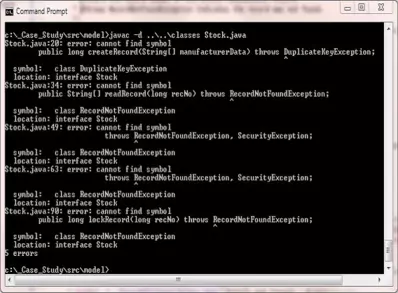
As you can see from the screenshot we get a compiler error because the compiler can't find the exception classes we just compiled. This is because we are now putting our compiled classes into separate folders
from our source using the -d
option. To find them we need to use another compiler option to point to the directories the class files reside in.
Compiling Our Source File With the -classpath
Option
When compiling our source files we have the option of pointing the compiler to directories where other compiled classes are, that we need to cleanly compile the class in question. There are two options available
to do this, these being -classpath
and the abbreviated version -cp
. In this example we will use -classpath
and for future compiles we will use -cp
.
Open your command line editor:
Change to directory cd c:\_Case_Study\src\model
Compile Stock.java
using the java compiler with the -classpath
and -d
options
javac -classpath ..\..\classes -d ..\..\classes Stock.java
The following screenshot shows that we now get a clean compile and also the classes now compiled into the classes\model
directory.
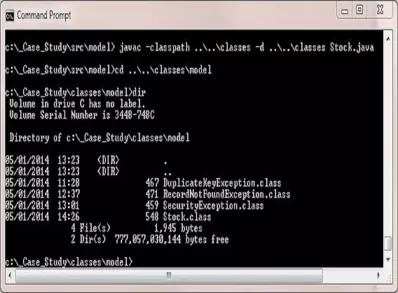
Lesson 4 Complete
In this lesson we coded Stock
Interface.
Related Java Tutorials
Beginning Java - Primitive Variables
Beginning Java - Conditional Statements
Beginning Java - Loop Statements
Objects & Classes - Class Structure and Syntax
Objects & Classes - Reference Variables
Objects & Classes - Methods
Objects & Classes - Instance Variables & Scope
Objects & Classes - Constructors
Objects & Classes - Static Members
OO Concepts - Encapsulation - Getters & Setters
OO Concepts - Inheritance Concepts - Using the super
keyword
OO Concepts - Interfaces
OO Concepts - The Object
Superclass - Checking Object Equality
OO Concepts - The Object
Superclass - The hashCode()
Method
OO Concepts - The Object
Superclass - The toString()
Method
Exceptions - Handling Exceptions
Exceptions - Declaring Exceptions
Exceptions - Creating Our Own Exceptions
API Contents - Inheritance - Using the package
keyword
API Contents - Inheritance - Using the import
keyword
Concurrency - Synchronization - Synchronized Blocks
Swing - RMI - Serialization
What's Next?
In the next lesson we code the manufacturer request class.