Model Part 1 - Manufacturer RequestS2C Home « Model Part 1 - Manufacturer Request
In our third lesson of the Model Part 1 section we look at the stakeholder request for the creation of a class representing the record information within the Manufacturer file. This will be called unsurprisingly Manufacturer
as the stakeholder sees a lot of future reuse of this file and it will also be helpful when implementing the Stock
interface in the next lesson.
Manufacturer Request Top
In this lesson we create the Manufacturer
class, wherein a Manufacturer
object is a representation of the Manufacturer file in an object oriented framework, allowing easy access to fields.
We will be creating a log file using the java.util.Logger
class which allows us to put messages into a file that we can view after a run of the application to help with debugging and to store information about events that have occurred and methods that have been invoked. We can also set the granularity of log messages when we need finer or less detailed information to appear in our log files. We will create log files in most of our other top level classes so that we can see the general flow through our coding if problems arise.
Access to the methods of the Manufacturer
class can be invoked remotely and so this object can be serialized, therefore we use a version number so that serialisation can occur without worrying about the underlying class changing between serialisation and deserialisation.
We will use statics for the length of each field within the Manufacturer file and private instance variables that can be accessed by public getters and setters.
A default no arguments constructor will be coded as well as a constructor to set initial values for a Manufacturer
object.
We will also override the equals()
, hashCode()
and
toString()
methods of the Object
class.
Another point of interest not covered elsewhere on the site are the annotation used for the Logger
, @SuppressWarnings("unused")
which supresses compiler warnings for a field not being
used and the Object
class overrides using @Override
which ensures we override correctly and informs browsers of the code that this
is an override. Annotations were introduced in java and are a form of metadata that provide data about a program but are not part of the program itself and have no direct effect on the operation of the code annotated.
Compiling The Manufacturer
Class Top
Cut and paste the following code into your text editor and save it in the c:\_Case_Study\src\model directory.
package model;
import java.util.logging.Logger;
/**
* A Manufacturer object is a representation of the Manufacturer file
* in an object oriented framework, allowing easy access to fields.
*
* @author Charlie
* @version 1.0
* @see model.StockImpl
*
*/
public class Manufacturer {
/**
* The Logger instance through which all log messages from this class are routed.
* Logger namespace is s2cCaseStudy
.
*/
private static Logger log = Logger.getLogger("s2cCaseStudy"); // Log output
/**
* A version number for the Manufacturer class so that serialisation
* can occur without worrying about the underlying class changing
* between serialisation and deserialisation.
*/
@SuppressWarnings("unused")
private static final long serialVersionUID = 2498052502L;
/* Declared constants for lengths of each field in the Manufacturer record. */
/**
* Length of Manufacturer deletedFlag field.
*/
static final int DELETED_FLAG_LENGTH = 1;
/**
* Length of Manufacturer name field.
*/
static final int NAME_LENGTH = 30;
/**
* Length of Manufacturer location field.
*/
static final int LOCATION_LENGTH = 30;
/**
* Length of Manufacturer Product field.
*/
static final int PRODUCT_LENGTH = 40;
/**
* Length of Manufacturer price field.
*/
static final int PRICE_LENGTH = 8;
/**
* Length of Manufacturer stockLevel field.
*/
static final int STOCK_LEVEL_LENGTH = 3;
/**
* Length of Manufacturer stockOrdered field.
*/
static final int STOCK_ORDERED_LENGTH = 3;
/**
* Length of a complete Manufacturer data record, calculated
* by adding all the fields together.
*/
public static final int MANUFACTURER_RECORD_LENGTH = DELETED_FLAG_LENGTH
+ NAME_LENGTH
+ LOCATION_LENGTH
+ PRODUCT_LENGTH
+ PRICE_LENGTH
+ STOCK_LEVEL_LENGTH
+ STOCK_ORDERED_LENGTH;
/* Instance variables. */
/**
* Stores the Deleted flag.
*/
private String deletedFlag = ""; // The deleted flag indicator.
/**
* Stores the name of the Manufacturer
*/
private String name = ""; // Manufacturer Name.
/**
* Stores the City Location of the Manufacturer.
*/
private String location = ""; // Manufacturer Location.
/**
* Stores the product name.
*/
private String product = ""; // Product name.
/**
* Stores the unit price of the product.
*/
private String price = ""; // Product unit price.
/**
* Stores the amount of stock available.
*/
private String stockLevel = ""; // Product available from manufacturer.
/**
* Stores the amount of stock ordered.
*/
private String stockOrdered = ""; // Amount of product ordered.
/* Manufacturer constructors. */
/**
* Create an instance of Constructor with default values.
*/
public Manufacturer() {
log.entering("Manufacturer", "Manufacturer");
log.exiting("Manufacturer", "Manufacturer");
}
/**
* Create an instance of Constructor with a list
* of initial values.
*
* @param deletedFlag The Deleted flag value.
* @param name The name of the Manufacturer.
* @param location The City Location of the Manufacturer.
* @param product Product name.
* @param price Product unit price.
* @param stockLevel Amount of product available from manufacturer.
* @param stockOrdered Amount of product ordered.
*/
public Manufacturer(String deletedFlag, String name, String location,
String product, String price, String stockLevel, String stockOrdered) {
log.entering("Manufacturer", "Manufacturer", new Object[]{name, location,
product, price, stockLevel, stockOrdered});
this.deletedFlag = deletedFlag;
this.name = name;
this.location = location;
this.product = product;
this.price = price;
this.stockLevel = stockLevel;
this.stockOrdered = stockOrdered;
log.exiting("Manufacturer", "Manufacturer");
}
/* Manufacturer getter and setter methods. */
/**
* Returns the value of the Deleted Flag.
*
* @returns A String
containing the deleted flag value.
*/
public String getDeletedFlag() {
log.entering("Manufacturer", "getDeletedFlag");
log.exiting("Manufacturer", "getDeletedFlag", this.deletedFlag);
return deletedFlag;
}
/**
* Sets the deleted flag value
*
* @param deletedFlag A String
containing the
* deleted flag value.
*/
public void setDeletedFlag(String deletedFlag) {
log.entering("Manufacturer", "setDeletedFlag");
this.deletedFlag = deletedFlag;
log.exiting("Manufacturer", "setDeletedFlag", this.deletedFlag);
}
/**
* Returns the value of the manufacturer name.
*
* @return A String
containing the name of the manufacturer.
*/
public String getName() {
log.entering("Manufacturer", "getName");
log.exiting("Manufacturer", "getName", this.name);
return name;
}
/**
* Sets the manufacturer name value
*
* @param name A String
containing the name of the manufacturer.
*/
public void setName(String name) {
log.entering("Manufacturer", "setName");
this.name = name;
log.exiting("Manufacturer", "setName", this.name);
}
/**
* Returns the value of the manufacturer location.
*
* @return A String
containing the manufacturer location.
*/
public String getLocation() {
log.entering("Manufacturer", "getLocation");
log.exiting("Manufacturer", "getLocation", this.location);
return location;
}
/**
* Sets the manufacturer location value.
*
* @param location A String
containing the manufacturer location.
*/
public void setLocation(String location) {
log.entering("Manufacturer", "setLocation");
this.location = location;
log.exiting("Manufacturer", "setLocation", this.location);
}
/**
* Returns the name of the product.
*
* @return product A String
of name of the product.
*/
public String getProduct() {
log.entering("Manufacturer", "getProduct");
log.exiting("Manufacturer", "getProduct", this.product);
return product;
}
/**
* Sets the types of work performed value.
*
* @param product A single String
of name of the product.
*/
public void setProduct(String product) {
log.entering("Manufacturer", "setProduct");
this.product = product;
log.exiting("Manufacturer", "setProduct", this.product);
}
/**
* Returns the unit price of a product.
*
* @return price A String
containing price of a product.
*/
public String getPrice() {
log.entering("Manufacturer", "getPrice");
log.exiting("Manufacturer", "getPrice", this.price);
return price;
}
/**
* Sets the unit price of a product.
*
* @param price A String
containing price of a product.
*/
public void setPrice(String price) {
log.entering("Manufacturer", "setPrice");
this.price = price;
log.exiting("Manufacturer", "setPrice", this.price);
}
/**
* Returns the stock level of a product.
*
* @return stockLevel A String
containing product stock level.
*/
public String getStockLevel() {
log.entering("Manufacturer", "getStockLevel");
log.exiting("Manufacturer", "getStockLevel", this.stockLevel);
return stockLevel;
}
/**
* Sets the stock level of a product.
*
* @param stockLevel A String
containing stock level.
*/
public void setStockLevel(String stockLevel) {
log.entering("Manufacturer", "setStockLevel");
this.stockLevel = stockLevel;
log.exiting("Manufacturer", "setStockLevel", this.stockLevel);
}
/**
* Returns the amount of stock on order.
*
* @return stockOrdered A String
containing stock on order.
*/
public String getStockOrdered() {
log.entering("Manufacturer", "getStockOrdered");
log.exiting("Manufacturer", "getStockOrdered", this.stockOrdered);
return stockOrdered;
}
/**
* Sets the amount of stock on order.
*
* @param stockOrdered A String
containing stock on order.
*/
public void setStockOrdered(String stockOrdered) {
log.entering("Manufacturer", "setStockOrdered");
this.stockOrdered = stockOrdered;
log.exiting("Manufacturer", "setStockOrdered", this.stockOrdered);
}
/* override Object methods equals(), hashCode() and toString(). */
/**
* Checks whether two Manufacturer objects are the same by
* comparing their name and manufacturer fields.
*
* @param obj The Manufacturer to compare with this Manufacturer
* @return true If this Manufacturer and other manufacturer have
* same name and location.
*/
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof Manufacturer))
return false;
Manufacturer other = (Manufacturer) obj;
if (location == null) {
if (other.location != null)
return false;
} else if (!location.equals(other.location))
return false;
if (name == null) {
if (other.name != null)
return false;
} else if (!name.equals(other.name))
return false;
return true;
}
/**
* Returns a hashCode for this Manufacturer object using name and location
* fields. Although this may not return unique hashCodes it should ensure
* the objects are spread fairly evenly across the buckets.
*
* @return hashKey An int
hashCode for this Manufacturer instance.
*/
@Override
public int hashCode() {
log.entering("Manufacturer", "hashCode");
String hashKey = name + location;
log.exiting("Manufacturer", "hashCode", hashKey.hashCode());
return hashKey.hashCode();
}
/**
* A String
representation of the Manufacturer class.
*
* @return A String
representation of the Manufacturer class.
*/
@Override
public String toString() {
log.entering("Manufacturer", "toString");
String strVal = "["
+ this.deletedFlag + "; "
+ this.name + "; "
+ this.location + "; "
+ this.product + "; "
+ this.price + "; "
+ this.stockLevel + "; "
+ this.stockOrdered
+ "]";
log.exiting("Manufacturer", "toString", strVal);
return strVal;
}
}
Compiling Our Source File With the -cp
and -d
Options
Open your command line editor:
Change to directory cd c:\_Case_Study\src\model
Compile Manufacturer.java
using the java compiler with the -cp
and -d
options
javac -cp ..\..\classes -d ..\..\classes Manufacturer.java
Compiling The ManufacturerFileAccessException
Class Top
Reading files is risky and so we wrap file access calls in try.. catch
blocks to catch any I/O exceptions that may occur. For the case study whenever we access the Manufacturer file we have to code for
a possible exception. So we will need to make a new exception to cater for I/O exceptions when trying to access the Manufacturer file, which we will call ManufacturerFileAccessException
.
Cut and paste the following code into your text editor and save it in the c:\_Case_Study\src\model directory.
package model;
/**
* Holds any data access exceptions that may occur in the Manufacturer file.
*
* @author Charlie
* @version 1.0
* @see StockImpl
*
*/
public class ManufacturerFileAccessException extends RuntimeException {
/**
* A version number for the ManufacturerFileAccessException class so that serialisation
* can occur without worrying about the underlying class changing
* between serialisation and deserialisation.
*
*/
private static final long serialVersionUID = 2498052502L;
/**
* Create a default ManufacturerFileAccessException
instance.
*
*/
public ManufacturerFileAccessException() {
super();
}
/**
* Create a ManufacturerFileAccessException
instance that accepts a String
.
*
* @param e The string description of the exception.
*/
public ManufacturerFileAccessException(String e) {
super(e);
}
/**
* Create a ManufacturerFileAccessException
instance and chain an exception.
*
* @param e The exception to wrap
*/
public ManufacturerFileAccessException(Throwable e) {
super(e);
}
}
Compiling Our Source File With the -cp
and -d
Options
Open your command line editor:
Change to directory cd c:\_Case_Study\src\model
Compile ManufacturerFileAccessException.java
using the java compiler with the -cp
and -d
options
javac -cp ..\..\classes -d ..\..\classes ManufacturerFileAccessException.java
The following screenshot shows that we now get a clean compile and also the Manufacturer
and ManufacturerFileAccessException
classes are now compiled into the classes\model
directory.
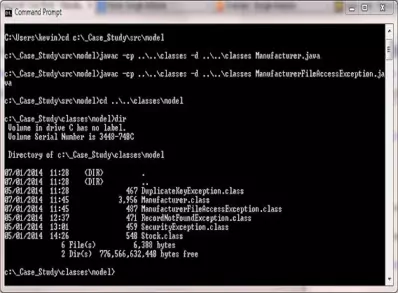
Lesson 5 Complete
In this lesson we coded Manufacturer
and ManufacturerFileAccessException
classes.
Related Java Tutorials
Beginning Java - Primitive Variables
Beginning Java - Conditional Statements
Beginning Java - Loop Statements
Objects & Classes - Class Structure and Syntax
Objects & Classes - Reference Variables
Objects & Classes - Methods
Objects & Classes - Instance Variables & Scope
Objects & Classes - Constructors
Objects & Classes - Static Members
OO Concepts - Encapsulation - Getters & Setters
OO Concepts - Inheritance Concepts - Using the super
keyword
OO Concepts - Interfaces
OO Concepts - The Object
Superclass - Checking Object Equality
OO Concepts - The Object
Superclass - The hashCode()
Method
OO Concepts - The Object
Superclass - The toString()
Method
Exceptions - Handling Exceptions
Exceptions - Declaring Exceptions
Exceptions - Creating Our Own Exceptions
API Contents - Inheritance - Using the package
keyword
API Contents - Inheritance - Using the import
keyword
Concurrency - Synchronization - Synchronized Blocks
Swing - RMI - Serialization
What's Next?
In our final lesson of the Model Part 1 we write an implementation class for the Stock
Interface.